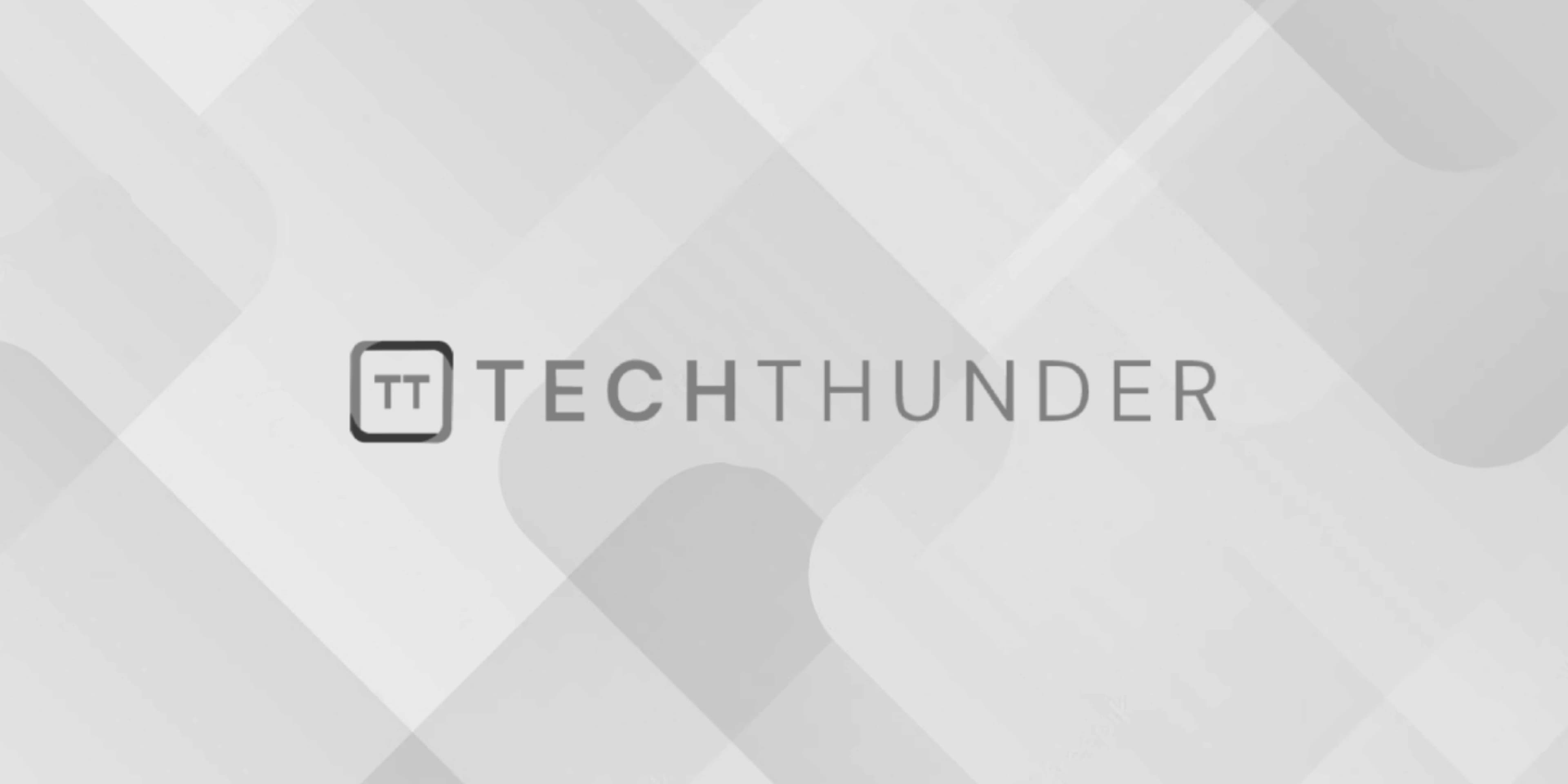
240 views
C++ Multimap
The C++ std::multimap
is a container provided by the C++ Standard Library that allows you to store and manage a collection of key-value pairs. It is similar to std::map
, but unlike std::map
, it allows multiple elements with the same key. In other words, it allows duplicate keys, and elements with the same key are stored in sorted order based on the key.
Here’s how to use std::multimap
in C++:
C++
#include <iostream>
#include <map>
int main() {
// Create a multimap with integer keys and string values
std::multimap<int, std::string> myMultiMap;
// Insert key-value pairs into the multimap
myMultiMap.insert(std::make_pair(1, "Alice"));
myMultiMap.insert(std::make_pair(2, "Bob"));
myMultiMap.insert(std::make_pair(1, "Charlie")); // Duplicate key 1
// Access values using keys
std::cout << "Values for key 1:" << std::endl;
auto range = myMultiMap.equal_range(1);
for (auto it = range.first; it != range.second; ++it) {
std::cout << it->second << std::endl;
}
// Check if a key exists in the multimap
if (myMultiMap.find(3) != myMultiMap.end()) {
std::cout << "Key 3 found in the multimap." << std::endl;
} else {
std::cout << "Key 3 not found in the multimap." << std::endl;
}
// Iterate over the multimap
for (const auto& pair : myMultiMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
return 0;
}
In this example:
- We include the
<map>
header to usestd::multimap
. - We create a multimap with integer keys and string values (
std::multimap<int, std::string>
). - Key-value pairs are inserted into the multimap using the
insert
function. - To access values with a specific key, we use the
equal_range
function to obtain a range of iterators, and then we iterate over that range. - We check if a key exists in the multimap using
find
. - To iterate over the entire multimap, we use a range-based for loop, which provides access to each key-value pair in the multimap.
std::multimap
is a useful data structure when you need to store multiple values associated with the same key while maintaining the sorted order based on keys. It is commonly used in various scenarios where you need to handle duplicate keys in a sorted manner.