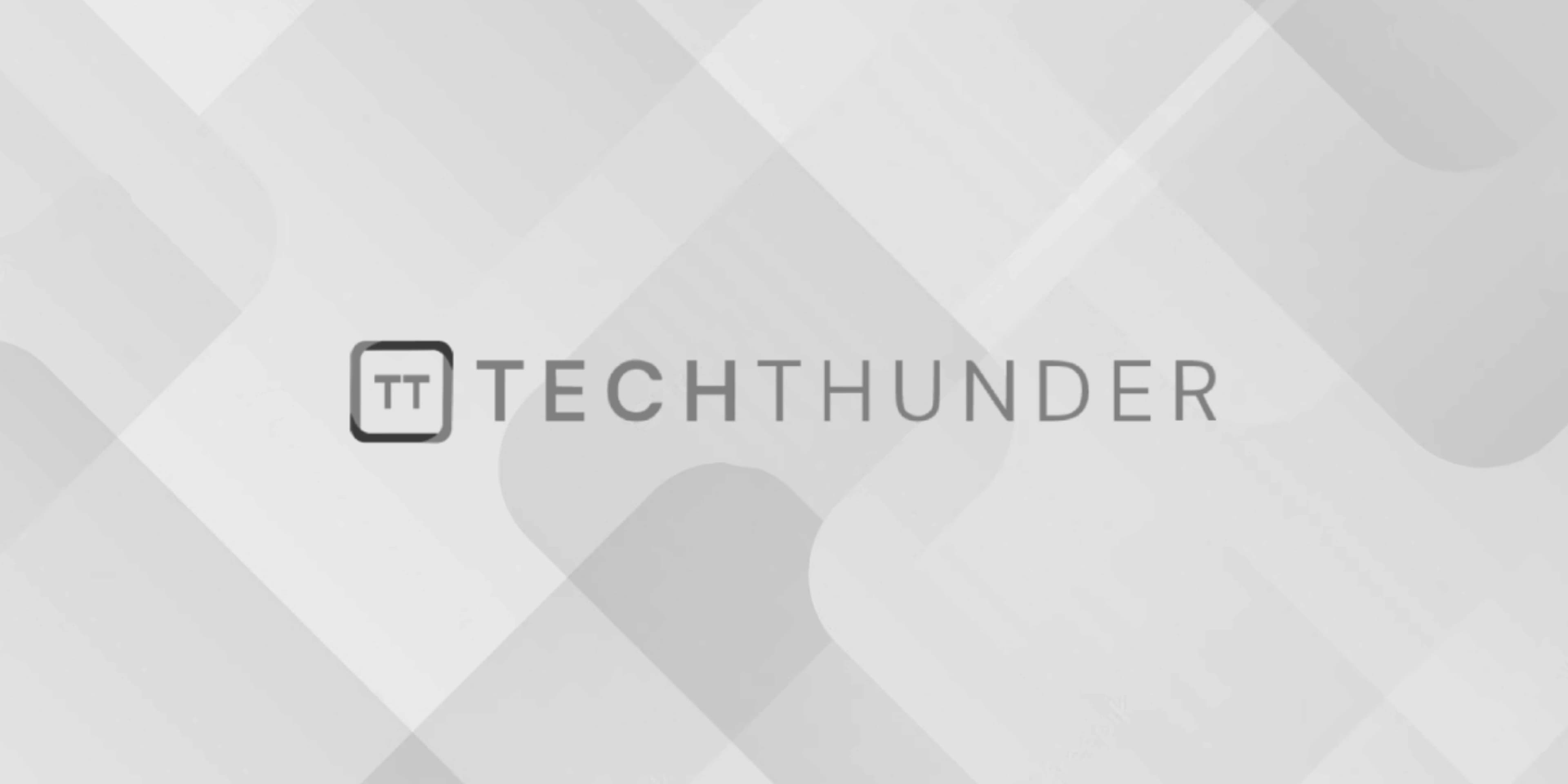
233 views
Initialise an Array of objects with Parameterised Constructors in C++
The C++ can initialize an array of objects with parameterized constructors by explicitly providing the constructor arguments for each object in the array during initialization. Here’s an example:
Let’s assume you have a class called Student
with a parameterized constructor that takes a name and an age:
C++
#include <iostream>
#include <string>
class Student {
public:
// Parameterized constructor
Student(const std::string& name, int age) : name(name), age(age) {}
// Member function to display student details
void display() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
private:
std::string name;
int age;
};
int main() {
// Initialize an array of Student objects with parameterized constructors
Student students[] = {
Student("Alice", 20),
Student("Bob", 22),
Student("Charlie", 21)
};
// Access and display the objects in the array
for (const Student& student : students) {
student.display();
}
return 0;
}
In this example:
- The
Student
class has a parameterized constructor that takes aname
and anage
. It also has adisplay
member function to display student details. - In the
main
function, we initialize an array ofStudent
objects with parameterized constructors by providing the constructor arguments for each object in the curly braces{}
. - We then loop through the array of
Student
objects and call thedisplay
member function to display the details of each student.
This way, you can create an array of objects and pass the required arguments to their parameterized constructors during initialization.