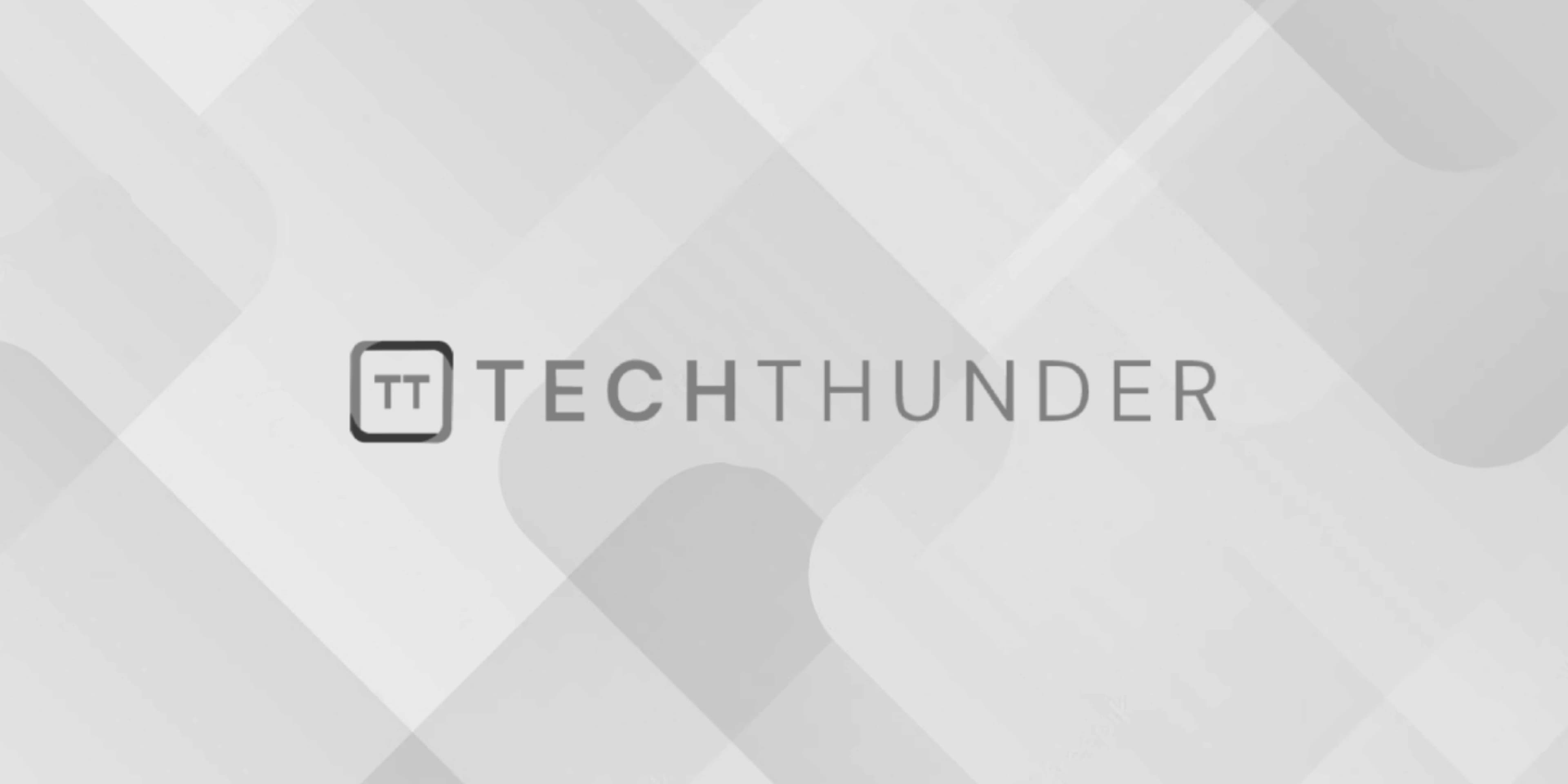
Smart Pointer in C++
Smart pointers in C++ are a set of classes that provide automatic memory management for dynamically allocated objects. They help avoid common issues associated with manual memory management, such as memory leaks and undefined behavior. Smart pointers are part of the C++ Standard Library and are defined in the <memory>
header. There are three main types of smart pointers in C++:
std::unique_ptr
:
std::unique_ptr
is a smart pointer that manages the ownership of a dynamically allocated object. It ensures that only onestd::unique_ptr
instance owns the object, and when that instance goes out of scope, the object is automatically deleted. Example ofstd::unique_ptr
:
#include <memory>
std::unique_ptr<int> ptr = std::make_unique<int>(42);
// When ptr goes out of scope, the int is automatically deleted.
std::shared_ptr
:
std::shared_ptr
is a smart pointer that allows multiple instances ofstd::shared_ptr
to share ownership of the same dynamically allocated object. The object is deleted only when the laststd::shared_ptr
owning it goes out of scope. Example ofstd::shared_ptr
:
#include <memory>
std::shared_ptr<int> ptr1 = std::make_shared<int>(42);
std::shared_ptr<int> ptr2 = ptr1; // Shared ownership
// When both ptr1 and ptr2 go out of scope, the int is deleted.
std::weak_ptr
:
std::weak_ptr
is used in conjunction withstd::shared_ptr
to break circular references. It provides a way to observe an object owned by one or morestd::shared_ptr
instances without affecting the object’s lifetime. It does not increase the reference count of the shared object. Example ofstd::weak_ptr
:
#include <memory>
std::shared_ptr<int> sharedPtr = std::make_shared<int>(42);
std::weak_ptr<int> weakPtr = sharedPtr;
// The object's lifetime is not extended by the weakPtr.
Smart pointers simplify memory management and help prevent memory leaks, as they automatically release memory when it is no longer needed. They also provide a level of safety and exception safety compared to manual memory management using raw pointers and new
/delete
.
It’s recommended to use smart pointers whenever possible to manage dynamic memory in C++ as they can significantly reduce the chances of memory-related bugs and make code more robust and maintainable.