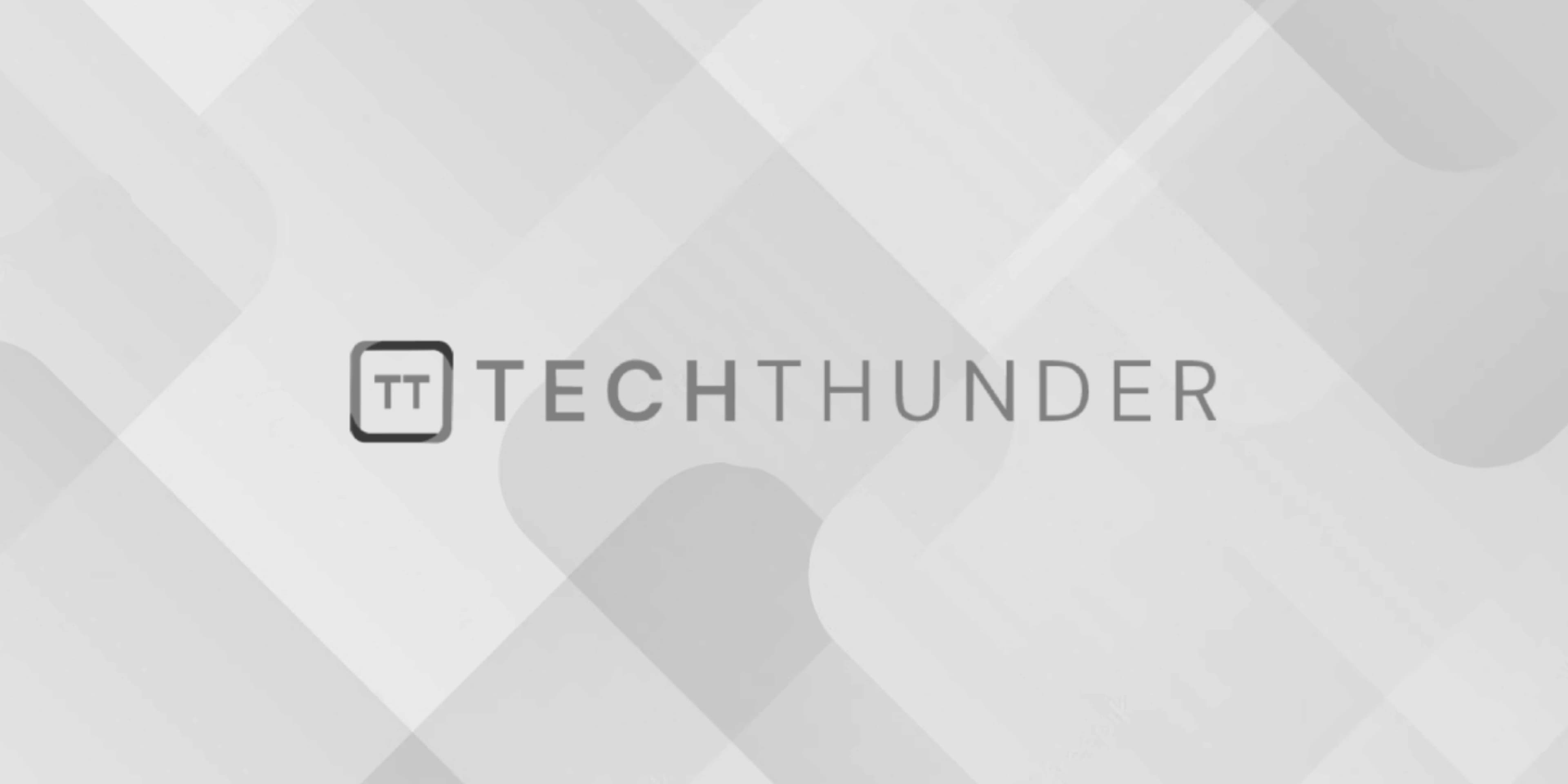
141 views
Reverse Number in C++
The reverse a number in C++, you can extract the digits from the original number one by one and build the reversed number accordingly. Here’s a simple program to reverse a number:
C++
#include <iostream>
int main() {
int number, reversedNumber = 0;
// Input an integer
std::cout << "Enter an integer: ";
std::cin >> number;
while (number != 0) {
int digit = number % 10; // Get the last digit
reversedNumber = reversedNumber * 10 + digit; // Add the digit to the reversed number
number /= 10; // Remove the last digit
}
std::cout << "Reversed number: " << reversedNumber << std::endl;
return 0;
}
In this program:
- The user is prompted to enter an integer.
- A
while
loop is used to reverse the number digit by digit. In each iteration, the last digit of the original number is extracted using the modulo operator (% 10
) and added to the reversed number after shifting the reversed number one position to the left by multiplying it by 10. The last digit is then removed from the original number using integer division (/ 10
). - The result is the reversed number, which is printed to the console.
Compile and run this program, and it will reverse the input integer and display the reversed number.