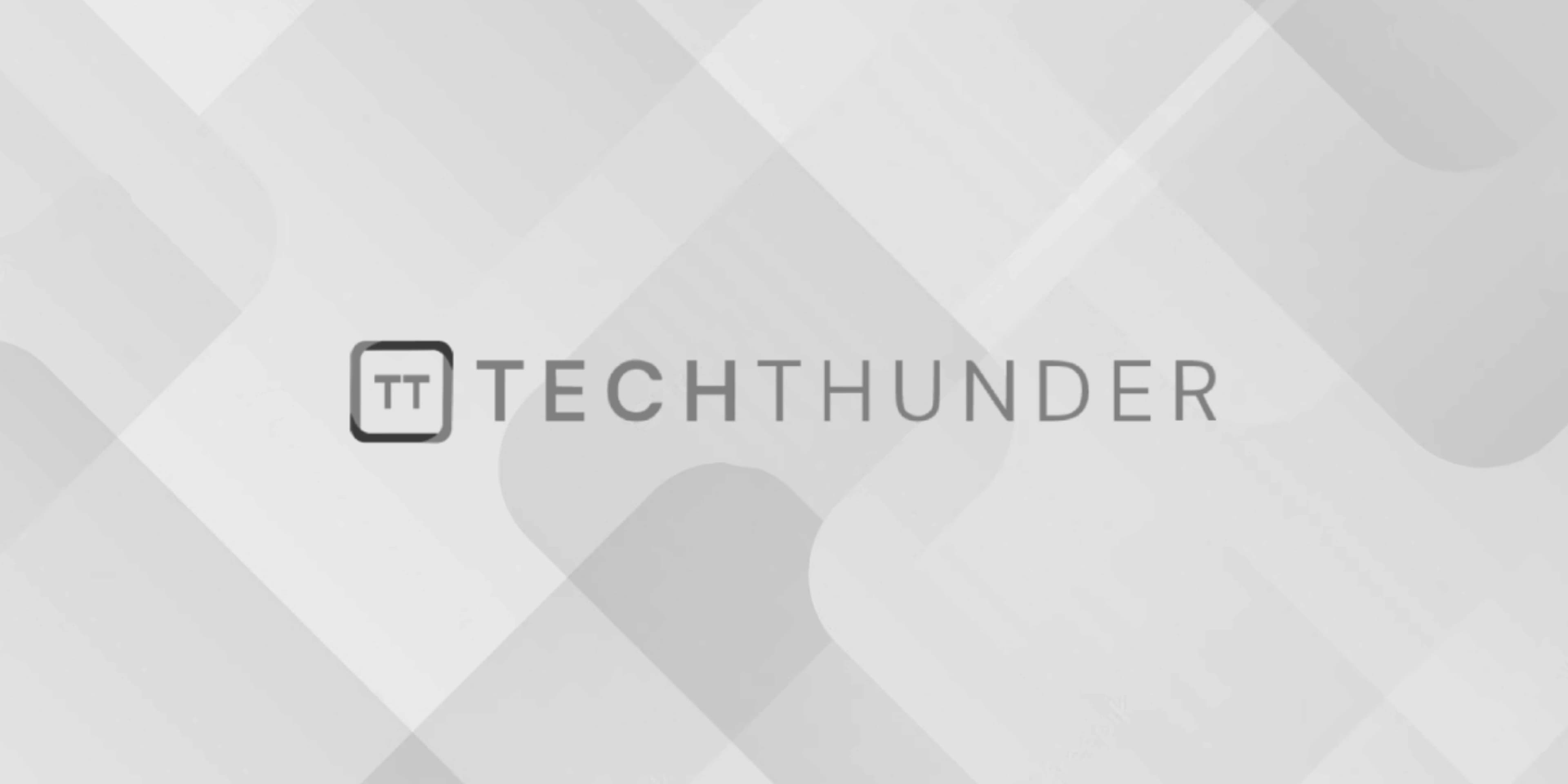
128 views
Stopwatch in C++
Creating a simple stopwatch in C++ involves measuring time intervals using the <chrono>
library, which provides precise timekeeping capabilities. Here’s a basic example of how to create a stopwatch in C++:
C++
#include <iostream>
#include <chrono>
#include <thread>
class Stopwatch {
public:
void Start() {
startTime = std::chrono::high_resolution_clock::now();
isRunning = true;
}
void Stop() {
endTime = std::chrono::high_resolution_clock::now();
isRunning = false;
}
void Reset() {
startTime = endTime = std::chrono::high_resolution_clock::now();
isRunning = false;
}
double ElapsedSeconds() {
if (isRunning) {
Stop();
}
std::chrono::duration<double> elapsed = endTime - startTime;
return elapsed.count();
}
private:
std::chrono::time_point<std::chrono::high_resolution_clock> startTime;
std::chrono::time_point<std::chrono::high_resolution_clock> endTime;
bool isRunning = false;
};
int main() {
Stopwatch stopwatch;
std::cout << "Press Enter to start the stopwatch..." << std::endl;
std::cin.get();
stopwatch.Start();
std::this_thread::sleep_for(std::chrono::seconds(3)); // Simulate some work
stopwatch.Stop();
std::cout << "Elapsed time: " << stopwatch.ElapsedSeconds() << " seconds" << std::endl;
return 0;
}
In this example:
- We create a
Stopwatch
class with methods to start, stop, reset, and calculate the elapsed time in seconds. - The
Start()
method records the start time, theStop()
method records the end time, and theReset()
method resets the stopwatch to its initial state. - The
ElapsedSeconds()
method calculates the elapsed time in seconds based on the start and end times. - In the
main
function, we create aStopwatch
object, start it, simulate some work usingstd::this_thread::sleep_for()
, stop it, and then display the elapsed time.
This is a basic example of a stopwatch. You can enhance it by adding features like lap times or a user-friendly interface for starting, stopping, and resetting the stopwatch.