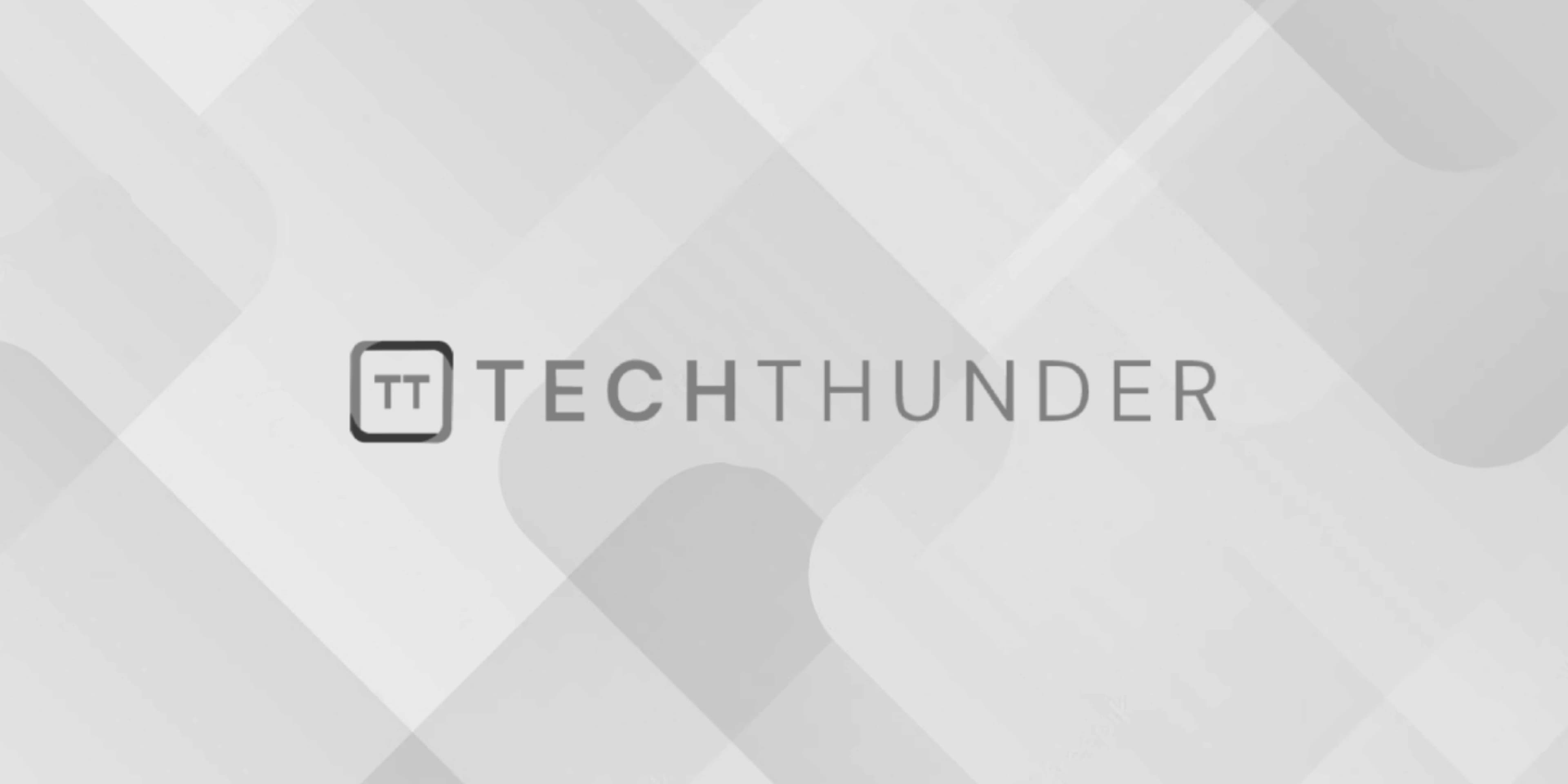
333 views
Add two Numbers using Class in C++
To add two numbers using a C++ class, you can define a class that has methods for setting the numbers, performing the addition, and displaying the result. Here’s an example:
C++
#include <iostream>
class Adder {
public:
// Constructor to initialize the sum to 0
Adder() : sum(0) {}
// Method to set the first number
void setNumber1(double num1) {
number1 = num1;
}
// Method to set the second number
void setNumber2(double num2) {
number2 = num2;
}
// Method to perform the addition
void add() {
sum = number1 + number2;
}
// Method to display the result
void display() {
std::cout << "Sum: " << sum << std::endl;
}
private:
double number1;
double number2;
double sum;
};
int main() {
Adder calculator; // Create an instance of the Adder class
double num1, num2;
// Get user input for the first number
std::cout << "Enter the first number: ";
std::cin >> num1;
// Get user input for the second number
std::cout << "Enter the second number: ";
std::cin >> num2;
// Set the numbers and perform the addition
calculator.setNumber1(num1);
calculator.setNumber2(num2);
calculator.add();
// Display the result
calculator.display();
return 0;
}
In this program:
- We define a
Adder
class that has private member variables fornumber1
,number2
, andsum
. - The class provides public member functions to set the two numbers, perform the addition, and display the result.
- In the
main
function, we create an instance of theAdder
class namedcalculator
. - We use
std::cin
to get user input for the two numbers. - We set the numbers using the
setNumber1
andsetNumber2
methods of thecalculator
object. - We perform the addition using the
add
method. - Finally, we display the result using the
display
method.
This program will add the two numbers and display the sum using a class-based approach.