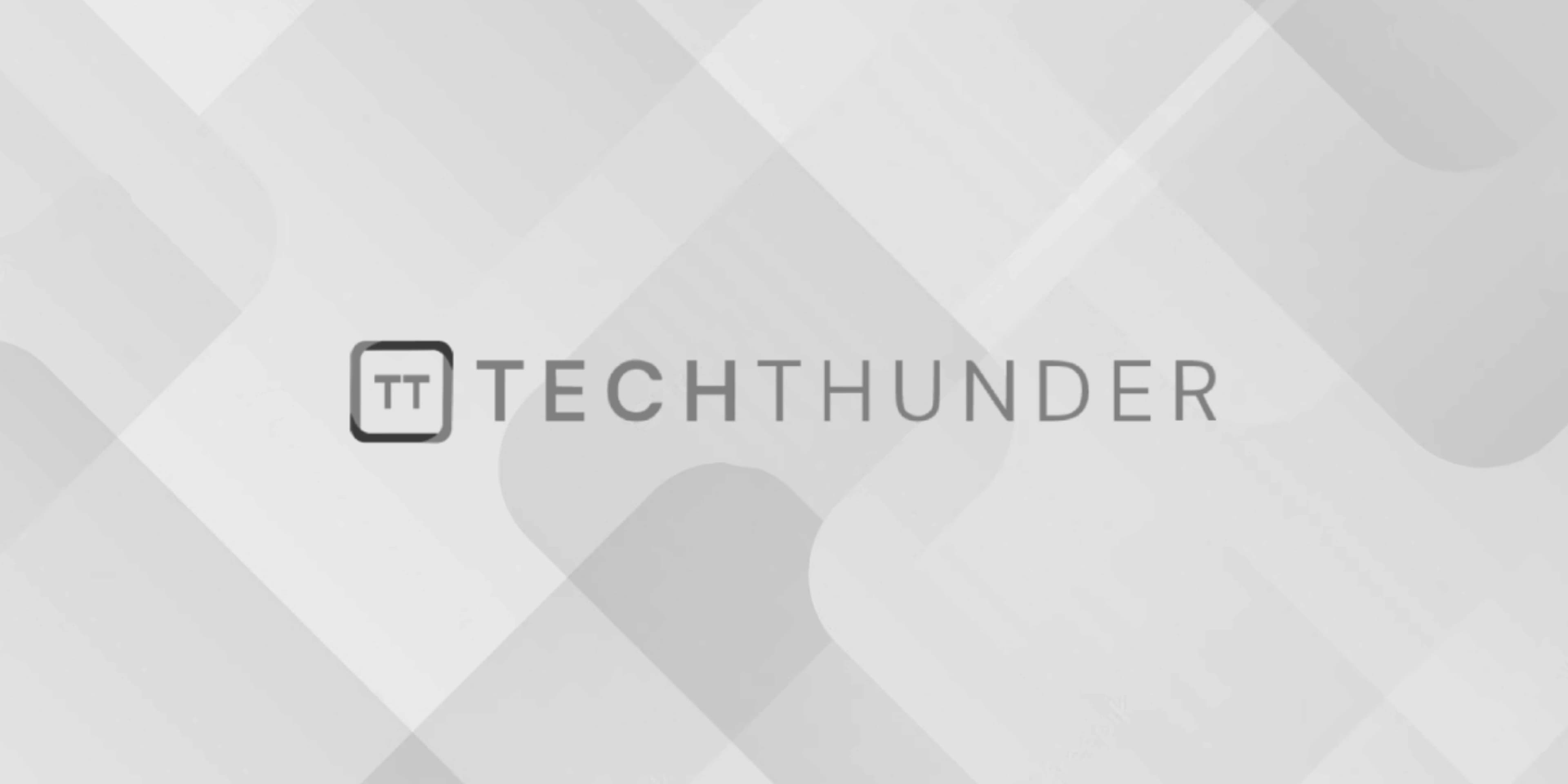
Dynamic memory allocation in C++
Dynamic memory allocation in C++ allows you to allocate memory at runtime, which is especially useful when you don’t know the memory requirements of your program in advance or when you need to create data structures of varying sizes. C++ provides two primary ways to perform dynamic memory allocation:
new
anddelete
Operators: Thenew
operator is used to allocate memory on the heap, and thedelete
operator is used to deallocate memory that was previously allocated withnew
. Here’s an example of dynamic memory allocation usingnew
:
int* dynamicArray = new int[5]; // Allocating an array of 5 integers
dynamicArray[0] = 1;
dynamicArray[1] = 2;
// ...
// Deallocate the memory when done
delete[] dynamicArray;
It’s important to use delete[]
when deallocating arrays allocated with new[]
and delete
when deallocating single objects allocated with new
.
malloc()
andfree()
Functions: C++ also allows you to use the C standard library functionsmalloc()
andfree()
for dynamic memory allocation:
int* dynamicArray = (int*)malloc(5 * sizeof(int)); // Allocating an array of 5 integers
dynamicArray[0] = 1;
dynamicArray[1] = 2;
// ...
// Deallocate the memory using free
free(dynamicArray);
When using malloc()
, you need to cast the result to the appropriate pointer type. Also, don’t forget to use free()
to deallocate memory allocated with malloc()
.
It’s important to note that when using dynamic memory allocation, you are responsible for managing memory explicitly, including deallocation. Failing to deallocate memory can lead to memory leaks, which can cause your program to consume more and more memory over time.
In modern C++, it’s often recommended to use smart pointers and containers like std::vector
or std::unique_ptr
whenever possible. These classes provide automatic memory management and help avoid many common memory-related errors.
Here’s an example of using std::vector
for dynamic storage:
#include <vector>
int main() {
std::vector<int> dynamicArray;
dynamicArray.push_back(1);
dynamicArray.push_back(2);
// ...
// No need to manually deallocate memory; it's handled by the vector
return 0;
}
Using std::vector
or smart pointers, such as std::unique_ptr
or std::shared_ptr
, is generally considered safer and more convenient than managing raw pointers and dynamic memory allocation manually.