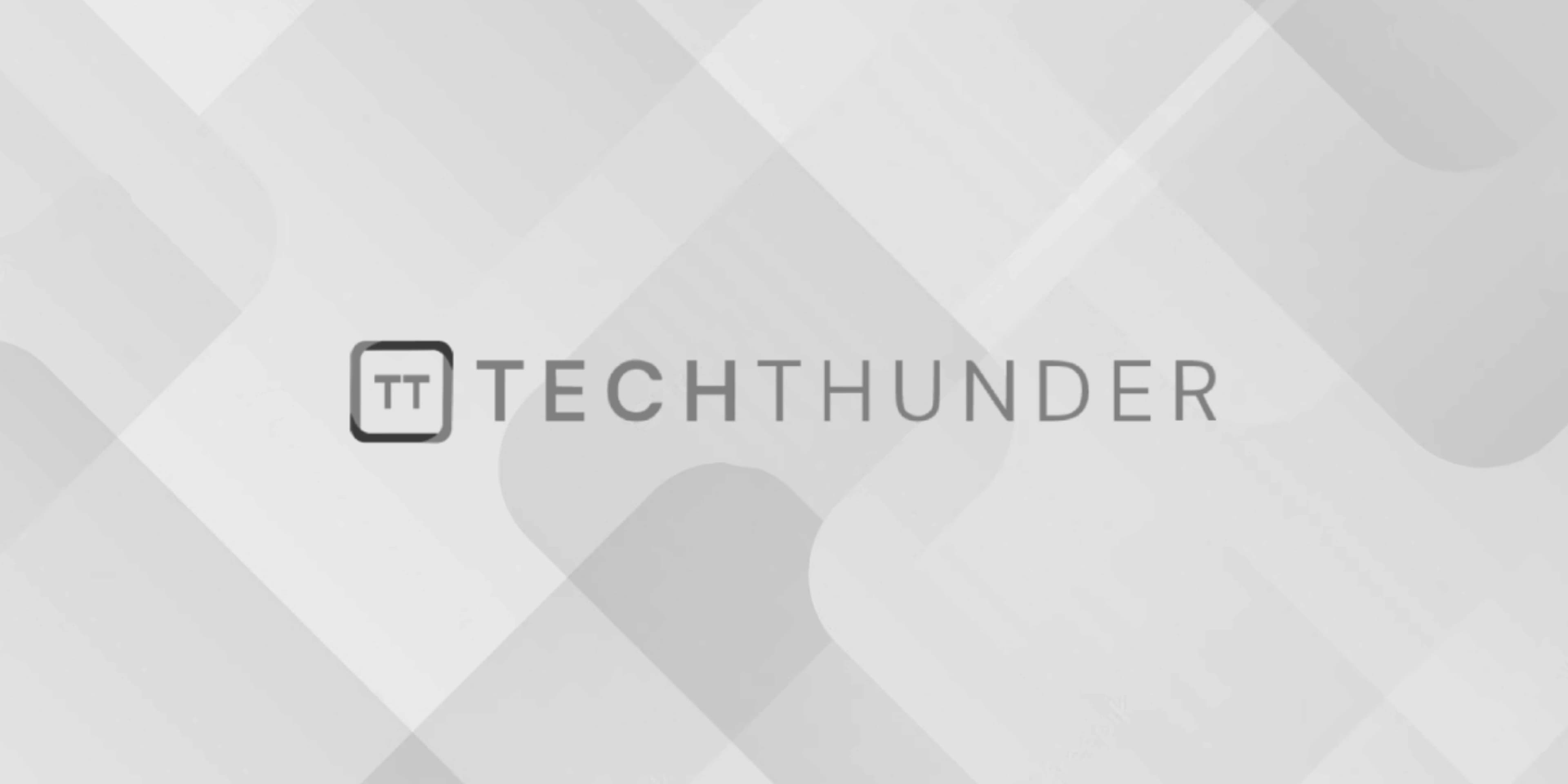
C++ Tutorial
Creating a comprehensive C++ tutorial is beyond the scope of a single response, but I can provide you with an outline of topics you might want to cover in a beginner’s C++ tutorial. You can explore these topics in more detail through online resources, textbooks, and coding exercises. Here’s a suggested outline:
1. Introduction to C++:
- What is C++?
- History and significance of C++.
- Setting up a C++ development environment (IDEs, compilers).
2. Basic C++ Syntax:
- Writing and running a “Hello, World!” program.
- Structure of a C++ program.
- Comments and basic code organization.
3. Variables and Data Types:
- Declaring and initializing variables.
- Fundamental data types (int, float, double, char, bool).
- Type modifiers (signed, unsigned, long, short).
4. Input and Output:
- Using
cin
andcout
for console input and output. - Formatting output with
setw
,setprecision
, etc.
5. Operators:
- Arithmetic operators (+, -, *, /, %).
- Relational operators (==, !=, <, >, <=, >=).
- Logical operators (&&, ||, !).
- Assignment operators (=, +=, -=, *=, /=, %=).
- Increment (++) and decrement (–) operators.
6. Control Flow:
if
,else if
, andelse
statements.switch
andcase
statements.for
,while
, anddo-while
loops.break
andcontinue
statements.
7. Functions:
- Defining and calling functions.
- Function parameters and return values.
- Function overloading.
- Function prototypes.
8. Arrays and Strings:
- Declaring and initializing arrays.
- Accessing array elements.
- Manipulating strings.
9. Pointers and References:
- Understanding memory addresses and pointers.
- Pointer arithmetic.
- Using references to avoid copying data.
10. Object-Oriented Programming (OOP):
– Introduction to classes and objects.
– Constructors and destructors.
– Encapsulation, inheritance, and polymorphism.
11. Standard Template Library (STL):
– Introduction to containers (vectors, lists, etc.).
– Algorithms (sorting, searching, etc.).
– Iterators.
12. Exception Handling:
– Handling exceptions using try
, catch
, and throw
.
– Custom exception classes.
13. File Input and Output:
– Reading and writing files with ifstream
and ofstream
.
14. Advanced C++ Concepts (Optional):
– Templates and generic programming.
– Lambda expressions.
– Smart pointers (shared_ptr, unique_ptr).
– Move semantics and rvalue references.
15. Practice and Projects:
– Encourage learners to work on small coding projects to apply their knowledge.
– Suggest coding exercises and challenges.
16. Debugging and Troubleshooting:
– Tips for debugging C++ programs.
– Common programming errors and how to avoid them.
17. Resources and Further Learning:
– Provide recommendations for C++ books, online tutorials, and forums for learners to continue their education.
Remember that learning programming is most effective when you combine reading and studying with hands-on practice. Encourage learners to write code regularly and work on real projects to reinforce their understanding of C++.