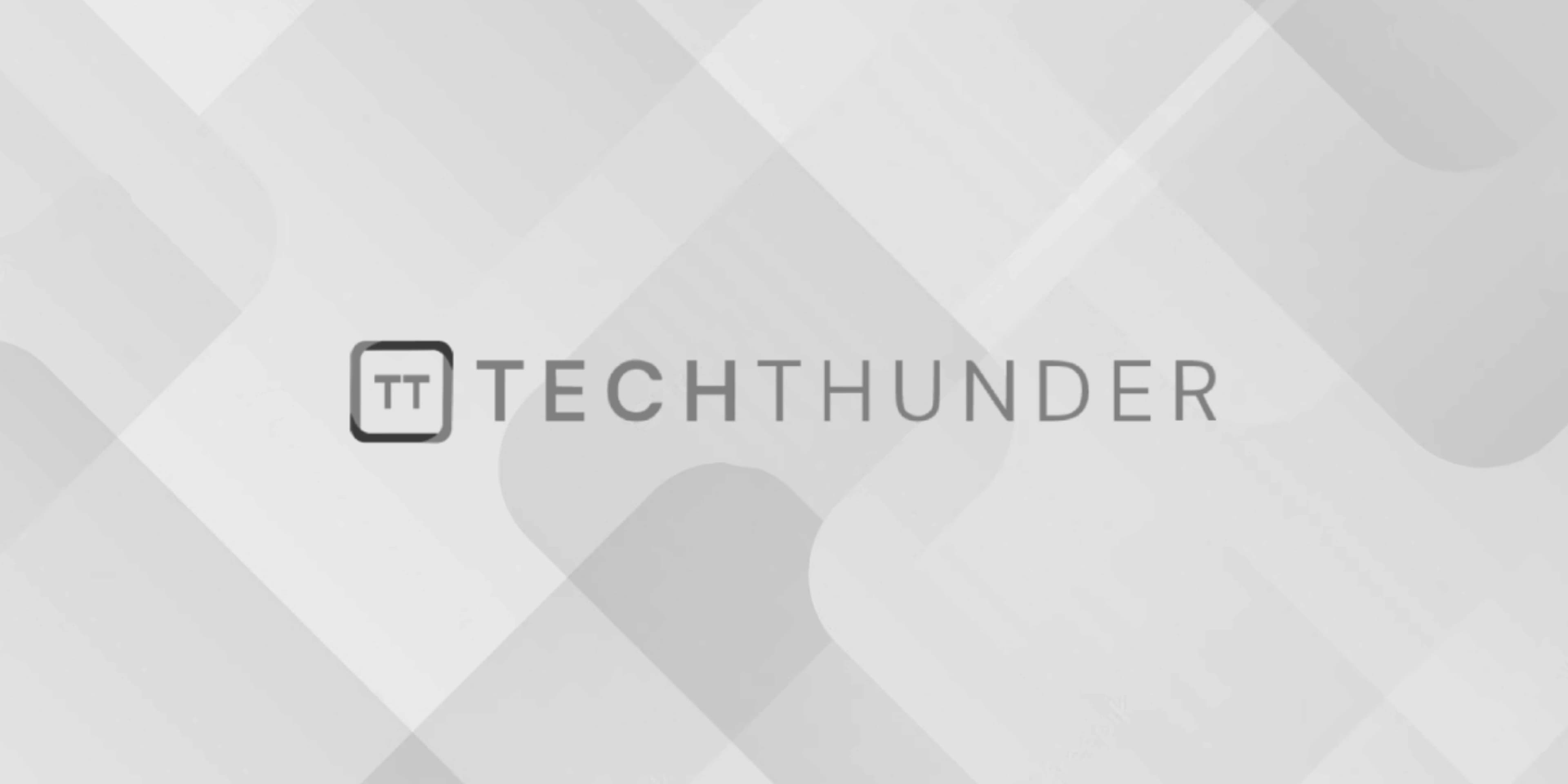
262 views
C++ program to group anagrams in the given stream of strings
The C++ program that takes a stream of strings as input and groups the anagrams together:
C++
#include <iostream>
#include <vector>
#include <unordered_map>
#include <algorithm>
using namespace std;
string sortString(string s) {
sort(s.begin(), s.end());
return s;
}
int main() {
unordered_map<string, vector<string>> anagramGroups;
int n;
cout << "Enter the number of strings: ";
cin >> n;
cin.ignore(); // Clear newline from buffer
for (int i = 0; i < n; ++i) {
string str;
cout << "Enter string " << i + 1 << ": ";
getline(cin, str);
string sortedStr = sortString(str);
anagramGroups[sortedStr].push_back(str);
}
cout << "Anagram groups:" << endl;
for (const auto& group : anagramGroups) {
cout << "Group: ";
for (const string& str : group.second) {
cout << str << " ";
}
cout << endl;
}
return 0;
}
Copy and paste this code into a .cpp
file and compile it using a C++ compiler. The program first sorts the characters in each string and then uses an unordered map to group anagrams together. Finally, it prints out the groups of anagrams.
Remember to adjust the input and output handling based on your requirements. This program assumes that each string is entered on a new line.