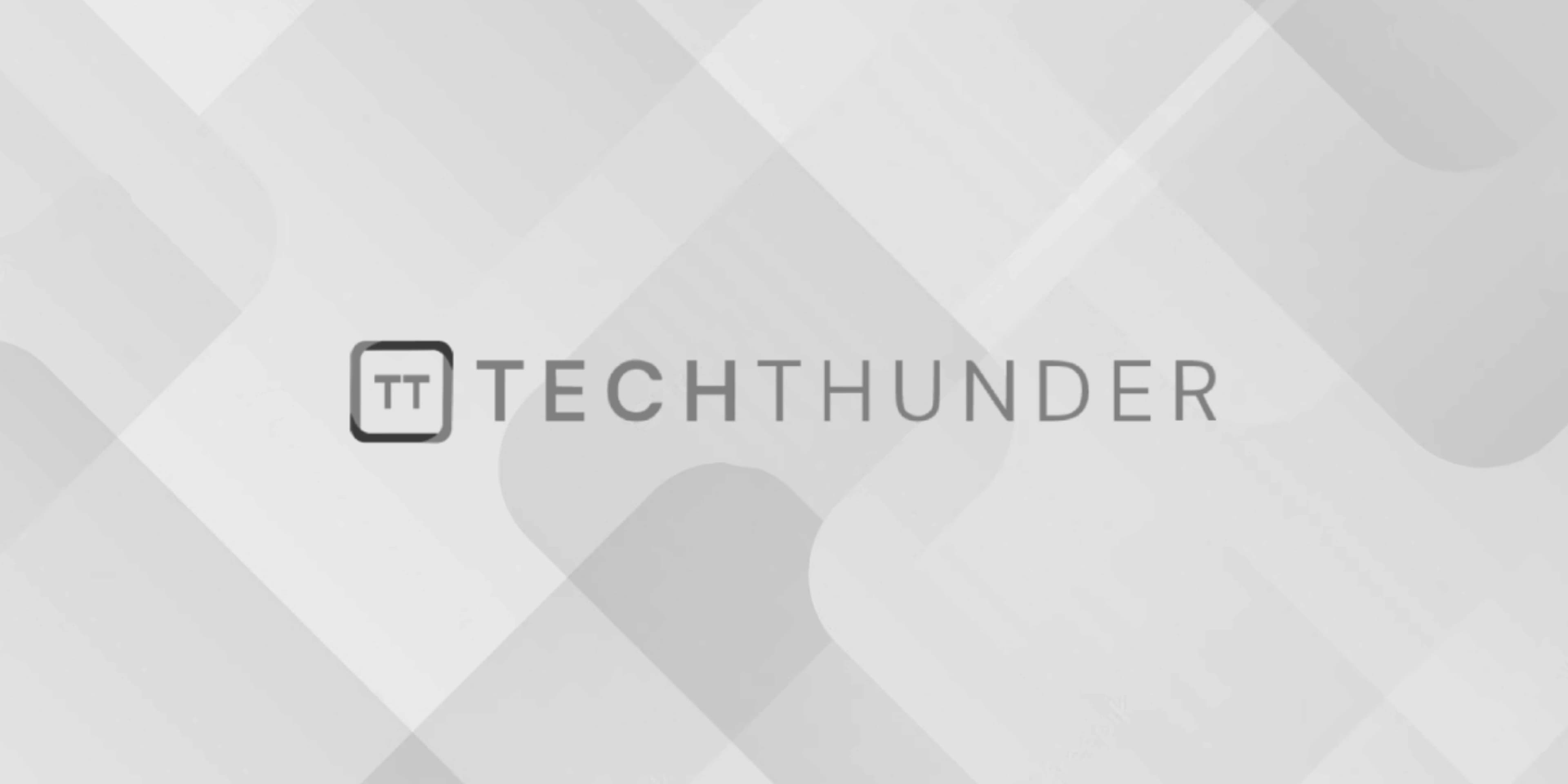
140 views
C++ Class Member Functions
The C++ member functions are functions that are associated with a class or an object of that class. They allow you to define the behavior or operations that can be performed on instances of the class. Member functions are an essential part of object-oriented programming (OOP) and are used to encapsulate data and functionality within a class. Here’s how you declare and define member functions in C++:
C++
class MyClass {
public:
// Member function declaration
void memberFunction1();
// Member function declaration with parameters
void memberFunction2(int arg1, double arg2);
// Member function with a return value
int memberFunction3();
// Constructor (special member function)
MyClass();
// Destructor (special member function)
~MyClass();
private:
// Member data (private by default)
int data1;
double data2;
};
// Member function definitions outside the class
void MyClass::memberFunction1() {
// Function body
// You can access member data here using 'data1' and 'data2'
}
void MyClass::memberFunction2(int arg1, double arg2) {
// Function body with parameters
}
int MyClass::memberFunction3() {
// Function body with a return value
return 42;
}
// Constructor definition
MyClass::MyClass() {
// Constructor body
// Initialize member data or perform setup operations
}
// Destructor definition
MyClass::~MyClass() {
// Destructor body
// Clean up resources, if needed
}
Here’s an explanation of the key elements:
- Member Function Declaration:
- Member functions are declared inside the class definition. They specify the name, parameters (if any), and return type (if any) of the function.
- Member functions can be public, private, or protected, depending on their intended accessibility.
- Member Function Definition:
- The actual implementation of member functions is provided outside the class using the scope resolution operator
::
. This is where you define what the member function does.
- Member Data:
- Member functions can access and manipulate member data (class variables) declared within the class.
- Constructor and Destructor:
- Constructors are special member functions used for initializing objects when they are created. They have the same name as the class and no return type.
- Destructors are special member functions used for cleanup operations when objects go out of scope or are explicitly deleted.
- Function Parameters:
- Member functions can take parameters just like regular functions. You can define their behavior based on these parameters.
To use a class and its member functions, you typically create objects of the class and then call the member functions on those objects:
C++
MyClass obj;
obj.memberFunction1();
int result = obj.memberFunction3();
This allows you to encapsulate data and behavior within classes, promoting modularity and encapsulation in your C++ code.