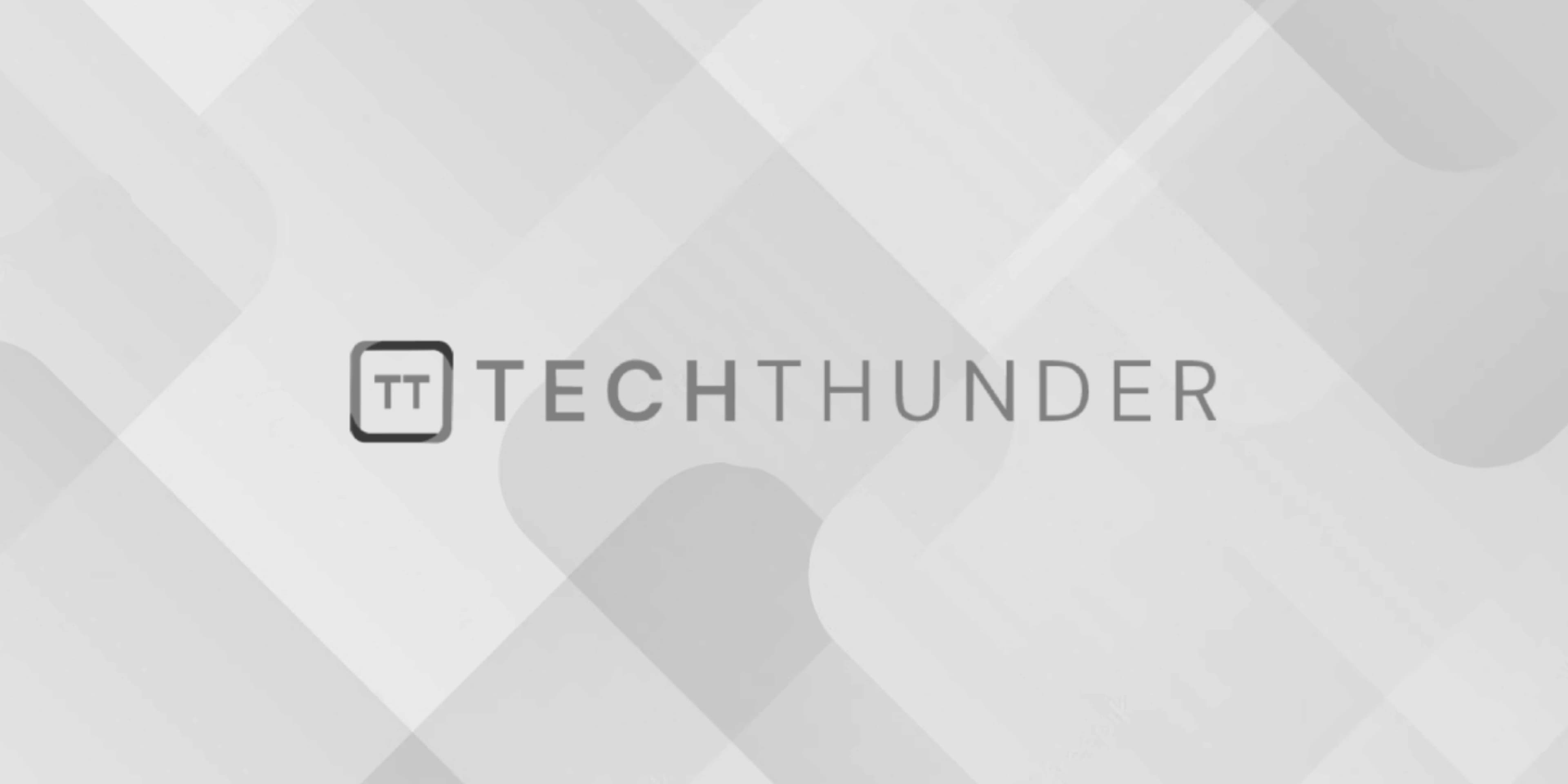
266 views
Snake Code in C++
Implementing a simple Snake game in C++ can be a fun project. Below is a basic example of how you can create a text-based Snake game using the console. This example uses the Windows Console API to manipulate the console for display and input. Note that this code is simplified for educational purposes and may not include all the features of a full-fledged Snake game.
C++
#include <iostream>
#include <conio.h> // For _getch() and _kbhit() on Windows
using namespace std;
bool gameOver;
const int width = 20;
const int height = 10;
int x, y, fruitX, fruitY, score;
int tailX[100], tailY[100];
int nTail;
enum eDirection { STOP = 0, LEFT, RIGHT, UP, DOWN };
eDirection dir;
// Setup function
void Setup() {
gameOver = false;
dir = STOP;
x = width / 2;
y = height / 2;
fruitX = rand() % width;
fruitY = rand() % height;
score = 0;
}
// Draw function
void Draw() {
system("cls"); // Clear the console
// Draw the top border
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
// Draw the playing field
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (j == 0)
cout << "#"; // Left border
if (i == y && j == x)
cout << "O"; // Snake's head
else if (i == fruitY && j == fruitX)
cout << "F"; // Fruit
else {
bool printTail = false;
for (int k = 0; k < nTail; k++) {
if (tailX[k] == j && tailY[k] == i) {
cout << "o"; // Snake's tail
printTail = true;
}
}
if (!printTail)
cout << " ";
}
if (j == width - 1)
cout << "#"; // Right border
}
cout << endl;
}
// Draw the bottom border
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
cout << "Score:" << score << endl;
}
// Input function
void Input() {
if (_kbhit()) {
switch (_getch()) {
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'x':
gameOver = true;
break;
}
}
}
// Logic function
void Logic() {
int prevX = tailX[0];
int prevY = tailY[0];
int prev2X, prev2Y;
tailX[0] = x;
tailY[0] = y;
for (int i = 1; i < nTail; i++) {
prev2X = tailX[i];
prev2Y = tailY[i];
tailX[i] = prevX;
tailY[i] = prevY;
prevX = prev2X;
prevY = prev2Y;
}
switch (dir) {
case LEFT:
x--;
break;
case RIGHT:
x++;
break;
case UP:
y--;
break;
case DOWN:
y++;
break;
default:
break;
}
if (x >= width) x = 0; else if (x < 0) x = width - 1;
if (y >= height) y = 0; else if (y < 0) y = height - 1;
for (int i = 0; i < nTail; i++)
if (tailX[i] == x && tailY[i] == y)
gameOver = true;
if (x == fruitX && y == fruitY) {
score += 10;
fruitX = rand() % width;
fruitY = rand() % height;
nTail++;
}
}
int main() {
Setup();
while (!gameOver) {
Draw();
Input();
Logic();
// Sleep(10); // Sleep for some milliseconds
}
return 0;
}
This code represents a simple text-based Snake game in C++. It uses the conio.h
library for keyboard input and the Windows Console API for console manipulation. Note that this is a minimal implementation, and you can expand it by adding more features such as increasing difficulty levels, speed control, and better graphics.