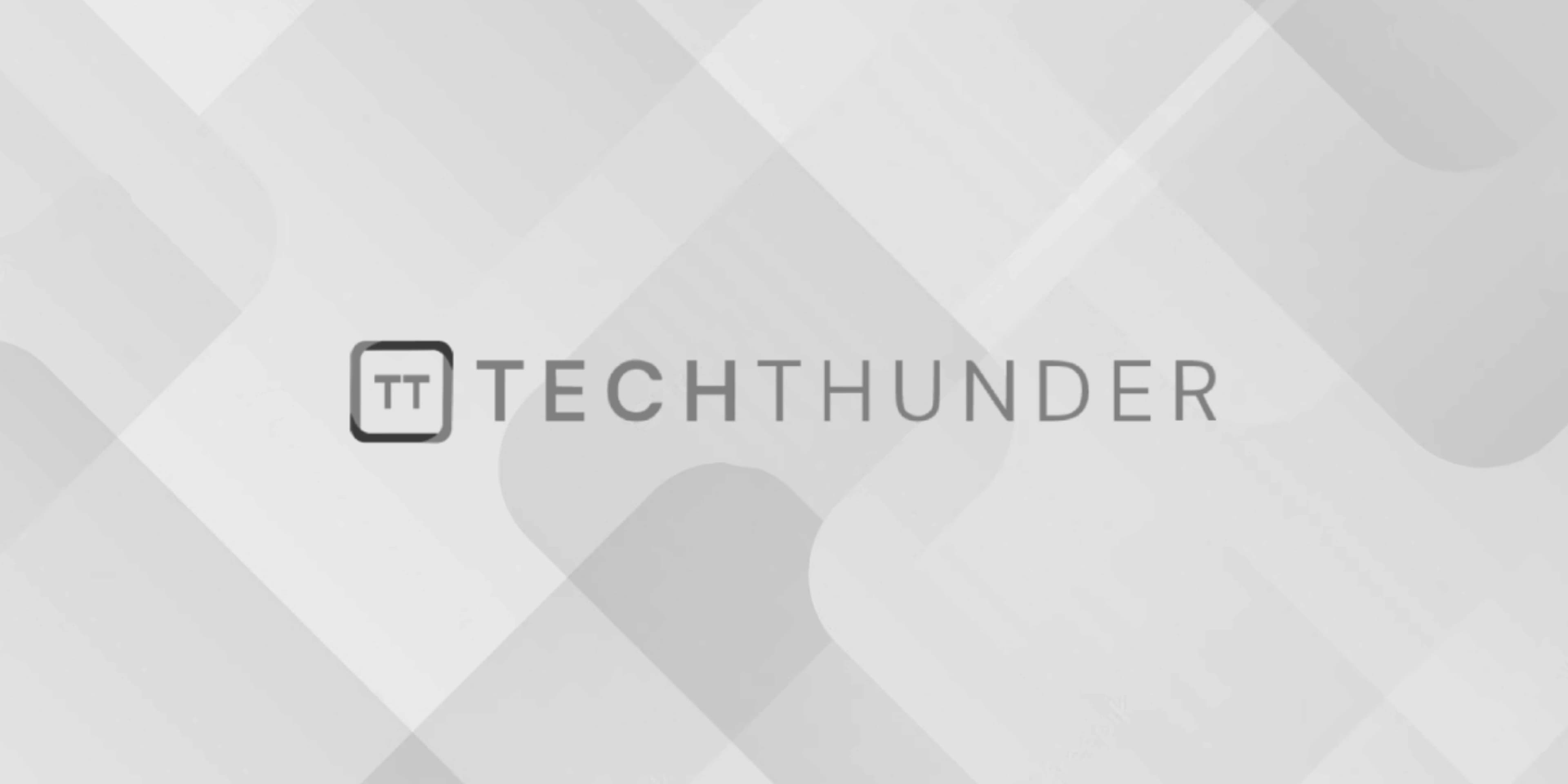
Add in Vector C++ Language
The C++ add elements to a vector using the push_back()
method or by directly assigning values to specific indices. Here are two common ways to add elements to a vector:
- Using
push_back()
method: Thepush_back()
method appends an element to the end of the vector. It is commonly used to add elements dynamically to a vector.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
// Add elements to the vector using push_back()
numbers.push_back(10);
numbers.push_back(20);
numbers.push_back(30);
// Display the vector
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
In this example, we create an empty vector numbers
and use push_back()
to add integers to it.
- Direct Assignment or Indexing: You can also add elements to a vector by directly assigning values to specific indices. However, you need to ensure that you’re not accessing an index that is out of bounds, as vectors do not automatically resize in this case.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers(3); // Create a vector with 3 elements
// Add elements using direct assignment
numbers[0] = 10;
numbers[1] = 20;
numbers[2] = 30;
// Display the vector
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
In this example, we create a vector numbers
with a size of 3 elements and then assign values to those elements using indexing. Note that if you exceed the allocated size (e.g., numbers[3] = 40;
), it will result in undefined behavior, so it’s essential to manage the vector’s size appropriately.
The push_back()
method is the safer and more dynamic way to add elements to a vector because it automatically resizes the vector as needed. However, if you know the size you need in advance, you can also use the constructor to initialize the vector with a specific size, as shown in the second example.