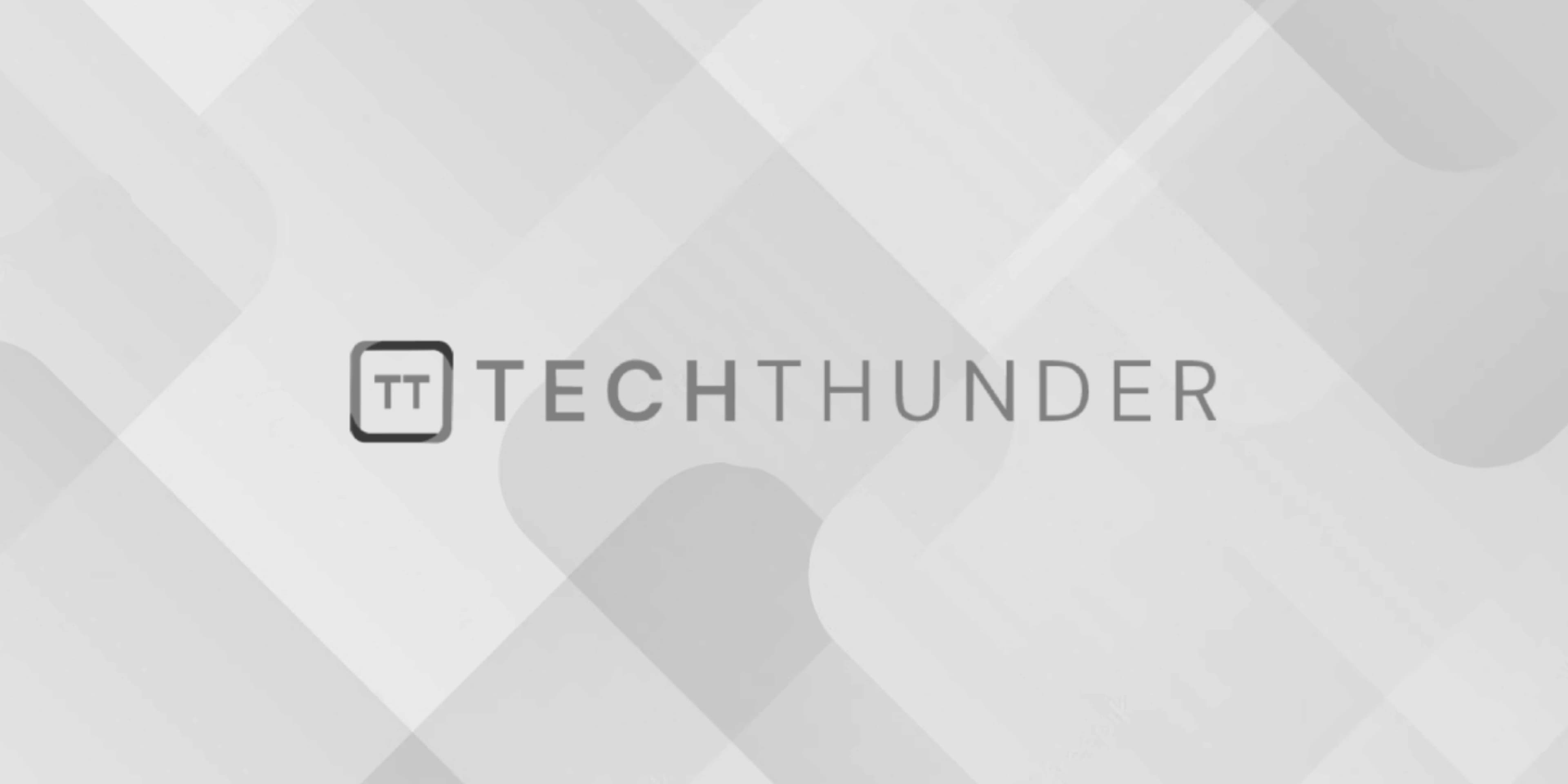
C++ Memory Management
Memory management in C++ involves the allocation and deallocation of memory for objects and data structures. There are several ways to manage memory in C++, including automatic, dynamic, and manual memory management.
1. Automatic Memory Management:
Automatic memory management, also known as stack allocation, is handled by the compiler. It involves allocating memory for local variables within a function and automatically releasing it when the function exits.
Example:
void someFunction() {
int localVar = 10; // Memory for localVar is allocated on the stack
// ...
} // Memory for localVar is automatically deallocated when someFunction() exits
2. Static Memory Allocation:
Static memory allocation involves allocating memory at compile time. This is commonly used for arrays and other data structures whose size is known at compile time.
Example:
int staticArray[10]; // Memory for staticArray is allocated at compile time
3. Dynamic Memory Allocation:
Dynamic memory allocation allows you to allocate memory at runtime using operators new
and delete
.
new
Operator:
int* ptr = new int; // Allocates memory for an integer on the heap
// ...
delete ptr; // Deallocates the memory when no longer needed
delete
Operator:
int* ptr = new int; // Allocate memory
// ...
delete ptr; // Deallocate memory
new[]
and delete[]
for Arrays:
int* dynamicArray = new int[10]; // Allocate an array of 10 integers on the heap
// ...
delete[] dynamicArray; // Deallocate the array when no longer needed
Advantages and Disadvantages of Dynamic Allocation:
Advantages:
- Allows you to allocate memory of a desired size at runtime.
- Helps in avoiding memory wastage.
- Useful when the size of data structures is unknown at compile time.
Disadvantages:
- Requires manual deallocation (using
delete
ordelete[]
). - Improper use can lead to memory leaks or undefined behavior.
- Can be slower than stack allocation due to the overhead of dynamic memory management.
4. Smart Pointers:
Smart pointers are objects that behave like pointers but also provide automatic memory management. They automatically call delete
when the pointer is no longer needed, avoiding memory leaks.
Example:
std::unique_ptr<int> smartPtr = std::make_unique<int>(42);
// Memory is automatically deallocated when smartPtr goes out of scope
5. RAII (Resource Acquisition Is Initialization):
RAII is a programming technique that binds the life cycle of a resource (like memory) to the scope of an object. When the object goes out of scope, the resource is automatically released.
Example using a custom class:
class Resource {
public:
Resource() {
// Acquire resource (e.g., allocate memory)
}
~Resource() {
// Release resource (e.g., deallocate memory)
}
};
void someFunction() {
Resource res; // Resource is automatically acquired
// ...
} // Resource is automatically released when someFunction() exits
6. Garbage Collection:
C++ does not have a built-in garbage collector like some other languages (e.g., Java, C#). However, there are libraries and tools available for garbage collection in C++, such as the Boehm-Demers-Weiser garbage collector.
Remember that good memory management practices are crucial for writing efficient, reliable, and bug-free code. Always ensure that you deallocate memory properly to avoid memory leaks. Additionally, prefer automatic memory management techniques like RAII and smart pointers to manual dynamic memory management whenever possible.