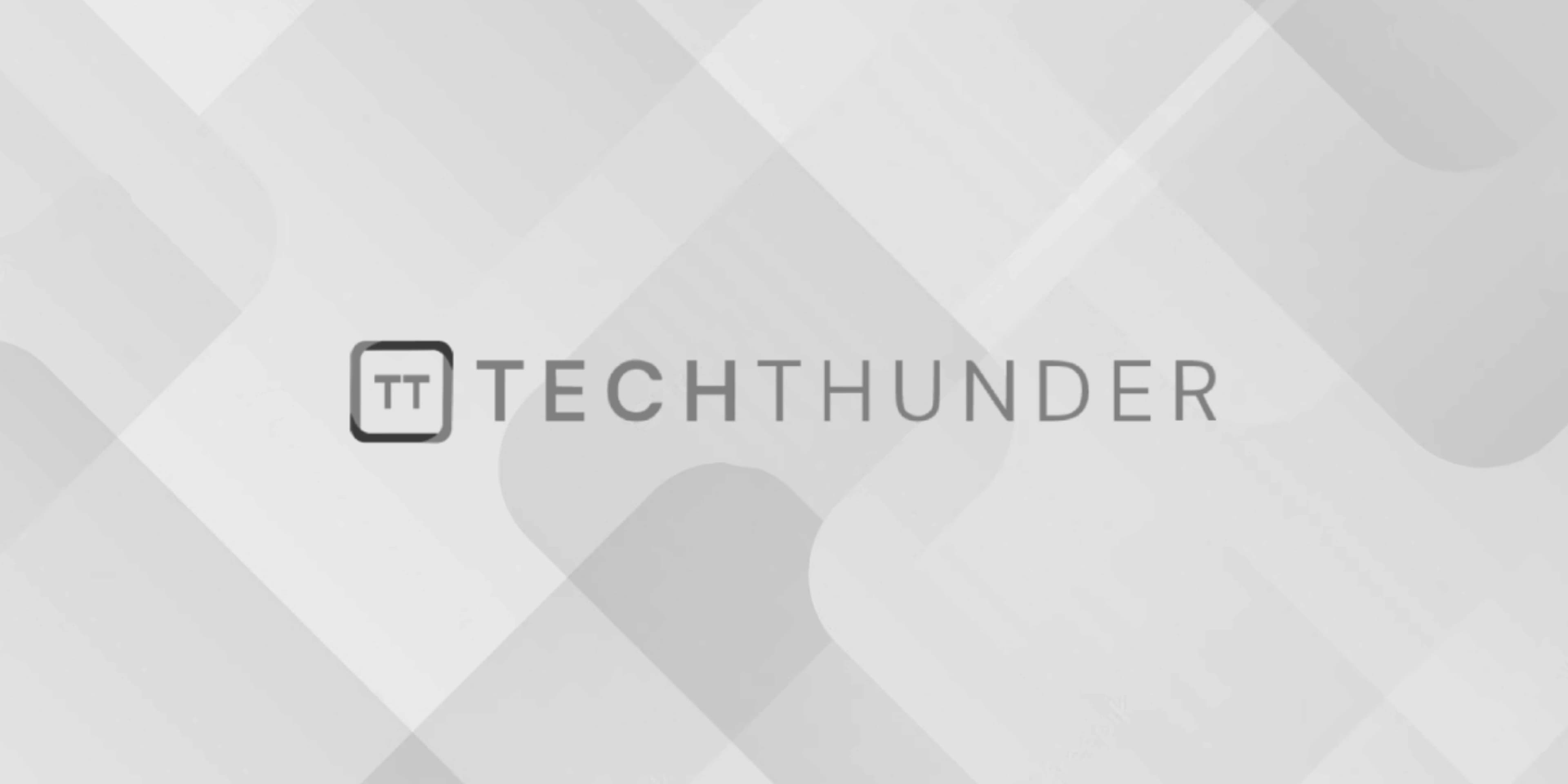
238 views
Set variable without using Arithmetic, Relational or Conditional Operator in C++
You can set a variable without using arithmetic, relational, or conditional operators in C++ by utilizing a technique called “bit manipulation.” Specifically, you can use bitwise operators to manipulate the bits of variables to set or clear specific bits.
Here’s an example of how you can set a specific bit in an integer variable without using arithmetic, relational, or conditional operators:
C++
#include <iostream>
int main() {
int number = 0; // Initialize the variable to 0
int bit_position = 3; // Set the bit at position 3 (0-based index)
// Use bitwise OR to set the bit at the specified position
number |= (1 << bit_position);
std::cout << "Number after setting bit " << bit_position << ": " << number << std::endl;
return 0;
}
In this example:
number
is the integer variable that we want to modify.bit_position
specifies the position of the bit we want to set (0-based index).(1 << bit_position)
is a bitwise left shift operation that shifts a1
bit to the left bybit_position
positions, effectively setting the bit at the desired position.number |= (1 << bit_position)
uses the bitwise OR (|
) operator to set the bit at the specified position innumber
.
After running this code, number
will have the bit at the specified position set, and you can verify this by printing its value.
You can use similar bit manipulation techniques to clear specific bits, toggle bits, or perform other bitwise operations on variables without relying on arithmetic, relational, or conditional operators.