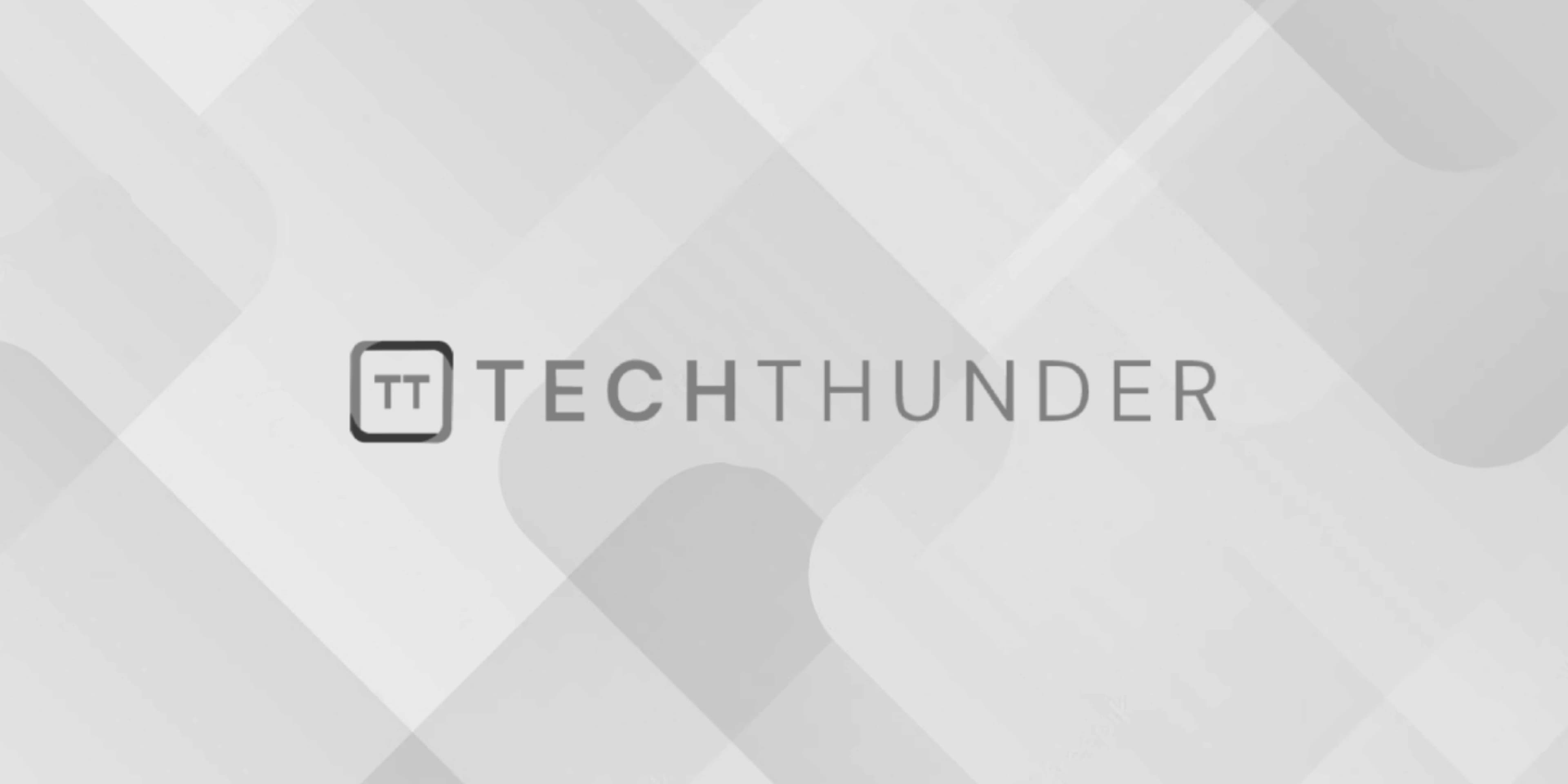
Multimap find() in C++ STL
The C++ STL can use the std::multimap::find()
function to search for a specific key in a std::multimap
container. A std::multimap
is an associative container that allows duplicate keys, and it stores key-value pairs in a sorted order based on the keys. The find()
function returns an iterator to the first occurrence of the specified key in the multimap or end()
if the key is not found.
Here’s the syntax for using std::multimap::find()
:
iterator find(const key_type& key);
key
: The key you want to search for in the multimap.iterator
: An iterator pointing to the first occurrence of the specified key. If the key is not found, it returnsend()
.
Here’s an example of how to use std::multimap::find()
:
#include <iostream>
#include <map>
int main() {
std::multimap<int, std::string> myMultimap;
// Insert key-value pairs into the multimap
myMultimap.insert({1, "Alice"});
myMultimap.insert({2, "Bob"});
myMultimap.insert({2, "Charlie"});
myMultimap.insert({3, "David"});
// Find the first occurrence of key 2
std::multimap<int, std::string>::iterator it = myMultimap.find(2);
if (it != myMultimap.end()) {
std::cout << "Key 2 found: " << it->second << std::endl;
} else {
std::cout << "Key 2 not found." << std::endl;
}
// Find the first occurrence of key 4 (not present)
it = myMultimap.find(4);
if (it != myMultimap.end()) {
std::cout << "Key 4 found: " << it->second << std::endl;
} else {
std::cout << "Key 4 not found." << std::endl;
}
return 0;
}
In this example:
- We create a
std::multimap
calledmyMultimap
and insert several key-value pairs into it. - We use
myMultimap.find(2)
to search for the first occurrence of the key2
in the multimap. Since key2
has multiple values associated with it, thefind()
function returns an iterator pointing to the first occurrence of2
(which corresponds to “Bob”). - We also attempt to find key
4
, which is not present in the multimap. In this case, thefind()
function returnsend()
because the key is not found. - We check the iterator returned by
find()
to determine whether the key was found or not.
When using std::multimap::find()
, keep in mind that it returns an iterator to the first occurrence of the key. If there are multiple values associated with the same key, you may need to use other techniques to access all the values associated with that key.