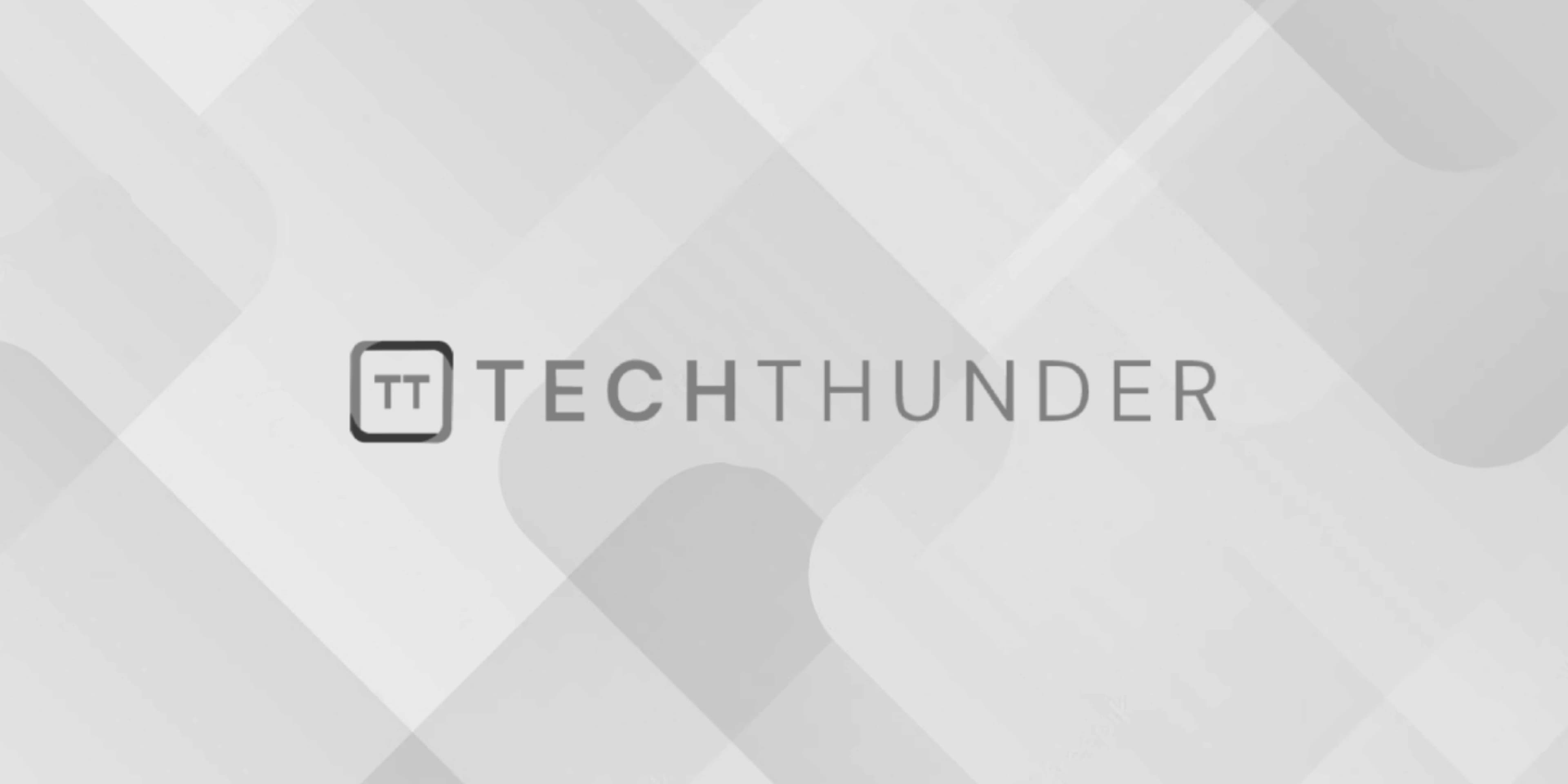
170 views
Array sum in C++
To calculate the sum of elements in an array in C++, you can use a loop to iterate through the array and accumulate the values. Here’s a simple example:
C++
#include <iostream>
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]); // Calculate the size of the array
int sum = 0;
// Loop through the array and calculate the sum
for (int i = 0; i < size; ++i) {
sum += arr[i];
}
std::cout << "Sum of elements in the array: " << sum << std::endl;
return 0;
}
In this example:
- We define an integer array
arr
with some values. - We calculate the size of the array using the
sizeof
operator divided by the size of a single element of the array. - We initialize a variable
sum
to zero to store the sum of elements. - We use a
for
loop to iterate through the array, adding each element to thesum
variable. - Finally, we print the sum of the elements to the console.
This is a basic example, and you can adapt it to work with arrays of different types and sizes as needed. Additionally, you can use standard library functions like std::accumulate
to simplify the sum calculation for more complex cases. Here’s an example using std::accumulate
:
C++
#include <iostream>
#include <numeric> // Include the numeric header for std::accumulate
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
int sum = std::accumulate(arr, arr + size, 0);
std::cout << "Sum of elements in the array: " << sum << std::endl;
return 0;
}
The std::accumulate
function takes an array or range of elements, starting and ending iterators, and an initial value for accumulation. It simplifies the sum calculation by encapsulating the loop logic.