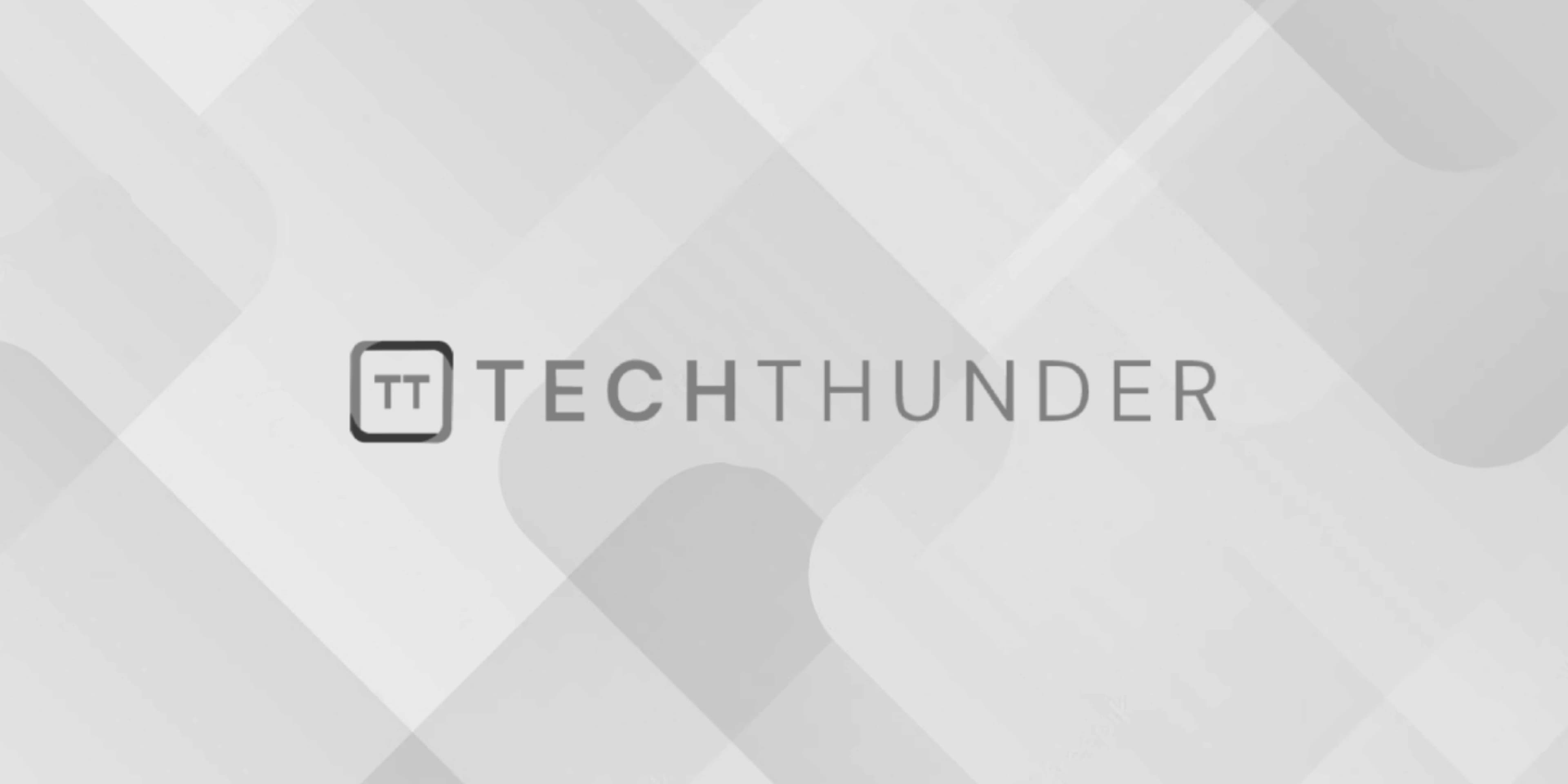
C++ Constructor
The C++ constructor is a special member function of a class that is automatically called when an object of that class is created. The purpose of a constructor is to initialize the object’s data members and prepare the object for use.
Here are some key points about constructors in C++:
- Syntax of a Constructor: A constructor has the same name as the class and does not have a return type, not even
void
.
class MyClass {
public:
MyClass() {
// Constructor code goes here
}
};
In this example, MyClass
has a default constructor.
- Types of Constructors:
- Default Constructor: A constructor that takes no arguments or has all of its parameters with default values.
- Parameterized Constructor: A constructor that takes one or more parameters to initialize the object.
- Copy Constructor: A constructor that creates an object by copying the data of an existing object.
- Copy Assignment Operator: Not technically a constructor, but it performs a similar role by allowing an existing object to be used to initialize another object.
- Default Constructor: If you don’t define any constructors for a class, C++ provides a default constructor automatically. The default constructor initializes the object with default values (e.g., zero for numeric types, null pointers for pointers, etc.).
class MyClass {
public:
// Default constructor provided by the compiler
};
- Parameterized Constructor: You can define constructors with parameters to allow for custom initialization of objects.
class Point {
private:
int x, y;
public:
Point(int a, int b) : x(a), y(b) {
// Constructor code
}
};
In this example, the Point
class has a parameterized constructor that takes two integers and initializes the x
and y
data members.
- Copy Constructor: A copy constructor creates an object by copying an existing object. It is called when an object is initialized with another object of the same type.
class Person {
private:
std::string name;
public:
Person(const Person& other) : name(other.name) {
// Copy constructor code
}
};
The copy constructor takes a reference to another object of the same type and initializes the new object with a copy of the data from the existing object.
- Destructor: A destructor is a special member function used to clean up resources (like dynamic memory allocations) when an object is about to be destroyed. It has the same name as the class, preceded by a tilde (
~
).
class MyClass {
public:
~MyClass() {
// Destructor code goes here
}
};
The destructor is automatically called when an object goes out of scope or when it is explicitly deleted.
Constructors and destructors are crucial for proper object initialization and cleanup, making them an integral part of C++ classes and object-oriented programming.