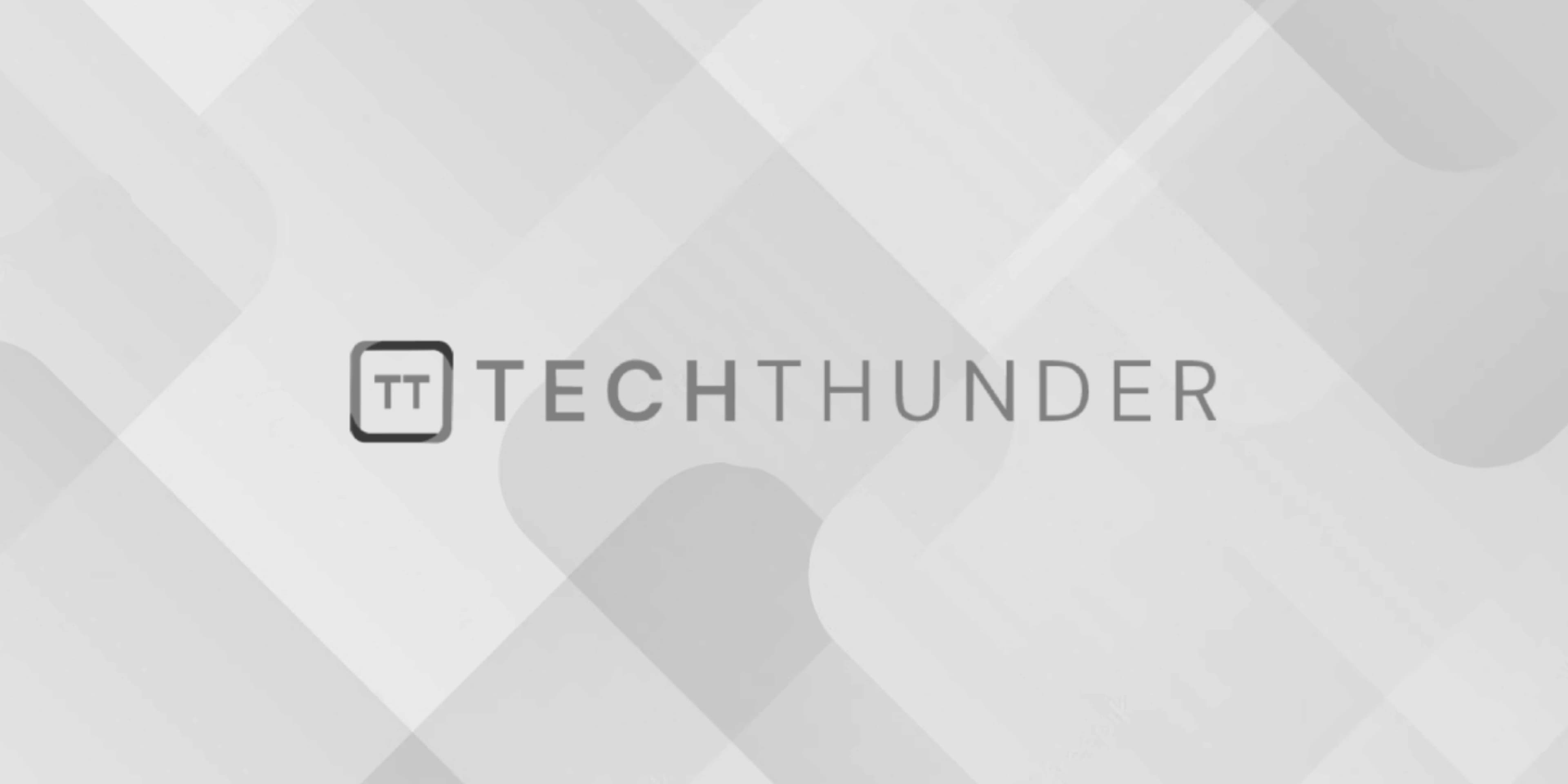
Multithreading in C++
Multithreading in C++ allows you to create and manage multiple threads of execution within a single program. This can be useful for parallelizing tasks to take advantage of modern multi-core processors and improving program performance. C++ provides a standard library for multithreading support, introduced in the C++11 standard, and later versions have added more features and improvements. Here’s an overview of how to work with multithreading in C++:
Basic Multithreading Example
#include <iostream>
#include <thread>
// Function to be executed by a thread
void threadFunction() {
for (int i = 0; i < 5; ++i) {
std::cout << "Thread ID: " << std::this_thread::get_id() << " Count: " << i << std::endl;
}
}
int main() {
// Create a thread and execute the threadFunction
std::thread t1(threadFunction);
// Join the thread to the main thread
t1.join();
std::cout << "Main thread ID: " << std::this_thread::get_id() << std::endl;
return 0;
}
In this example:
- We include the necessary headers (
<iostream>
and<thread>
) for multithreading. - We define a function
threadFunction()
that will be executed by a separate thread. - In
main()
, we create a new threadt1
and passthreadFunction
as the function to be executed in that thread. - We use
join()
to ensure that the main thread waits fort1
to finish before proceeding. This is important to avoid the main thread exiting before the thread has completed its work.
Key Concepts and Functions
Here are some key concepts and functions related to multithreading in C++:
std::thread
: Thestd::thread
class represents a thread of execution. You create a thread by passing a function or callable object to its constructor, as shown in the example above.std::this_thread::get_id()
: This function retrieves the ID of the current thread.std::thread::join()
: Thejoin()
method blocks the calling thread until the thread it is called on finishes execution.std::thread::detach()
: Thedetach()
method allows the thread to run independently, and you won’t need to join it later. Use with caution, as you lose control over the thread’s lifetime.std::thread::hardware_concurrency()
: This function returns the number of concurrent threads that the implementation’s thread library can run.
Data Sharing and Synchronization
When using multithreading, you must be careful about data sharing and synchronization between threads to avoid race conditions and data corruption. C++ provides various synchronization primitives such as std::mutex
, std::condition_variable
, and std::atomic
for this purpose.
Here’s a basic example using a mutex for synchronization:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void threadFunction(int id) {
for (int i = 0; i < 5; ++i) {
std::lock_guard<std::mutex> lock(mtx); // Lock the mutex
std::cout << "Thread " << id << ": " << i << std::endl;
}
}
int main() {
std::thread t1(threadFunction, 1);
std::thread t2(threadFunction, 2);
t1.join();
t2.join();
return 0;
}
In this example, we use a std::mutex
to protect access to the std::cout
stream, ensuring that only one thread can print at a time to avoid interleaved output.
Keep in mind that multithreading can introduce complexities, and proper synchronization is crucial to avoid issues like data races. Advanced synchronization techniques and libraries such as std::condition_variable
, std::atomic
, and std::thread
provide more robust solutions for managing multithreaded code.