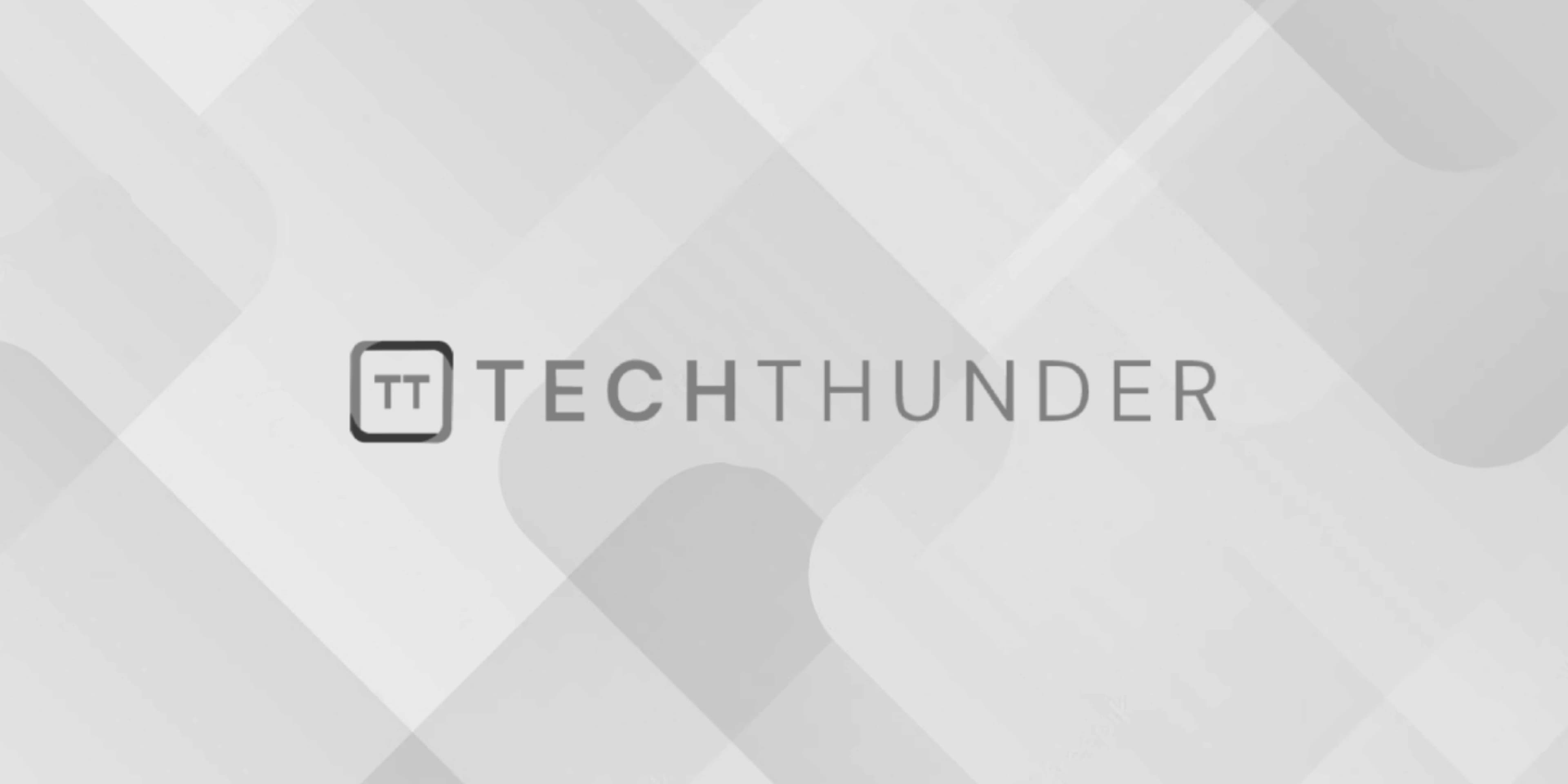
102 views
Check if bits in Range L to R of Two Numbers are Complement of Each other or Not in C++
To check if the bits in a given range [L, R] of two numbers are complements of each other in C++, you can use bitwise operations. The XOR (^) operator is particularly useful for this task because XORing two bits results in 1 if the bits are different (complements) and 0 if they are the same.
Here’s a C++ function to check if the bits in the specified range [L, R] of two numbers are complements:
C++
#include <iostream>
bool areBitsComplements(int num1, int num2, int L, int R) {
// Create a mask with 1s in the range [L, R]
int mask = ((1 << (R - L + 1)) - 1) << L;
// Extract the bits in the specified range from both numbers
int bits1 = (num1 & mask) >> L;
int bits2 = (num2 & mask) >> L;
// Check if the bits are complements by XORing them
return (bits1 ^ bits2) == ((1 << (R - L + 1)) - 1);
}
int main() {
int num1 = 12; // Binary: 1100
int num2 = 3; // Binary: 0011
int L = 0;
int R = 3;
bool result = areBitsComplements(num1, num2, L, R);
if (result) {
std::cout << "Bits in range [" << L << ", " << R << "] are complements." << std::endl;
} else {
std::cout << "Bits in range [" << L << ", " << R << "] are not complements." << std::endl;
}
return 0;
}
In this example:
- The
areBitsComplements
function takes two numbers,num1
andnum2
, as well as the range [L, R] to check. - It creates a mask with 1s in the specified range [L, R] using bitwise operations.
- It extracts the bits in the specified range from both
num1
andnum2
using bitwise AND and shifting. - It XORs the extracted bits and checks if the result is a sequence of 1s, which would indicate that the bits in the range are complements.
- Finally, the
main
function demonstrates how to use this function to check if the bits in the range [L, R] ofnum1
andnum2
are complements.
Keep in mind that this code assumes that the range [L, R] is valid and within the bit-width of the integers being checked.