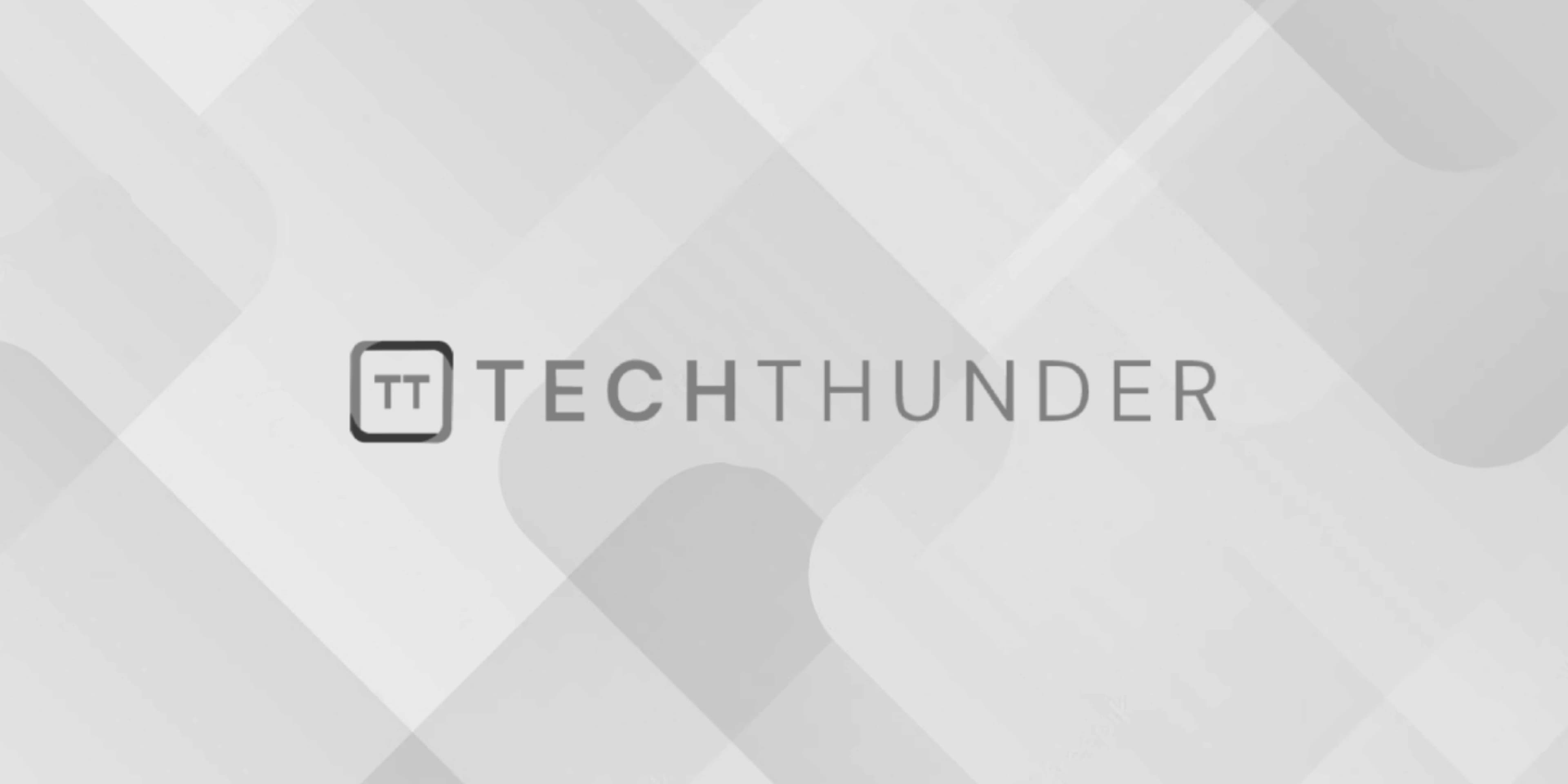
C++ Number in Characters
If you want to convert a number to its equivalent characters (digits) in C++, you can use a few different approaches. Here are two common methods:
Method 1: Using String Conversion
You can convert a number to a string in C++ and then access its individual characters. Here’s how to do it:
#include <iostream>
#include <string>
int main() {
int number = 12345; // Replace with your desired number
// Convert the number to a string
std::string numberStr = std::to_string(number);
// Iterate through the characters and print them
for (char c : numberStr) {
std::cout << c << " ";
}
return 0;
}
In this code, std::to_string()
is used to convert the integer number
into a string. Then, a loop is used to iterate through each character in the string and print it.
Method 2: Using Modulo and Division
You can extract the individual digits of a number by repeatedly using modulo and integer division. Here’s how you can do it:
#include <iostream>
int main() {
int number = 12345; // Replace with your desired number
// Extract and print individual digits
while (number > 0) {
int digit = number % 10; // Get the last digit
std::cout << digit << " ";
number /= 10; // Remove the last digit
}
return 0;
}
In this code, the number % 10
operation extracts the last digit of the number, and number /= 10
removes the last digit. This process is repeated in a loop until the entire number is processed.
Both of these methods will allow you to convert a number into its individual characters (digits) in C++. Choose the one that best fits your needs and programming style.