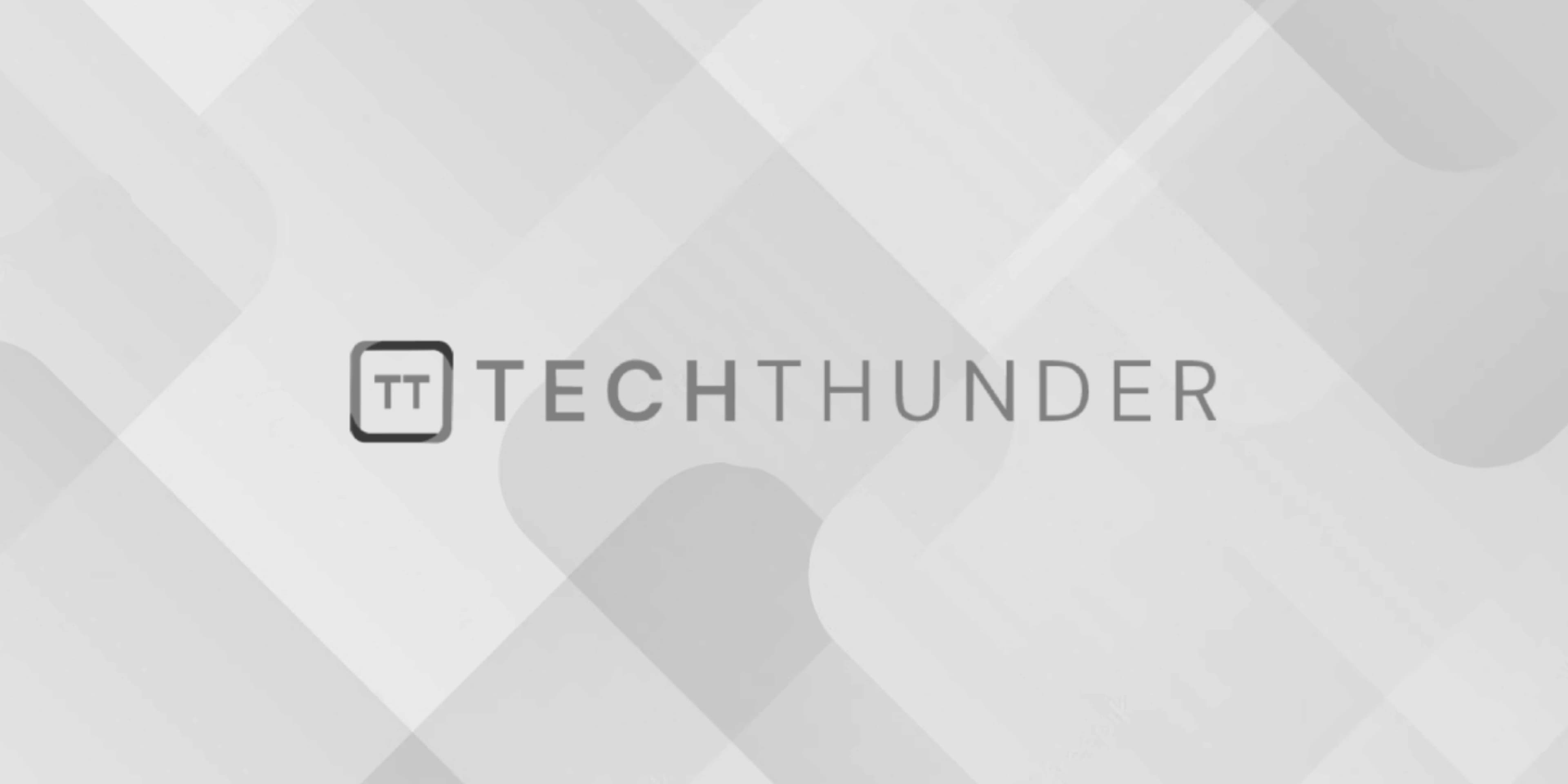
321 views
Characteristics of Destructor in C++
The destructor is a special member function of a class that is automatically called when an object of that class goes out of scope or is explicitly deleted. The purpose of a destructor is to clean up any resources or memory allocated by the object during its lifetime. Here are the key characteristics of destructors in C++:
- Name and Syntax: A destructor has the same name as the class, preceded by a tilde (
~
). It doesn’t take any arguments or return values. The syntax for declaring a destructor is:
~ClassName();
- No Explicit Invocation: Destructors are automatically called when an object’s scope is exited or when the
delete
operator is used to explicitly destroy an object. - No Overloading: Unlike constructors, destructors cannot be overloaded with different parameter lists. Each class can have only one destructor.
- Order of Execution: When multiple objects are destroyed, their destructors are called in the reverse order of their construction. Inheritance also affects the order of destructor calls: the base class destructor is called before the derived class destructor.
- Virtual Destructors: If you have a class hierarchy with virtual functions and you plan to delete objects of derived classes through a base class pointer, it’s recommended to make the base class destructor virtual. This ensures that the correct destructor is called for the derived class objects, preventing memory leaks.
C++
class Base {
public:
virtual ~Base() { }
};
class Derived : public Base {
public:
~Derived() { }
};
- Resource Cleanup: Destructors are often used to release resources such as memory, file handles, network connections, or other external resources acquired by the object. This helps prevent resource leaks.
- Implicitly Generated Destructors: If a class does not define its own destructor, the compiler will provide a default destructor. The default destructor performs no special actions, which might not be sufficient for classes that manage resources.
- Manual Resource Management: Classes that manage dynamically allocated memory (using
new
anddelete
) should perform memory deallocation in their destructor to avoid memory leaks. Similarly, objects that hold other non-memory resources should release them in the destructor. - Exceptions: Destructors should avoid throwing exceptions since there is limited control over their invocation, and throwing exceptions during stack unwinding can lead to program termination.
- Use Cases: Destructors are used to ensure proper cleanup of resources, closing files, releasing memory, and generally performing any necessary cleanup operations when an object is no longer needed.
In summary, destructors are an essential component of C++ classes, responsible for releasing resources and performing cleanup when objects are no longer needed. Properly managing destructors helps maintain a clean and efficient program with minimal resource leaks.