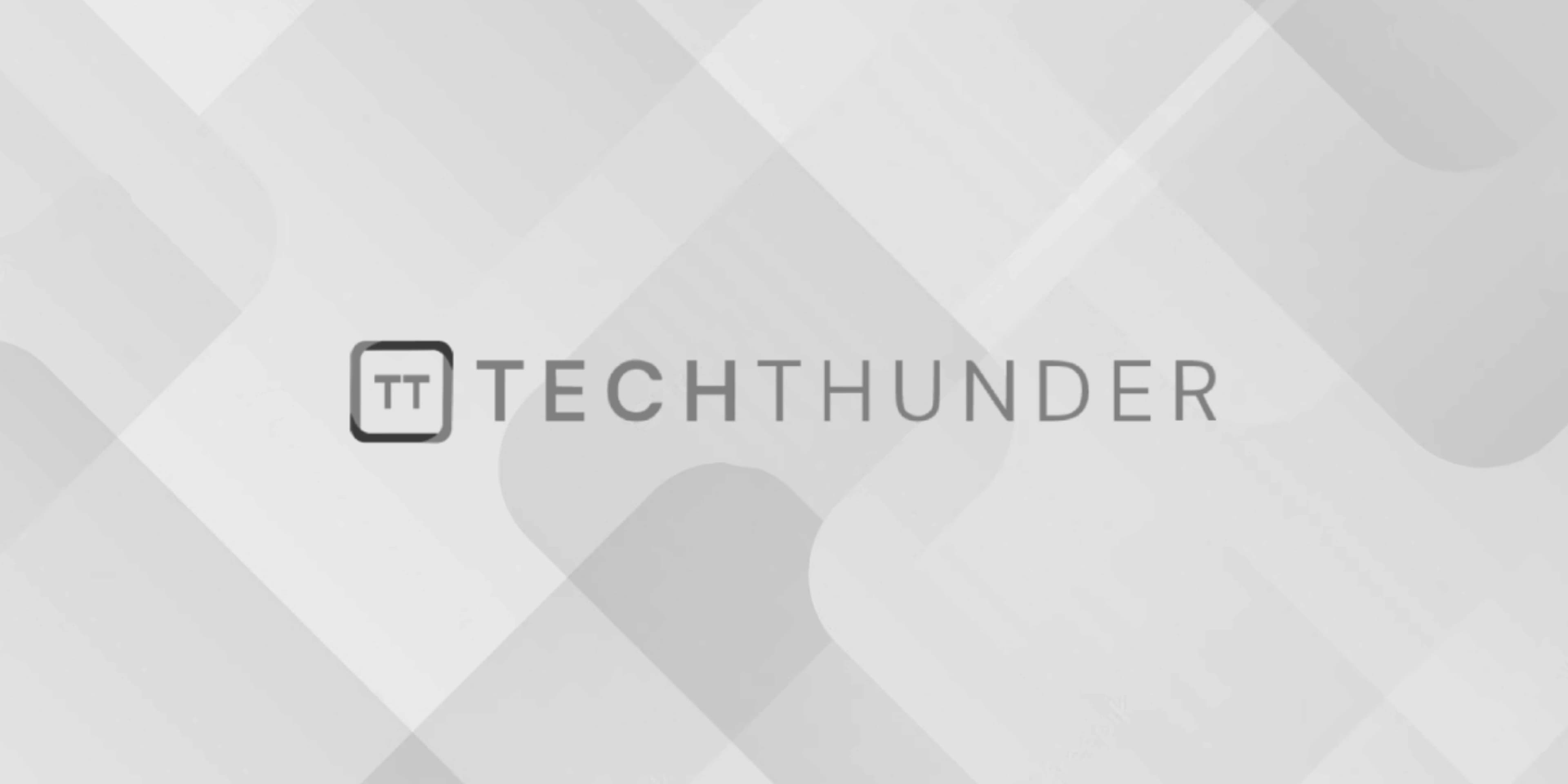
Parameterised Constructor in C++
The C++ parameterized constructor is a constructor that takes one or more parameters when an object of a class is created. Parameterized constructors allow you to initialize the object’s data members with specific values during object creation. They provide a way to set the initial state of an object based on the arguments provided to the constructor.
Here’s the basic syntax for defining a parameterized constructor in C++:
class MyClass {
public:
// Parameterized constructor
MyClass(int param1, double param2) {
// Initialize data members with the provided parameters
memberVar1 = param1;
memberVar2 = param2;
}
// Other member functions and data members
private:
int memberVar1;
double memberVar2;
};
int main() {
// Create an object of MyClass and provide arguments to the constructor
MyClass obj(42, 3.14);
// The constructor initializes memberVar1 to 42 and memberVar2 to 3.14
return 0;
}
In this example:
- The
MyClass
class has a parameterized constructor that takes two parameters, an integer (param1
) and a double (param2
). - Inside the constructor, the data members
memberVar1
andmemberVar2
are initialized with the values provided as arguments. - In the
main()
function, we create an object ofMyClass
namedobj
and pass42
and3.14
as arguments to the constructor. This results in the initialization ofmemberVar1
with42
andmemberVar2
with3.14
.
Parameterized constructors are useful when you want to ensure that objects of a class are created in a well-defined state, and you want to provide initial values for the object’s data members based on the requirements of your program. They allow you to customize the construction process of objects and are commonly used in C++ classes to provide flexibility and ensure correct object initialization.