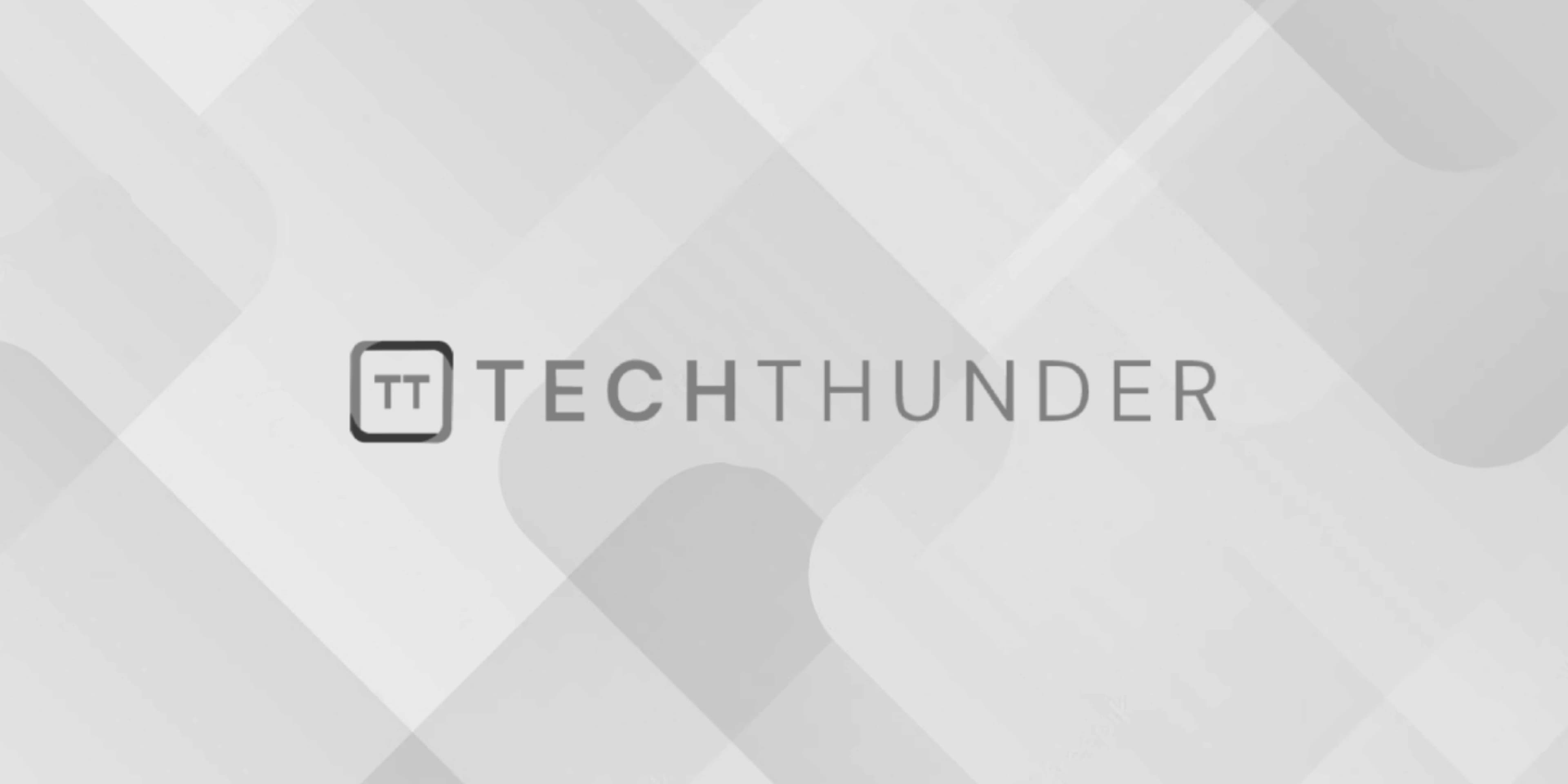
What is operator overloading in C++
Operator overloading in C++ is a feature that allows you to redefine the behavior of existing operators or create custom operators for user-defined types (classes or structures). It enables you to use operators with user-defined types in a way that is meaningful and natural for your application. This feature is a fundamental part of C++’s support for object-oriented programming and operator polymorphism.
Here’s an overview of operator overloading in C++:
- Operator Overloading Syntax: To overload an operator, you need to define a special member function for the operator in your class. The operator function is defined using the
operator
keyword followed by the operator you want to overload. For example, to overload the+
operator, you would define a member function like this:
returnType operator+(const SomeClass& other) const {
// Define the behavior of the + operator for your class
}
Here, returnType
is the type of the result when the +
operator is applied to objects of your class.
- Examples of Operator Overloading: Here are some common operators that can be overloaded in C++:
- Arithmetic operators like
+
,-
,*
,/
,%
. - Comparison operators like
==
,!=
,<
,<=
,>
,>=
. - Unary operators like
+
,-
,++
,--
,!
. - Assignment operators like
=
,+=
,-=
,*=
,/=
, etc. You can also overload custom operators for your classes, such as a matrix multiplication operator or a custom addition operator.
- Rules and Guidelines: When overloading operators, there are some important rules and guidelines to follow:
- You cannot create new operators in C++. You can only overload existing operators.
- You cannot change the precedence and associativity of operators through overloading. The overloaded operator will have the same precedence and associativity as the built-in operator.
- Some operators, like
()
,[]
, and->
, have special overloading rules and syntax. - Overloaded operators can be member functions of a class or global functions. Member function overloads have access to the private members of the class.
- Example of Operator Overloading: Here’s a simple example of overloading the
+
operator for a user-definedComplex
class:
class Complex {
private:
double real;
double imag;
public:
Complex(double r, double i) : real(r), imag(i) {}
// Overload the + operator for Complex objects
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// Getter functions for real and imag
double getReal() const { return real; }
double getImag() const { return imag; }
};
int main() {
Complex a(2.0, 3.0);
Complex b(1.5, 2.5);
Complex result = a + b;
std::cout << "Result: " << result.getReal() << " + " << result.getImag() << "i" << std::endl;
return 0;
}
In this example, the +
operator is overloaded for Complex
objects to perform complex number addition in a natural way.
Operator overloading allows you to write more intuitive and expressive code when working with user-defined types. However, it should be used judiciously, and operator behaviors should be consistent with their expected meanings to avoid confusion in your code.