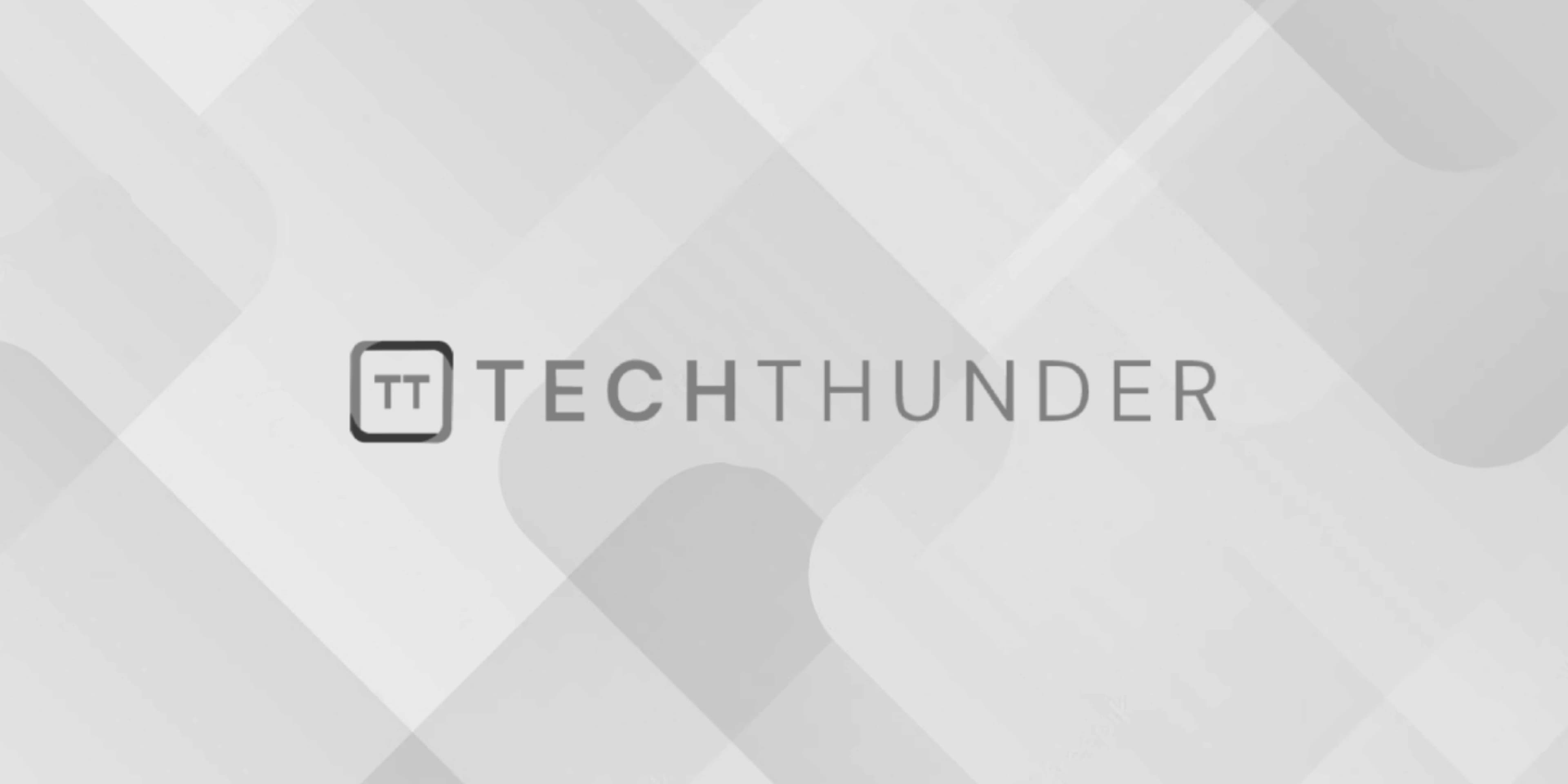
136 views
C++ Data types
The C++ data types define the type of data a variable can hold. Each data type has a specific set of values it can represent and a range of operations that can be performed on it. Here are some of the basic data types in C++:
- Integer Types:
int
: Represents integers, typically with a size of 4 bytes on most systems.short
: Represents smaller integers, with a size of at least 2 bytes.long
: Represents larger integers, with a size of at least 4 bytes.long long
: Represents very large integers, introduced in C++11.
- Floating-Point Types:
float
: Represents single-precision floating-point numbers.double
: Represents double-precision floating-point numbers.long double
: Represents extended-precision floating-point numbers.
- Character Types:
char
: Represents a single character, typically 1 byte in size.wchar_t
: Represents a wide character, used for representing characters from extended character sets.
- Boolean Type:
bool
: Represents a boolean value, which can be eithertrue
orfalse
.
- Void Type:
void
: Represents an absence of a type. It is used as the return type for functions that do not return a value.
- Derived Data Types:
arrays
: A collection of elements of the same data type, indexed by a continuous range of values.pointers
: Variables that store memory addresses. They point to the location of other variables.references
: Provide an alias to a variable.
- User-Defined Data Types:
struct
: A compound data type that groups together variables under a single name.class
: Similar to astruct
, but can include member functions and has additional features like inheritance and polymorphism.enum
: Defines an enumeration type, which allows you to create named constants.
- Type Modifiers:
signed
andunsigned
: Modify integer types to indicate whether they can represent both positive and negative numbers, or only non-negative numbers.short
andlong
: Modify integer types to represent numbers of different sizes.
- Type Aliases (introduced in C++11):
using
: Allows you to create aliases for existing data types.
C++
using myType = int; // 'myType' is now an alias for 'int'
- Auto Type Deduction (introduced in C++11):
auto
: Allows the compiler to automatically deduce the data type based on the initialization expression.
C++
auto x = 10; // 'x' is deduced as an int
Choosing the right data type is important for efficiently using memory and ensuring that your program can handle the range of values you expect. It’s also essential for preventing overflows or loss of precision in calculations. Understanding