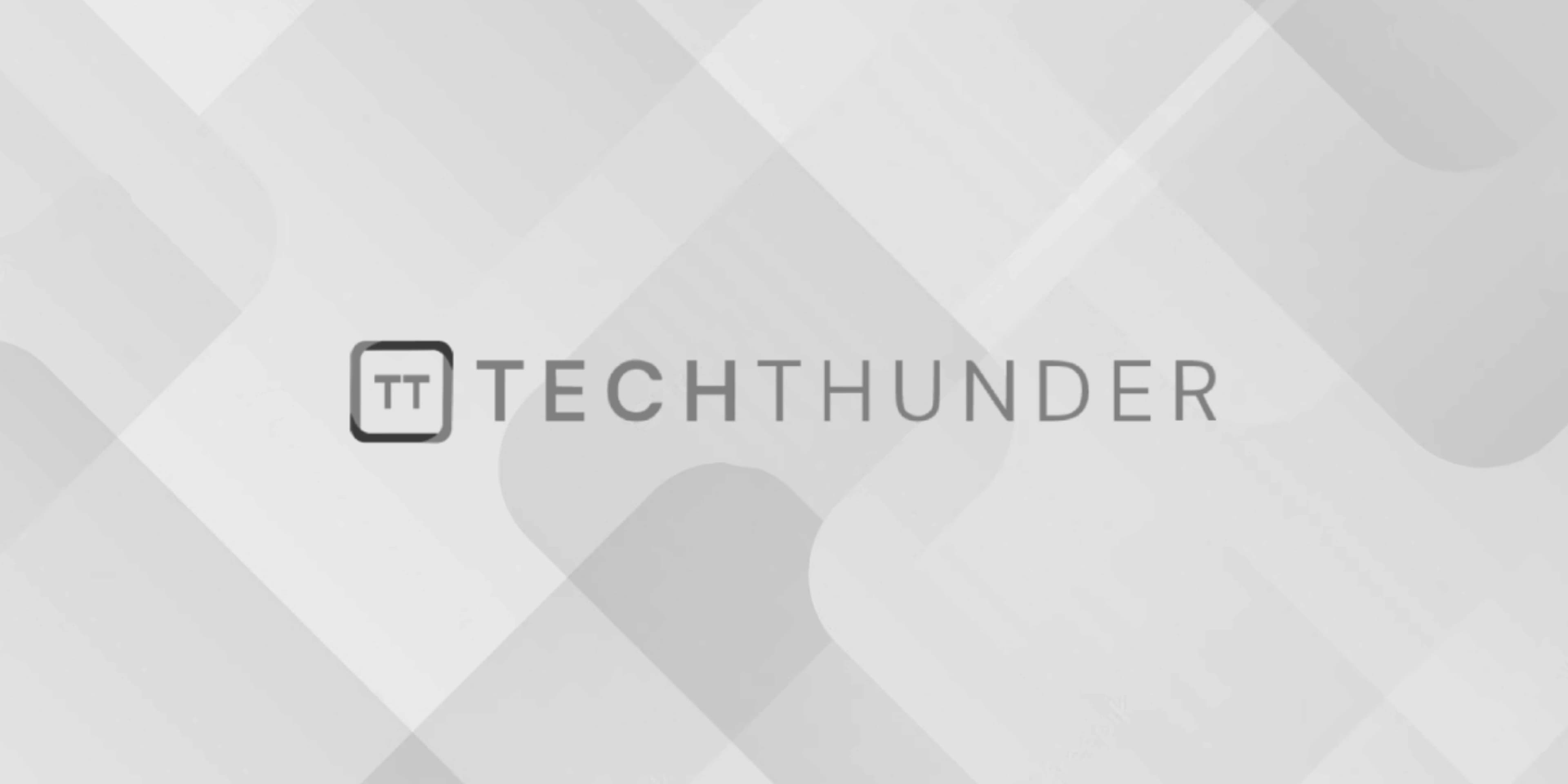
Swap Number in C++
Swapping two numbers in C++ can be done using various methods. Here are three common ways to swap two numbers:
Method 1: Using a Temporary Variable
This is the most straightforward method. You use a temporary variable to store one of the numbers, then assign the value of the second number to the first number, and finally, assign the value of the temporary variable to the second number.
#include <iostream>
int main() {
int a, b;
std::cout << "Enter two numbers: ";
std::cin >> a >> b;
// Swap using a temporary variable
int temp = a;
a = b;
b = temp;
std::cout << "After swapping: a = " << a << ", b = " << b << std::endl;
return 0;
}
Method 2: Using Arithmetic Operations
You can swap two numbers without a temporary variable by using arithmetic operations (addition and subtraction). This method works for integer and floating-point numbers.
#include <iostream>
int main() {
double a, b;
std::cout << "Enter two numbers: ";
std::cin >> a >> b;
// Swap using arithmetic operations
a = a + b;
b = a - b;
a = a - b;
std::cout << "After swapping: a = " << a << ", b = " << b << std::endl;
return 0;
}
Method 3: Using Bitwise XOR
You can swap two integers without using a temporary variable by applying the bitwise XOR operation. This method works only for integer values.
#include <iostream>
int main() {
int a, b;
std::cout << "Enter two numbers: ";
std::cin >> a >> b;
// Swap using bitwise XOR
a = a ^ b;
b = a ^ b;
a = a ^ b;
std::cout << "After swapping: a = " << a << ", b = " << b << std::endl;
return 0;
}
All three methods will effectively swap the values of a
and b
. Choose the one that suits your specific needs or constraints.