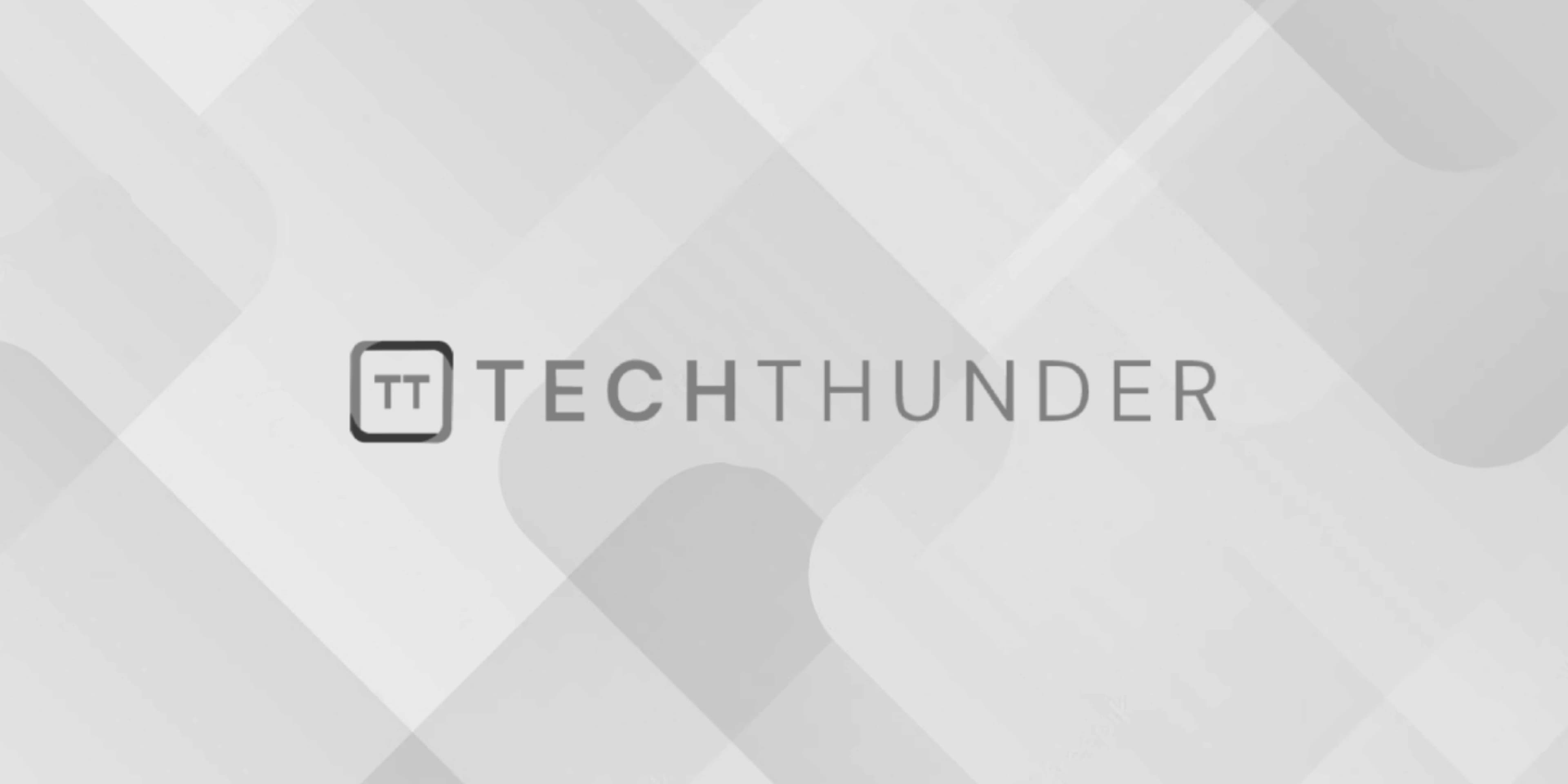
Adding Two Arrays in C++
The add two arrays element-wise by iterating through them and adding corresponding elements together. Here’s an example of how you can do this:
#include <iostream>
int main() {
const int size = 5; // Define the size of the arrays
int array1[size] = {1, 2, 3, 4, 5};
int array2[size] = {6, 7, 8, 9, 10};
int sum[size]; // Resultant array to store the sum
// Add the elements of array1 and array2 element-wise
for (int i = 0; i < size; ++i) {
sum[i] = array1[i] + array2[i];
}
// Display the result
std::cout << "Sum of arrays:" << std::endl;
for (int i = 0; i < size; ++i) {
std::cout << sum[i] << " ";
}
std::cout << std::endl;
return 0;
}
In this example, we have two arrays array1
and array2
, each containing five integers. We create a third array sum
to store the result of the addition. We iterate through both array1
and array2
using a loop, adding the elements at the same index and storing the result in the corresponding index of the sum
array.
After the addition, we display the elements of the sum
array, which will contain the result of adding array1
and array2
element-wise.
Keep in mind that this code assumes that both array1
and array2
have the same size. If they have different sizes, you may need to handle that situation accordingly to avoid accessing out-of-bounds memory.