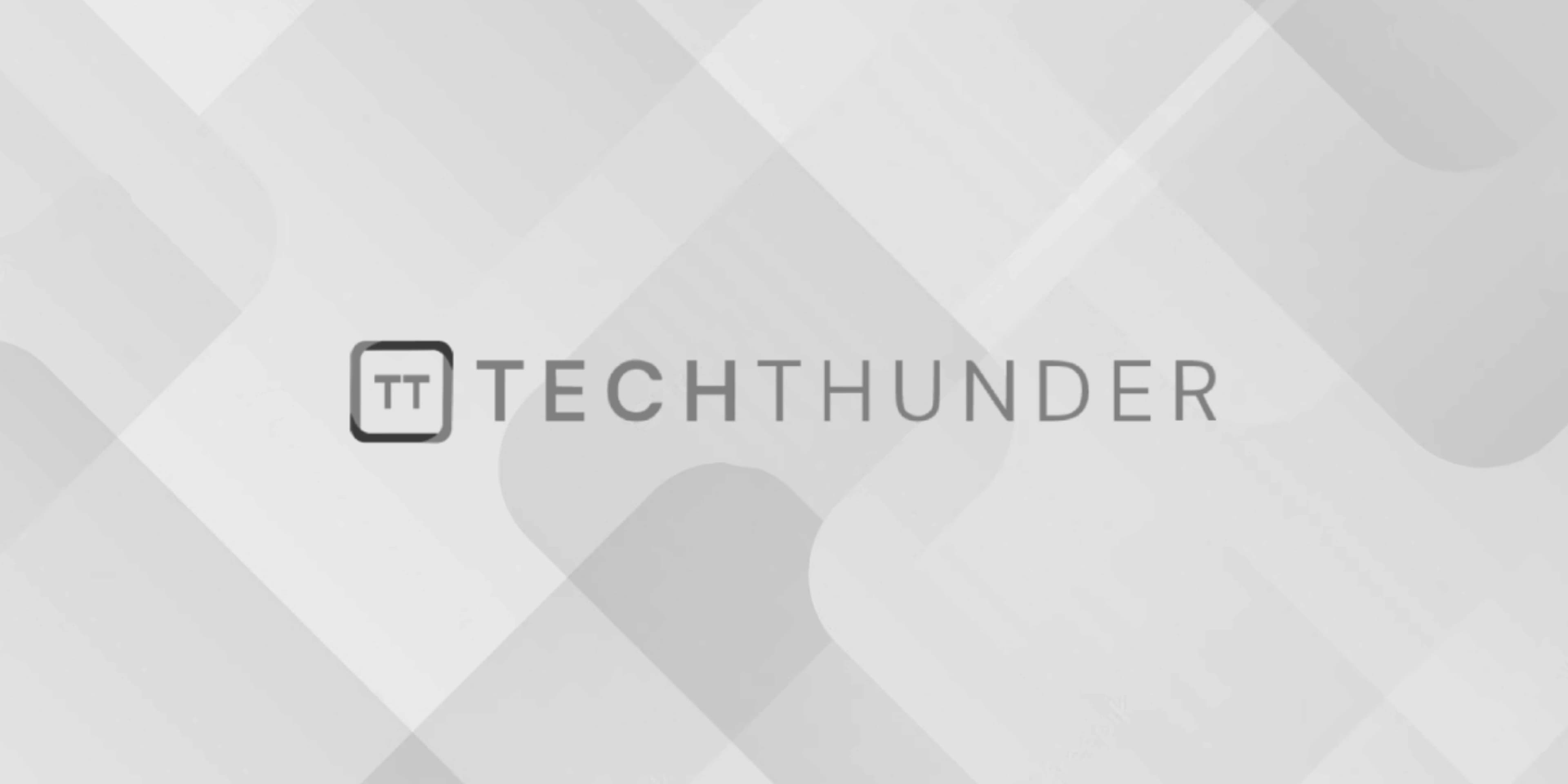
183 views
Pointers such as Dangling, Void, Null, and Wild in C++
The C++ pointers are a fundamental concept for working with memory and data structures. There are several types of pointers, including dangling pointers, void pointers, null pointers, and wild pointers. Let’s explore each of them:
- Dangling Pointers:
- A dangling pointer is a pointer that points to a memory location that has been deallocated or freed.
- Dangling pointers often occur when a pointer is pointing to a memory address that was valid at some point but is no longer valid because the memory has been released.
- Accessing or modifying the memory through a dangling pointer can lead to undefined behavior and can be a source of difficult-to-debug errors.
- Void Pointers:
- A void pointer is a special type of pointer that doesn’t have a specific data type associated with it.
- You can use a void pointer to store the address of any data type, but you need to explicitly cast it to the appropriate data type before dereferencing it.
- Void pointers are often used in C and C++ for functions that need to work with generic data or for dynamic memory allocation functions like
malloc()
andfree()
, which return and accept void pointers.
- Null Pointers:
- A null pointer is a pointer that does not point to any memory location.
- In C++ (and C), you can assign a pointer the value
nullptr
to indicate that it doesn’t point to anything. - Using a null pointer is generally safe, as long as you check for it before dereferencing it to avoid a segmentation fault or undefined behavior.
- Wild Pointers:
- A wild pointer is a pointer that is not initialized or set to a specific memory address.
- Using a wild pointer can lead to undefined behavior because you are trying to access memory that is not guaranteed to be allocated for your program.
- It’s essential to initialize pointers before using them to avoid wild pointer issues.
Here’s a brief example to illustrate these concepts:
C++
int* danglingPtr; // This pointer is uninitialized and contains garbage value.
int* validPtr = nullptr; // This pointer is explicitly set to nullptr.
int* wildPtr; // This pointer is uninitialized (wild), and its value is unpredictable.
void* genericPtr = nullptr; // This is a void pointer, often used for generic data.
if (validPtr != nullptr) {
*validPtr = 42; // Safe to use, as it's initialized and not a dangling pointer.
}
if (wildPtr != nullptr) {
*wildPtr = 10; // Dangerous! Wild pointer; undefined behavior may occur.
}
if (danglingPtr != nullptr) {
*danglingPtr = 20; // Dangerous! Dangling pointer; undefined behavior may occur.
}
if (genericPtr != nullptr) {
// To use a void pointer, you need to cast it to the appropriate type.
int* intPtr = static_cast<int*>(genericPtr);
*intPtr = 30;
}
It’s crucial to manage pointers carefully to avoid memory-related issues and undefined behavior in your C++ programs. Using nullptr for null pointers and initializing pointers properly can help prevent many common pointer-related problems.