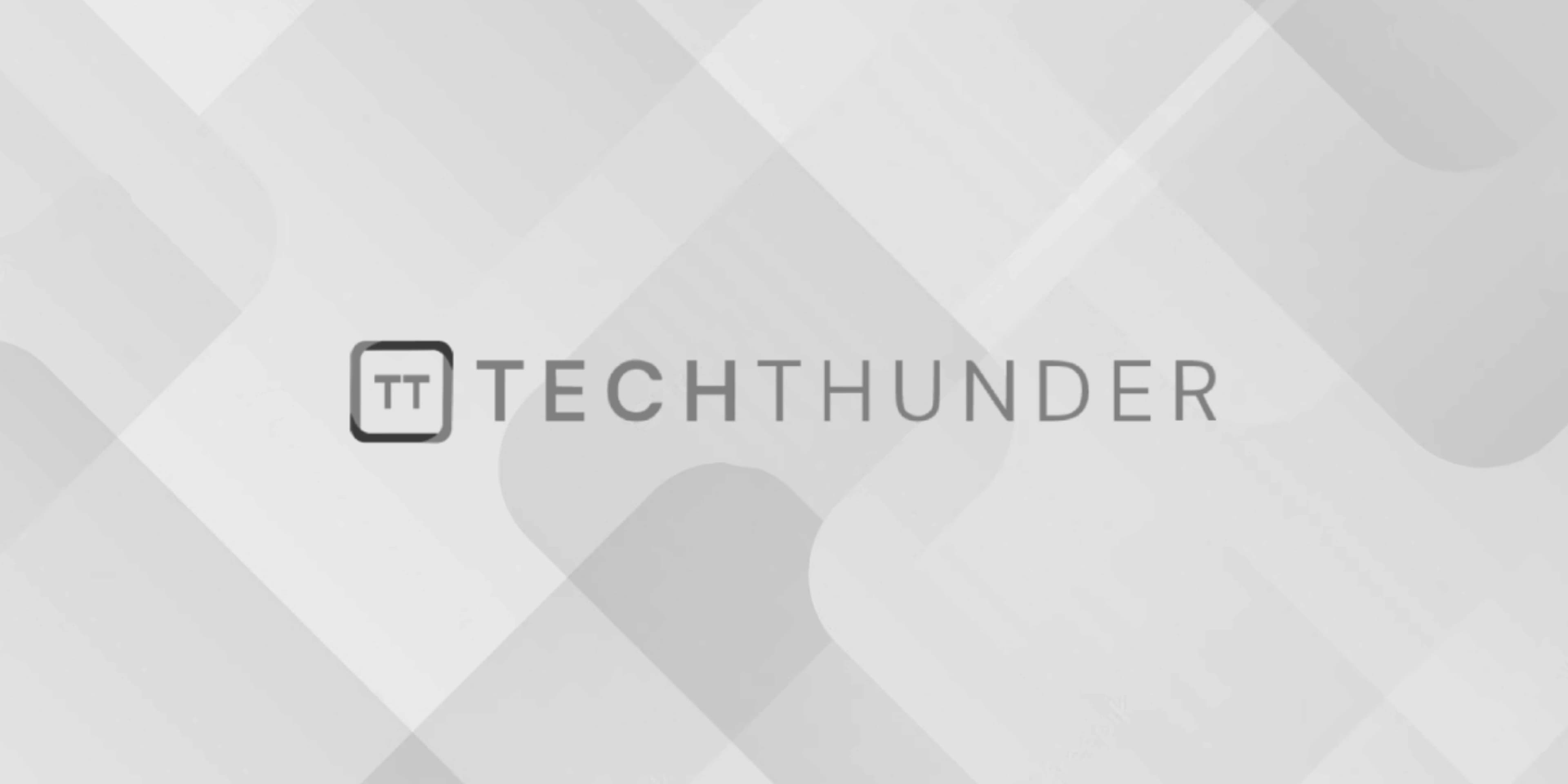
160 views
Find max in Array Function C++
You can find the maximum value in an array in C++ by iterating through the array and keeping track of the maximum value encountered so far. Here’s a simple function to do that:
C++
#include <iostream>
// Function to find the maximum value in an array
int findMax(const int arr[], int size) {
if (size <= 0) {
// Handle the case of an empty array or invalid size
throw std::invalid_argument("Invalid array size");
}
int maxVal = arr[0]; // Assume the first element is the maximum
for (int i = 1; i < size; ++i) {
if (arr[i] > maxVal) {
maxVal = arr[i]; // Update maxVal if a larger element is found
}
}
return maxVal;
}
int main() {
int arr[] = {12, 45, 6, 78, 32, 56, 89, 21};
int size = sizeof(arr) / sizeof(arr[0]);
try {
int max = findMax(arr, size);
std::cout << "The maximum value in the array is: " << max << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << e.what() << std::endl;
}
return 0;
}
In this code:
- The
findMax
function takes an integer arrayarr
and its size as input parameters. - It initializes
maxVal
with the first element of the array and then iterates through the array. - If a larger value is encountered during the iteration,
maxVal
is updated. - The
main
function demonstrates how to use thefindMax
function with an example array.
This code will find the maximum value in the array and handle cases where the array is empty or the size is invalid.