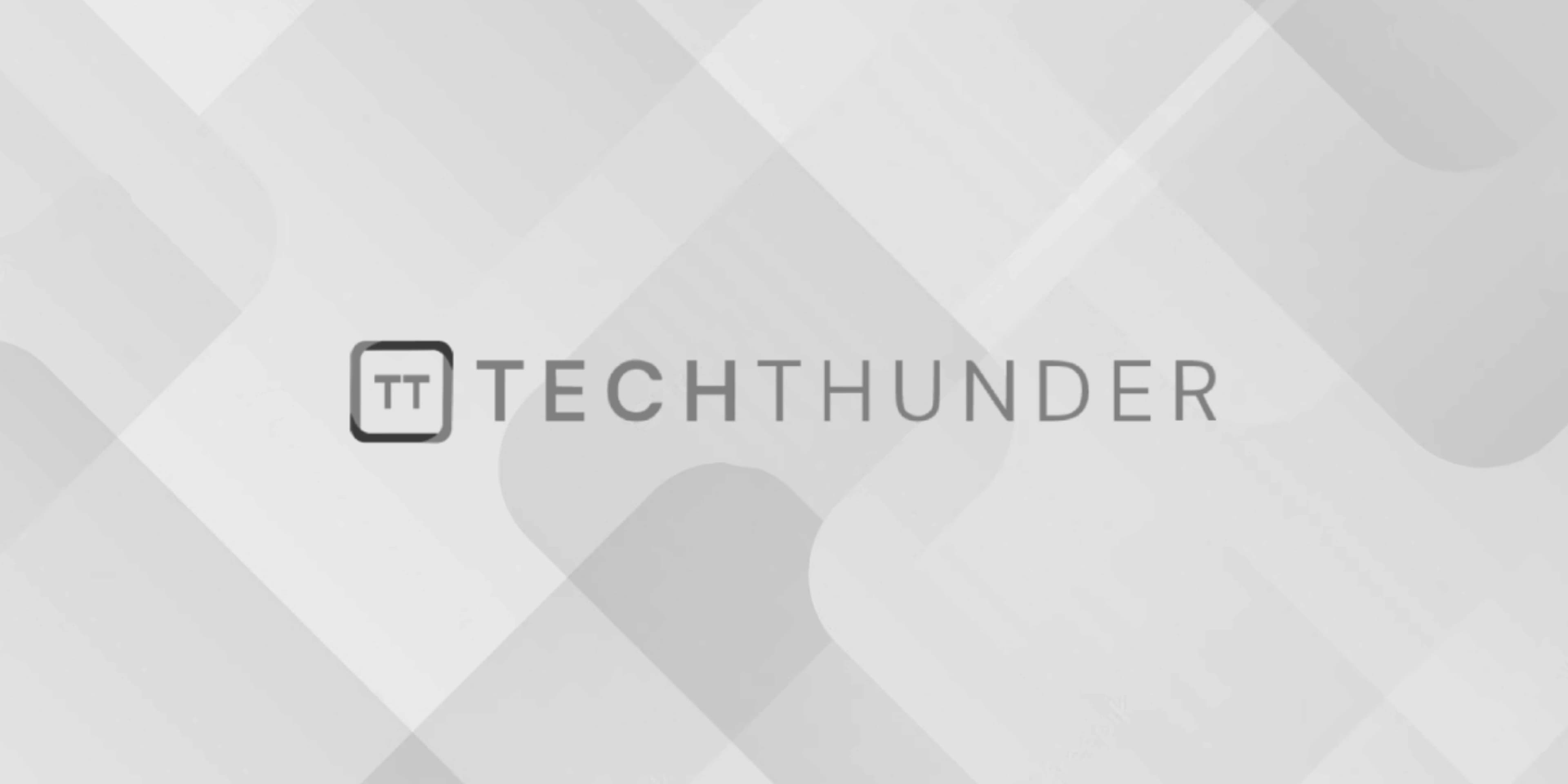
242 views
PID Controller C++
Creating a PID controller in C++ involves implementing a class or a set of functions to handle the control logic. A PID controller is commonly used in control systems to regulate a process by adjusting a control input based on the error (the difference between the desired setpoint and the actual process variable). Here’s a simple example of a PID controller in C++:
C++
#include <iostream>
class PIDController {
public:
PIDController(double kp, double ki, double kd)
: kp_(kp), ki_(ki), kd_(kd), prev_error_(0), integral_(0) {}
double calculate(double setpoint, double process_variable) {
double error = setpoint - process_variable;
integral_ += error;
double derivative = error - prev_error_;
prev_error_ = error;
double output = kp_ * error + ki_ * integral_ + kd_ * derivative;
return output;
}
private:
double kp_; // Proportional gain
double ki_; // Integral gain
double kd_; // Derivative gain
double prev_error_; // Previous error
double integral_; // Integral of errors
};
int main() {
// Example usage of the PID controller
PIDController pid(0.5, 0.2, 0.1); // You can adjust these gains accordingly
double setpoint = 50.0; // Desired setpoint
double process_variable = 0.0; // Initial process variable
for (int i = 0; i < 100; ++i) {
double control_output = pid.calculate(setpoint, process_variable);
// Simulate the process by updating the process_variable
// In a real system, this value would come from a sensor
process_variable += control_output;
std::cout << "Iteration " << i << ": Setpoint=" << setpoint
<< ", Process Variable=" << process_variable
<< ", Control Output=" << control_output << std::endl;
}
return 0;
}
In this example:
- The
PIDController
class takes the proportional (kp), integral (ki), and derivative (kd) gains as parameters in its constructor. - The
calculate
method computes the control output based on the current error, integral of errors, and derivative of the error. - In the
main
function, we create an instance of the PID controller and use it to control a simulated process variable.
Remember to adjust the PID gains (kp, ki, and kd) according to your specific control system requirements. Additionally, in a real-world application, you would replace the simulated process variable update with actual sensor readings and control of a physical system.