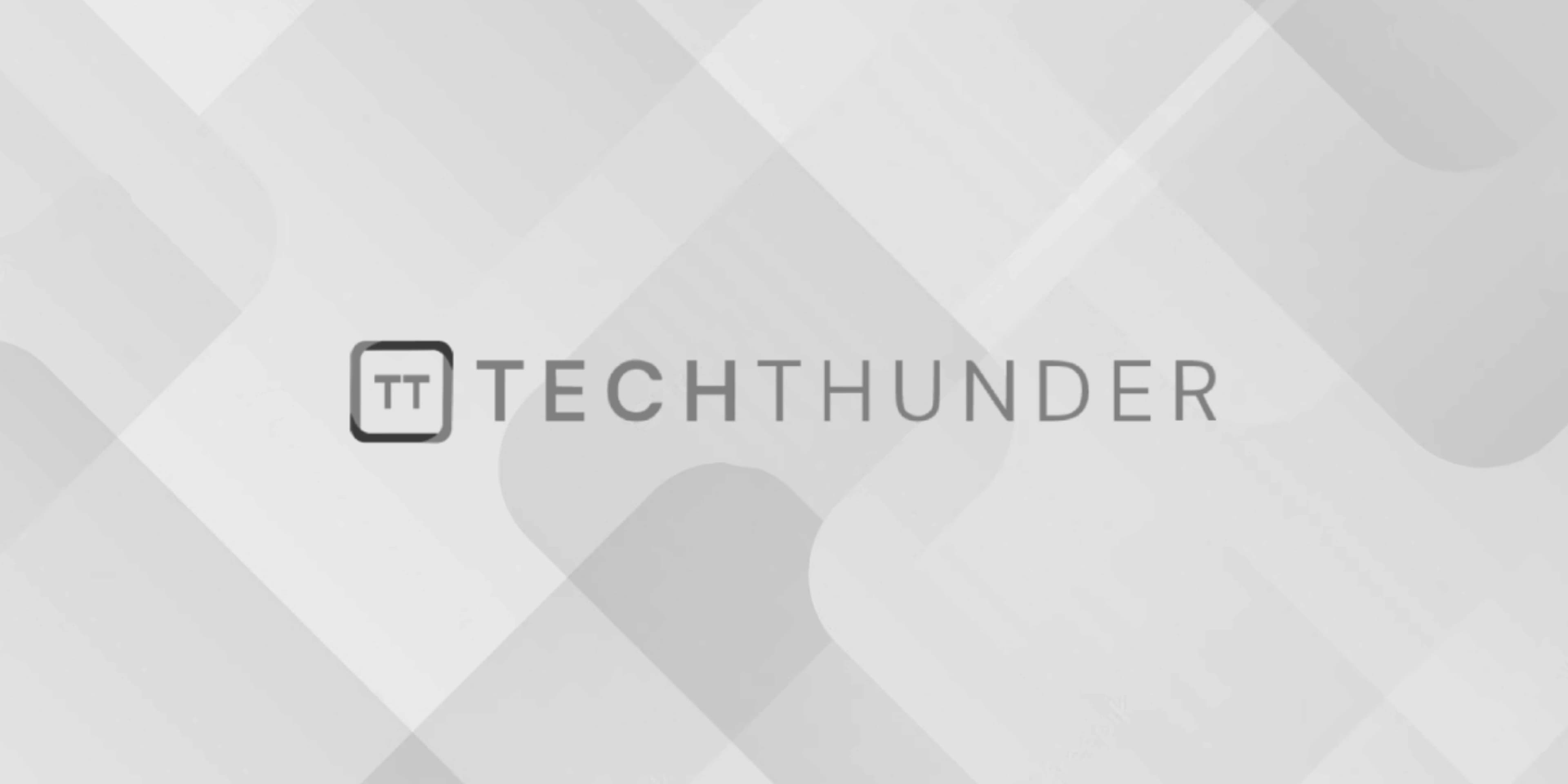
285 views
Alphabet Triangle in C++
To create an alphabet triangle in C++, you can use nested loops to print a pattern of alphabets. Here’s an example of how to create an alphabet triangle:
C++
#include <iostream>
int main() {
int n;
char currentChar = 'A';
// Input the number of rows for the alphabet triangle
std::cout << "Enter the number of rows: ";
std::cin >> n;
for (int i = 1; i <= n; ++i) {
// Print spaces for formatting
for (int j = 1; j <= n - i; ++j) {
std::cout << " ";
}
// Print alphabets in ascending order
for (int k = 1; k <= i; ++k) {
std::cout << currentChar << " ";
++currentChar; // Move to the next alphabet
}
// Move to the next line
std::cout << std::endl;
}
return 0;
}
In this program:
- The user is prompted to enter the number of rows for the alphabet triangle.
- A variable
currentChar
is used to keep track of the current alphabet, initially set to ‘A’. - Nested loops are used to iterate through the rows and columns of the triangle.
- In the inner loop, the current alphabet (
currentChar
) is printed, andcurrentChar
is incremented to move to the next alphabet. - The result is a pattern of alphabets forming an alphabet triangle.
For example, if you enter 5
as the number of rows, the program will generate the following alphabet triangle:
Plaintext
A
B C
D E F
G H I J
K L M N O
You can adjust the number of rows by changing the value of n
to create an alphabet triangle of the desired size.