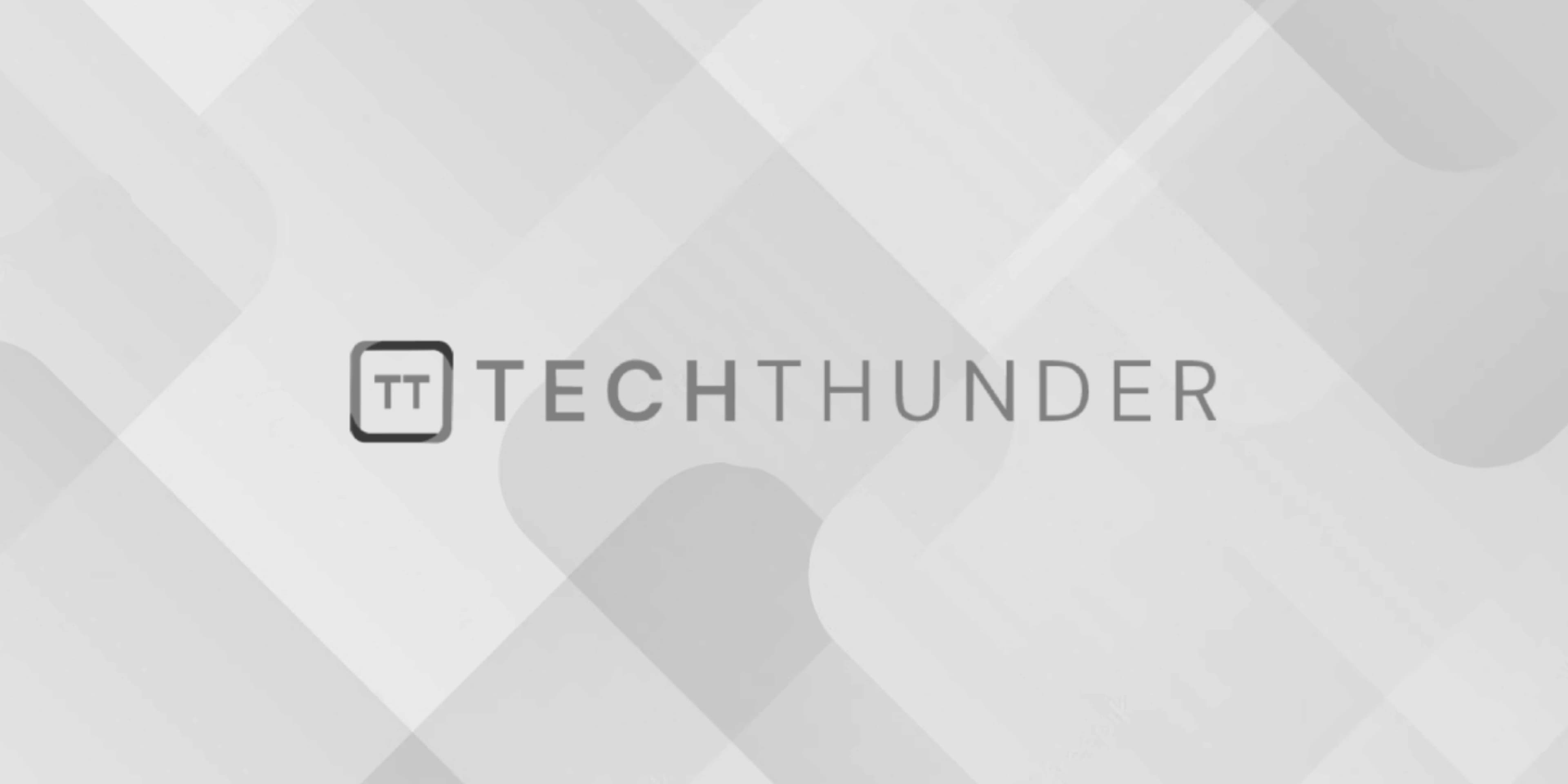
C++ Multidimensional Arrays
The C++ multidimensional arrays are arrays with more than one dimension, essentially creating a matrix or a grid of values. You can declare and work with multidimensional arrays using various syntaxes and techniques. Here are some common ways to define and use multidimensional arrays in C++:
Method 1: Using Nested Arrays
You can create multidimensional arrays by nesting arrays within each other. This method is straightforward and works for arrays of any dimension.
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Accessing elements is done using multiple indices:
int element = matrix[1][2]; // Accesses the value 6 (second row, third column)
Method 2: Using Arrays of Pointers
Another approach is to create an array of pointers, where each pointer points to an array. This method provides more flexibility because each subarray can have a different size.
int* matrix[3];
matrix[0] = new int[3]{1, 2, 3};
matrix[1] = new int[2]{4, 5};
matrix[2] = new int[4]{6, 7, 8, 9};
Accessing elements is still done using multiple indices:
int element = matrix[1][1]; // Accesses the value 5
Don’t forget to deallocate memory when using this method:
for (int i = 0; i < 3; ++i) {
delete[] matrix[i];
}
Method 3: Using std::vector
(Preferred)
In modern C++, it’s recommended to use std::vector
for multidimensional arrays because it provides dynamic sizing, automatic memory management, and better safety.
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int element = matrix[1][2]; // Accesses the value 6
return 0;
}
This method is preferred because std::vector
automatically handles memory allocation and deallocation, and it provides a safer and more flexible way to work with multidimensional arrays.
Method 4: Using std::array
(C++11 and later)
In C++11 and later, you can also use std::array
to create fixed-size multidimensional arrays with a more modern syntax.
#include <iostream>
#include <array>
int main() {
std::array<std::array<int, 3>, 3> matrix = {{
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
}};
int element = matrix[2][1]; // Accesses the value 8
return 0;
}
std::array
provides compile-time size checking and safety similar to C-style arrays but with more modern C++ features.
Choose the method that best suits your needs and the requirements of your program. In modern C++, std::vector
and std::array
are often preferred due to their flexibility, safety, and ease of use.