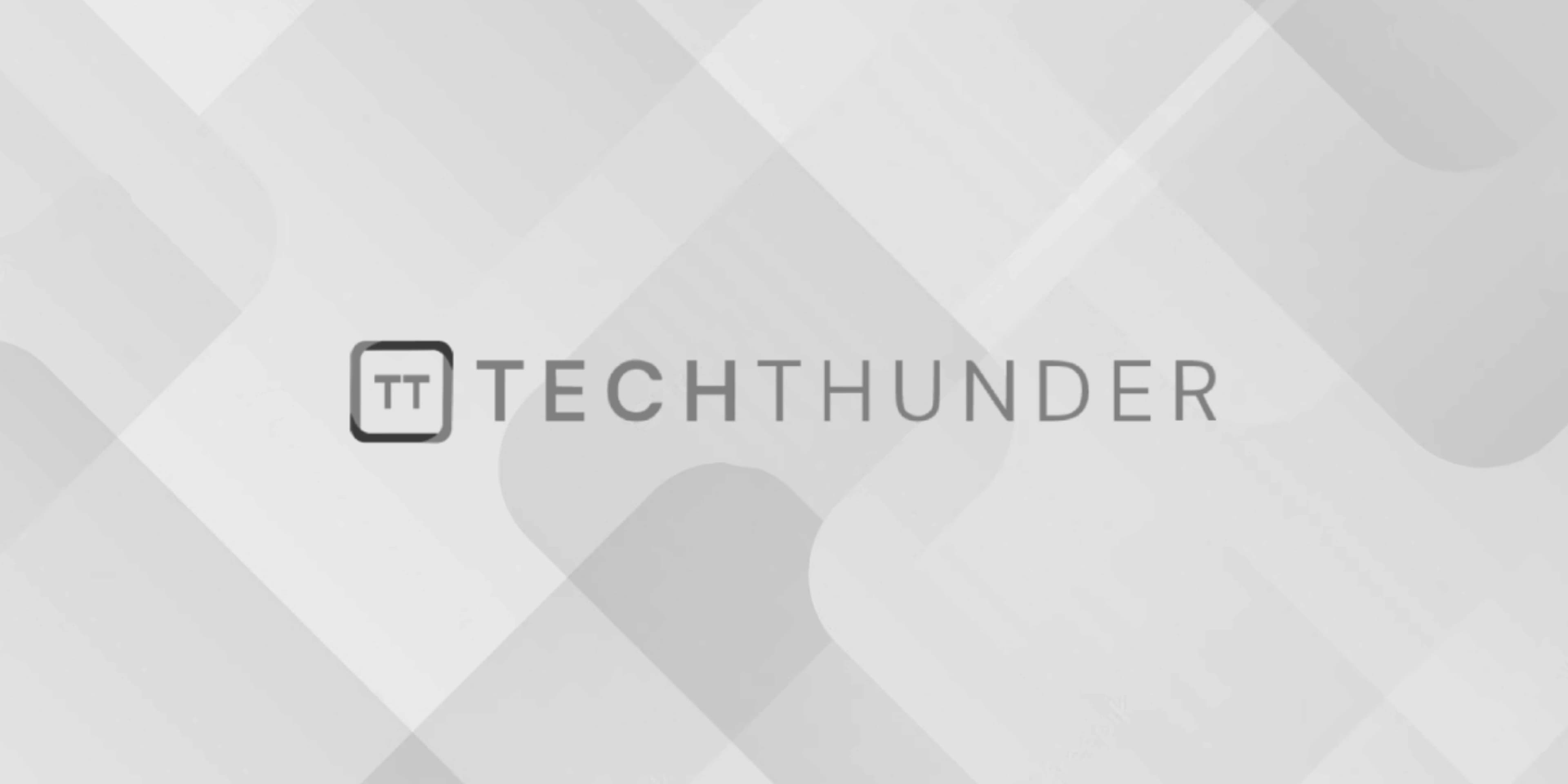
Catching Base and Derived Classes as Exceptions in C++ and Java
The both C++ and Java, you can catch both base and derived classes as exceptions using try-catch blocks. This allows you to handle exceptions at different levels of specificity, from the most specific (derived class) to the more general (base class).
In C++:
In C++, you can catch exceptions by their base and derived types using catch blocks. Here’s an example:
#include <iostream>
#include <stdexcept>
class MyBaseException : public std::exception {
public:
const char* what() const noexcept override {
return "MyBaseException";
}
};
class MyDerivedException : public MyBaseException {
public:
const char* what() const noexcept override {
return "MyDerivedException";
}
};
int main() {
try {
// Throw a derived exception
throw MyDerivedException();
} catch (MyDerivedException& e) {
std::cout << "Caught MyDerivedException: " << e.what() << std::endl;
} catch (MyBaseException& e) {
std::cout << "Caught MyBaseException: " << e.what() << std::endl;
} catch (std::exception& e) {
std::cout << "Caught std::exception: " << e.what() << std::endl;
}
return 0;
}
In this example, we first throw a MyDerivedException
and then catch it. We also catch its base class, MyBaseException
, and the more general std::exception
. The catch blocks are evaluated from top to bottom, so it’s important to catch the most specific exceptions first.
In Java:
In Java, you can catch exceptions by their base and derived types using try-catch blocks in a similar way:
class MyBaseException extends Exception {
MyBaseException() {
super("MyBaseException");
}
}
class MyDerivedException extends MyBaseException {
MyDerivedException() {
super("MyDerivedException");
}
}
public class ExceptionExample {
public static void main(String[] args) {
try {
// Throw a derived exception
throw new MyDerivedException();
} catch (MyDerivedException e) {
System.out.println("Caught MyDerivedException: " + e.getMessage());
} catch (MyBaseException e) {
System.out.println("Caught MyBaseException: " + e.getMessage());
} catch (Exception e) {
System.out.println("Caught Exception: " + e.getMessage());
}
}
}
In this Java example, we use custom exception classes, MyBaseException
and MyDerivedException
, and catch them in a similar fashion to C++. Again, it’s important to catch the exceptions from the most specific to the more general. Java uses inheritance in exception classes, similar to C++.