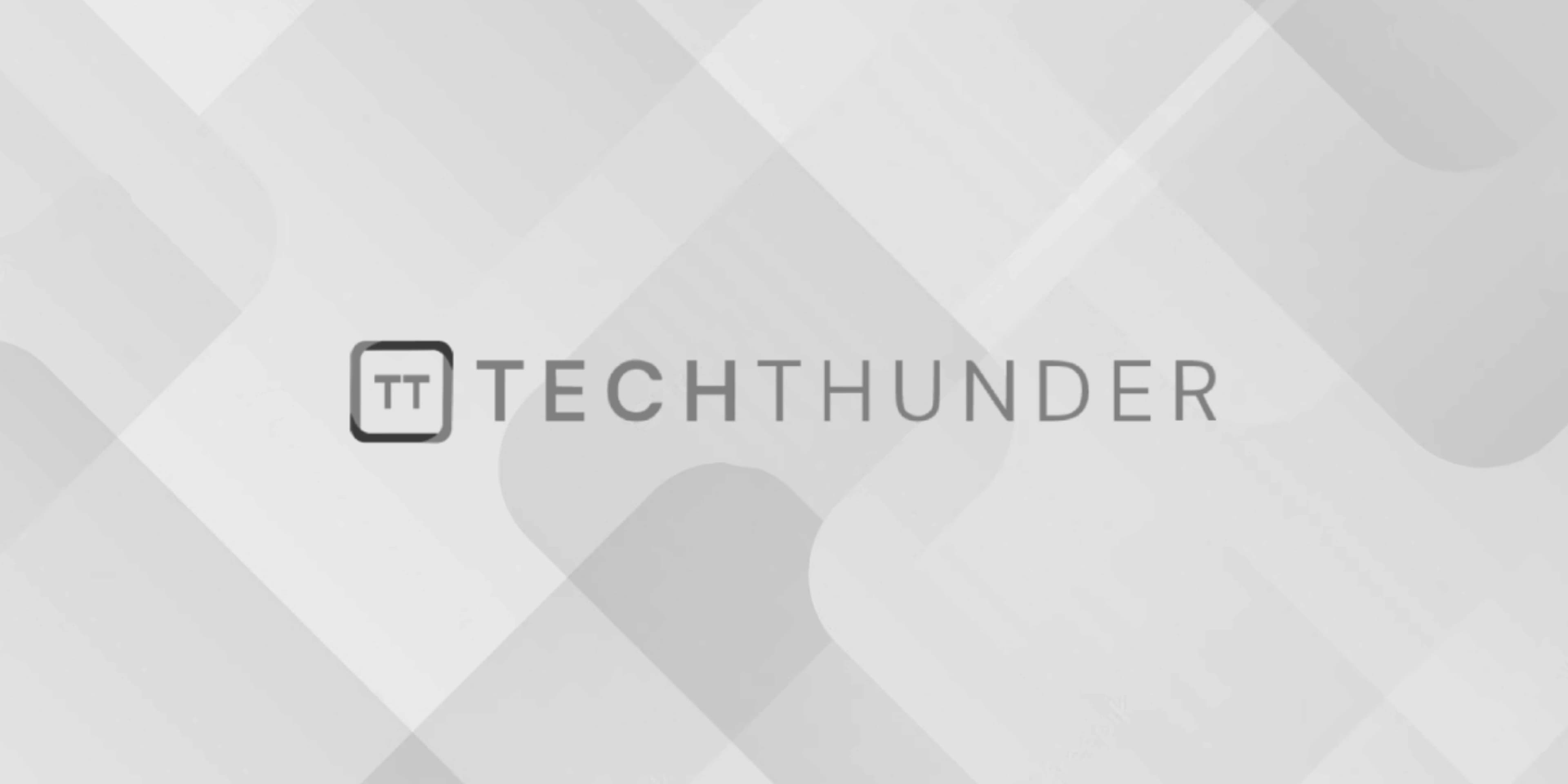
139 views
Associative Containers in C++
The C++ associative containers are a category of containers in the Standard Template Library (STL) that allow for efficient retrieval of elements based on their keys. These containers are implemented as binary search trees or hash tables, which allow for fast lookup times.
Here are the main associative containers in C++:
- std::set:
- Represents a sorted set of unique elements.
- Elements are automatically sorted when inserted.
- Useful when you need to maintain a sorted collection of elements without duplicates. Example:
C++
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {3, 1, 4, 1, 5, 9, 2, 6, 5};
for (int element : mySet) {
std::cout << element << " ";
}
return 0;
}
- std::multiset:
- Allows duplicates and maintains elements in sorted order.
- Useful when you need to maintain a sorted collection that can have duplicates. Example:
C++
#include <iostream>
#include <set>
int main() {
std::multiset<int> myMultiSet = {3, 1, 4, 1, 5, 9, 2, 6, 5};
for (int element : myMultiSet) {
std::cout << element << " ";
}
return 0;
}
- std::map:
- Represents a collection of key-value pairs.
- Elements are sorted by their keys.
- Each key must be unique. Example:
C++
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap = { {"apple", 3}, {"banana", 2}, {"cherry", 5} };
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
- std::multimap:
- Allows multiple elements with the same key.
- Elements are sorted by their keys.
- Useful when you need to store multiple values for the same key. Example:
C++
#include <iostream>
#include <map>
int main() {
std::multimap<std::string, int> myMultiMap = { {"apple", 3}, {"banana", 2}, {"apple", 5}
};
for (const auto& pair : myMultiMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
These containers provide efficient lookup times for elements based on their keys. The choice between them depends on whether you need unique keys, allow duplicates, or need to associate multiple values with the same key.