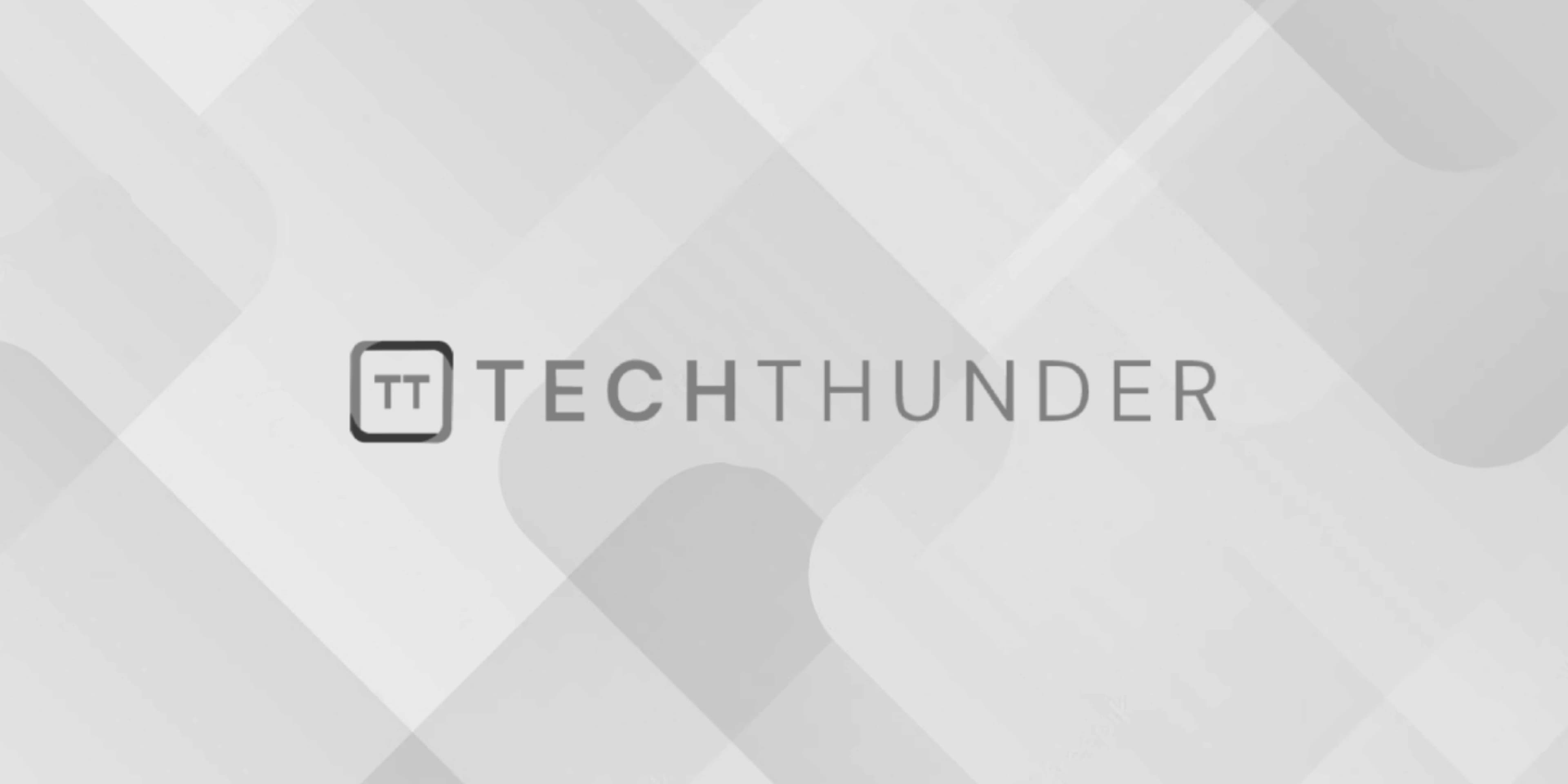
multimap::emplace_hint() Function in C++ STL
The std::multimap::emplace_hint()
function in C++ is a member function of the std::multimap
container from the C++ Standard Library. It allows you to efficiently insert an element into a multimap
while providing a hint about the position where the element should be inserted. Using a hint can help improve the insertion performance, especially when you have some knowledge about the expected location of the element.
Here’s the syntax of the emplace_hint()
function:
iterator emplace_hint(const_iterator position, Args... args);
position
: A hint indicating the position where the element should be inserted. The function will attempt to insert the element at or near this position.Args... args
: Arguments that are forwarded to the constructor of the element being inserted.
The emplace_hint()
function returns an iterator pointing to the newly inserted element or to the element that prevented the insertion if it failed.
Here’s an example of how to use emplace_hint()
with a multimap
:
#include <iostream>
#include <map>
int main() {
std::multimap<int, std::string> studentMap;
// Using emplace_hint to insert elements efficiently
std::multimap<int, std::string>::iterator hint = studentMap.begin();
hint = studentMap.emplace_hint(hint, 101, "Alice"); // Insert at or near the beginning
hint = studentMap.emplace_hint(hint, 105, "Bob"); // Insert after Alice
// Insert more elements
studentMap.emplace_hint(hint, 103, "Charlie");
studentMap.emplace_hint(hint, 104, "David");
// Print the multimap
for (const auto& pair : studentMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
In this example:
- We create a
std::multimap
namedstudentMap
that associates student IDs (integers) with their names (strings). - We use
emplace_hint()
to efficiently insert elements at or near the specified position in themultimap
. The hint iterator is initially set tobegin()
, and we insert elements with student IDs and names. - The output will show the elements in the
multimap
in the order they were inserted. Usingemplace_hint()
can help optimize insertion when you have some knowledge about the desired position.
Note that the use of hints is optional, and the C++ Standard Library implementation will still ensure correct insertion even without hints. Hints can be beneficial in scenarios where you want to optimize the insertion performance.