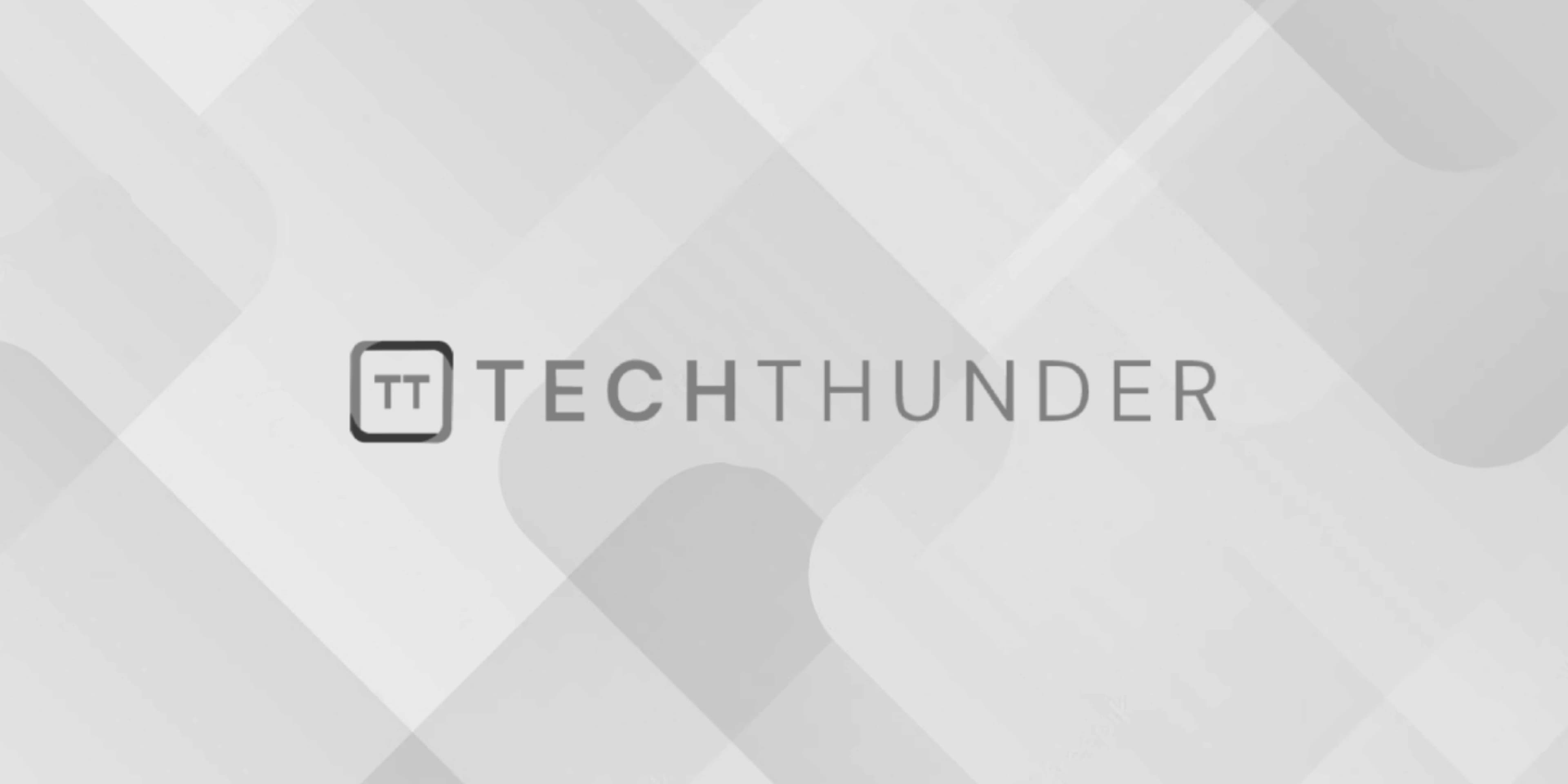
C++ date and time
You can work with date and time information using the <chrono>
and <ctime>
libraries for measuring time intervals and formatting time values, respectively. Additionally, the C++ Standard Library provides the <iomanip>
library for formatting output. Here’s a basic overview of how to work with date and time in C++:
1. Using <chrono>
for Time Intervals:
The <chrono>
library provides a way to work with time durations and time points. Here’s how you can use it:
#include <iostream>
#include <chrono>
int main() {
// Create a time duration of 5 seconds
std::chrono::seconds duration(5);
// Get the number of seconds in the duration
int seconds = duration.count();
std::cout << "Duration in seconds: " << seconds << std::endl;
// Get the current time point
std::chrono::system_clock::time_point now = std::chrono::system_clock::now();
// Perform some operations with time points
std::chrono::system_clock::time_point later = now + duration;
return 0;
}
2. Using <ctime>
for Formatting Time:
The <ctime>
library provides functions for formatting and parsing time values. Here’s an example:
#include <iostream>
#include <ctime>
int main() {
// Get the current time
std::time_t currentTime = std::time(nullptr);
// Convert time to a string representation
std::tm* timeInfo = std::localtime(¤tTime);
char timeString[100];
std::strftime(timeString, sizeof(timeString), "%Y-%m-%d %H:%M:%S", timeInfo);
std::cout << "Current time: " << timeString << std::endl;
return 0;
}
3. Using <iomanip>
for Formatting Output:
You can use the <iomanip>
library to format the output of date and time values:
#include <iostream>
#include <iomanip>
#include <ctime>
int main() {
std::time_t currentTime = std::time(nullptr);
std::tm* timeInfo = std::localtime(¤tTime);
// Format and print the time
std::cout << "Current time: ";
std::cout << std::put_time(timeInfo, "%Y-%m-%d %H:%M:%S") << std::endl;
return 0;
}
These are basic examples, and the C++ Standard Library provides more comprehensive facilities for working with date and time, including std::chrono
for precise timing, std::tm
for date and time components, and formatting and parsing functions like std::strftime
and std::put_time
. Depending on your specific needs, you can choose the appropriate library and functions to work with date and time in C++.