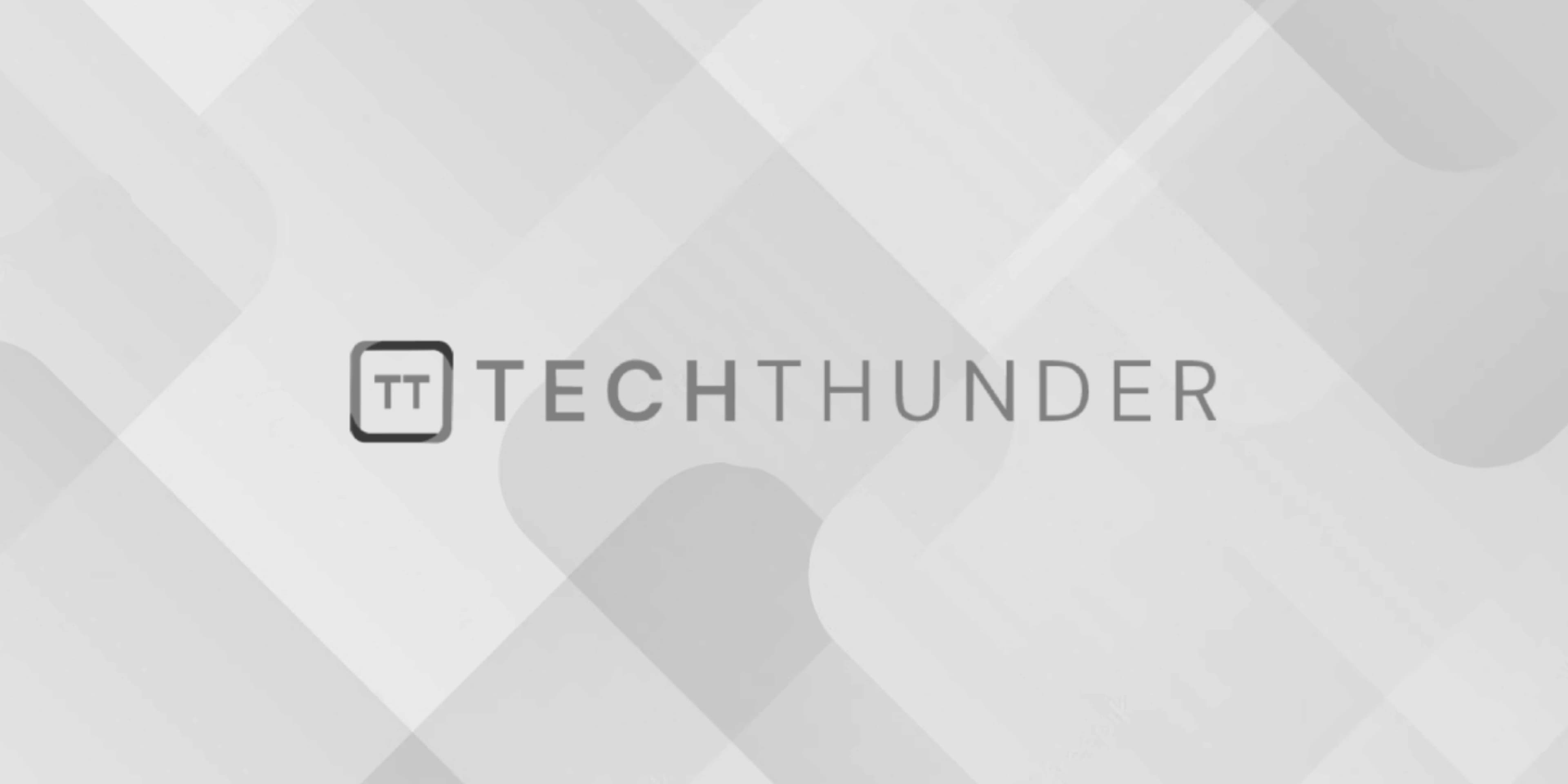
C++ Polymorphism
Polymorphism is one of the key principles of object-oriented programming (OOP) and is supported in C++. It allows objects of different classes to be treated as objects of a common base class. Polymorphism enables you to write more flexible and extensible code by providing a way to work with objects in a uniform and abstract manner, regardless of their specific types.
There are two main types of polymorphism in C++: compile-time (or static) polymorphism and runtime (or dynamic) polymorphism. Let’s explore both of them:
- Compile-Time Polymorphism: Compile-time polymorphism, also known as static polymorphism or method overloading, occurs when multiple functions with the same name exist in the same scope, but they have different parameter lists. The appropriate function to call is determined at compile time based on the function’s signature and the arguments provided during the function call.
// Function overloading
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
In this example, the compiler selects the appropriate add
function based on the argument types. This is known as function overloading and is a form of compile-time polymorphism.
- Runtime Polymorphism: Runtime polymorphism, also known as dynamic polymorphism, occurs when different classes are related through inheritance and they override a common base class’s virtual functions. The specific function to call is determined at runtime based on the actual object type.
class Animal {
public:
virtual void makeSound() {
std::cout << "Animal makes a sound" << std::endl;
}
};
class Dog : public Animal {
public:
void makeSound() override {
std::cout << "Dog barks" << std::endl;
}
};
class Cat : public Animal {
public:
void makeSound() override {
std::cout << "Cat meows" << std::endl;
}
};
In this example, Dog
and Cat
are derived classes of Animal
, and they override the makeSound
function. At runtime, the appropriate makeSound
function is called based on the actual object type.
int main() {
Animal* animalPtr;
Dog dog;
Cat cat;
animalPtr = &dog;
animalPtr->makeSound(); // Calls Dog's makeSound
animalPtr = &cat;
animalPtr->makeSound(); // Calls Cat's makeSound
return 0;
}
In this code, even though animalPtr
is a pointer to an Animal
, it calls the appropriate makeSound
function based on the actual object type (polymorphism).
Polymorphism is a powerful concept that promotes code reusability and flexibility. It allows you to create classes with common interfaces and override methods to provide specialized behavior in derived classes. This enables you to write code that works with objects at a high level of abstraction, making it easier to extend and maintain your software.