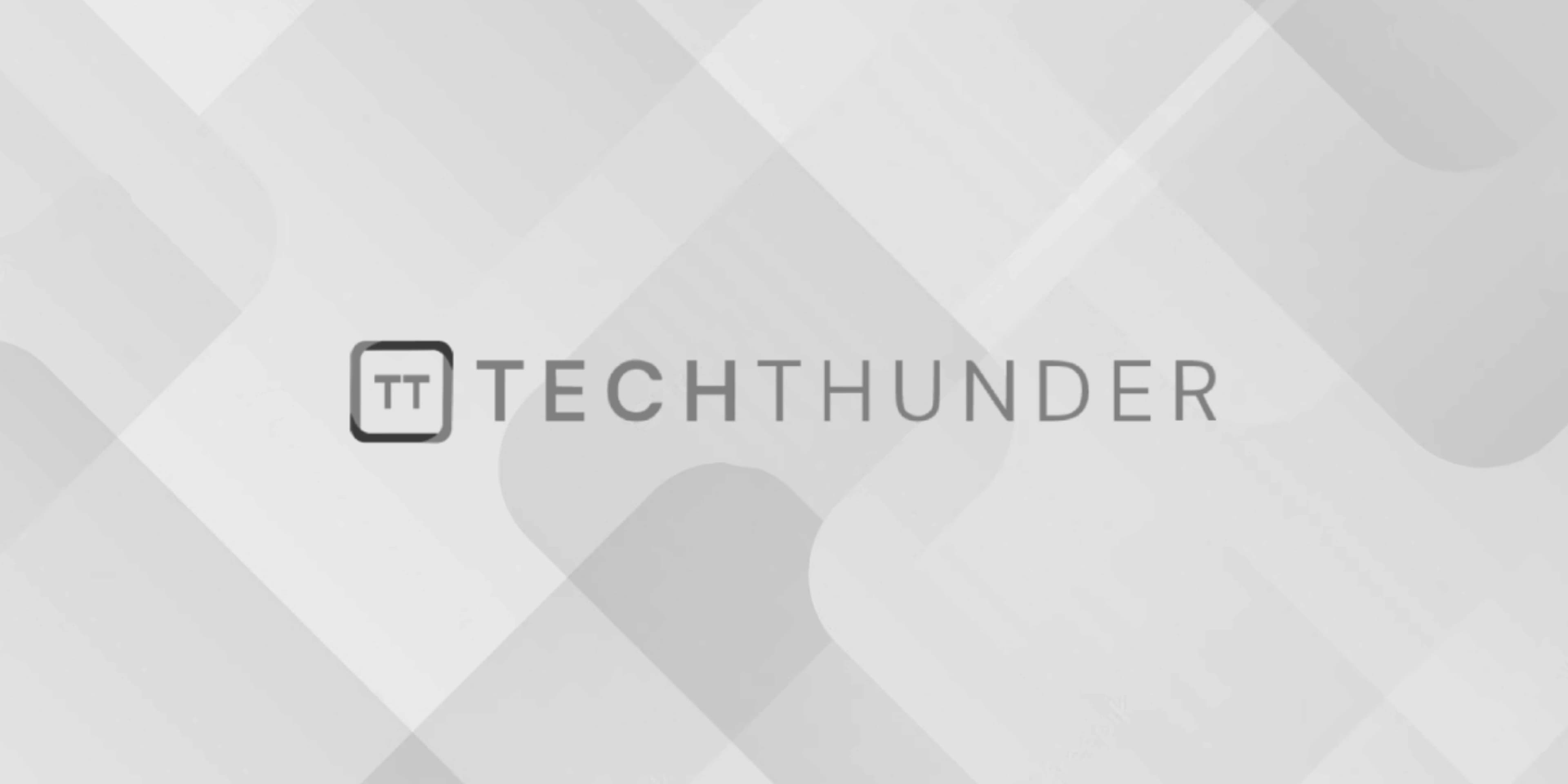
153 views
C++ User-Defined
The C++ can define your own user-defined types using structures (structs) and classes. These types allow you to encapsulate data and functions into a single unit, providing a way to model real-world objects or abstract concepts. Here, I’ll explain how to define your own user-defined types using both structs and classes.
- Using Structs: A struct is a simple way to define a user-defined type with public data members. Here’s an example:
C++
// Define a struct representing a Point in 2D space
struct Point {
double x; // public data member
double y; // public data member
};
You can then create instances of this struct:
C++
Point p1;
p1.x = 1.0;
p1.y = 2.0;
- Using Classes: A class is similar to a struct but allows you to encapsulate data and functions (methods) together with access control (public, private, and protected). Here’s an example:
C++
// Define a class representing a Rectangle
class Rectangle {
public:
// Constructors
Rectangle(); // Default constructor
Rectangle(double width, double height); // Parameterized constructor
// Member functions
double area() const;
double perimeter() const;
private:
double width; // private data member
double height; // private data member
};
// Define the constructor implementations
Rectangle::Rectangle() : width(0.0), height(0.0) {
}
Rectangle::Rectangle(double w, double h) : width(w), height(h) {
}
// Define member function implementations
double Rectangle::area() const {
return width * height;
}
double Rectangle::perimeter() const {
return 2 * (width + height);
}
You can create instances of the class and use its member functions:
C++
Rectangle r1; // Create a default rectangle (0x0)
Rectangle r2(3.0, 4.0); // Create a rectangle with width 3.0 and height 4.0
double r1_area = r1.area(); // Calculate the area of r1
double r2_perimeter = r2.perimeter(); // Calculate the perimeter of r2
User-defined types allow you to create complex data structures and objects that can represent various entities and behaviors in your C++ programs. You can also implement constructors, destructors, and operator overloads to customize the behavior of your user-defined types as needed.