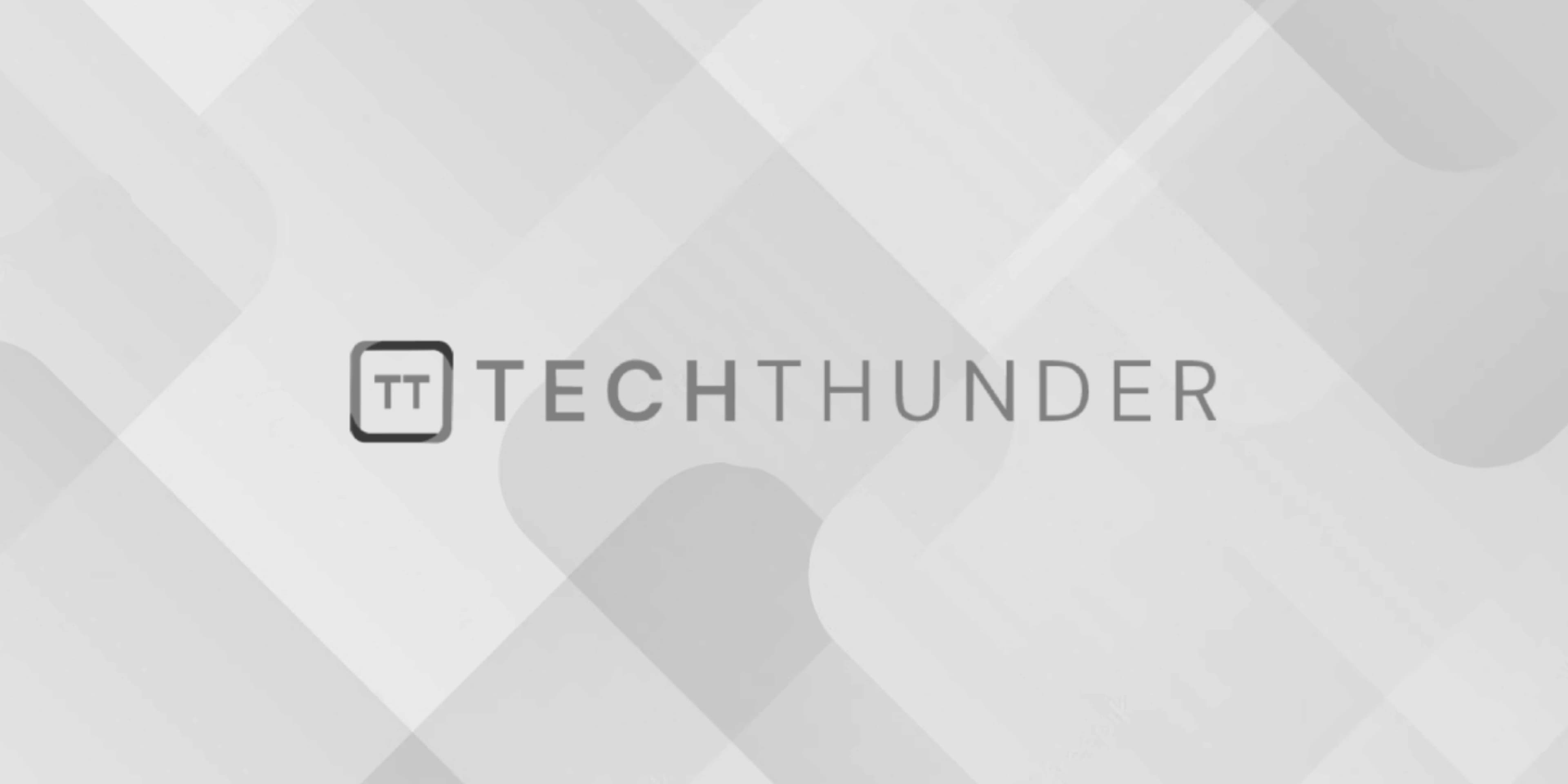
C++ Bitset
The C++ std::bitset
is a standard library class provided by the <bitset>
header that represents a fixed-size sequence of bits. It is a container that stores a sequence of binary values (0s and 1s) and provides various operations for manipulating and inspecting these bits. Here’s an overview of how to use std::bitset
:
Declaration and Initialization:
You can declare and initialize a std::bitset
with a specified size during declaration or later. For example:
#include <bitset>
#include <iostream>
int main() {
// Declare and initialize a bitset of size 8 with all bits set to 0.
std::bitset<8> bits1;
// Initialize a bitset with a binary string.
std::bitset<8> bits2("10101010");
// Initialize a bitset with an integer value.
std::bitset<8> bits3(170);
std::cout << "bits1: " << bits1 << std::endl;
std::cout << "bits2: " << bits2 << std::endl;
std::cout << "bits3: " << bits3 << std::endl;
return 0;
}
Bit Manipulation:
std::bitset
provides various methods for manipulating individual bits or sets of bits, including:
set()
: Sets a specific bit to 1.reset()
: Resets (sets to 0) a specific bit.flip()
: Toggles (flips) the value of a specific bit.test()
: Tests the value of a specific bit.
std::bitset<8> bits(42);
bits.set(2); // Set the 3rd bit to 1
bits.reset(5); // Reset the 6th bit to 0
bits.flip(0); // Toggle the 1st bit
bool bitValue = bits.test(3); // Test the 4th bit
Bitwise Operations:
You can perform bitwise operations on std::bitset
objects, including AND (&
), OR (|
), XOR (^
), and NOT (~
).
std::bitset<8> bits1("11001100");
std::bitset<8> bits2("10101010");
std::bitset<8> resultAND = bits1 & bits2;
std::bitset<8> resultOR = bits1 | bits2;
std::bitset<8> resultXOR = bits1 ^ bits2;
std::bitset<8> resultNOT = ~bits1;
Conversion:
You can convert a std::bitset
to an integer using the to_ulong()
or to_ullong()
functions. These functions return the decimal equivalent of the binary representation stored in the bitset.
std::bitset<8> bits("10101010");
unsigned long decimalValue = bits.to_ulong();
Size and Count:
You can get the size (number of bits) of a std::bitset
using the size()
function, and you can count the number of set bits (1s) using the count()
function.
std::bitset<8> bits("10101010");
size_t size = bits.size(); // Returns 8
size_t countSetBits = bits.count(); // Returns 4
std::bitset
is useful when you need to work with a fixed-size sequence of bits and perform bit-level operations efficiently.