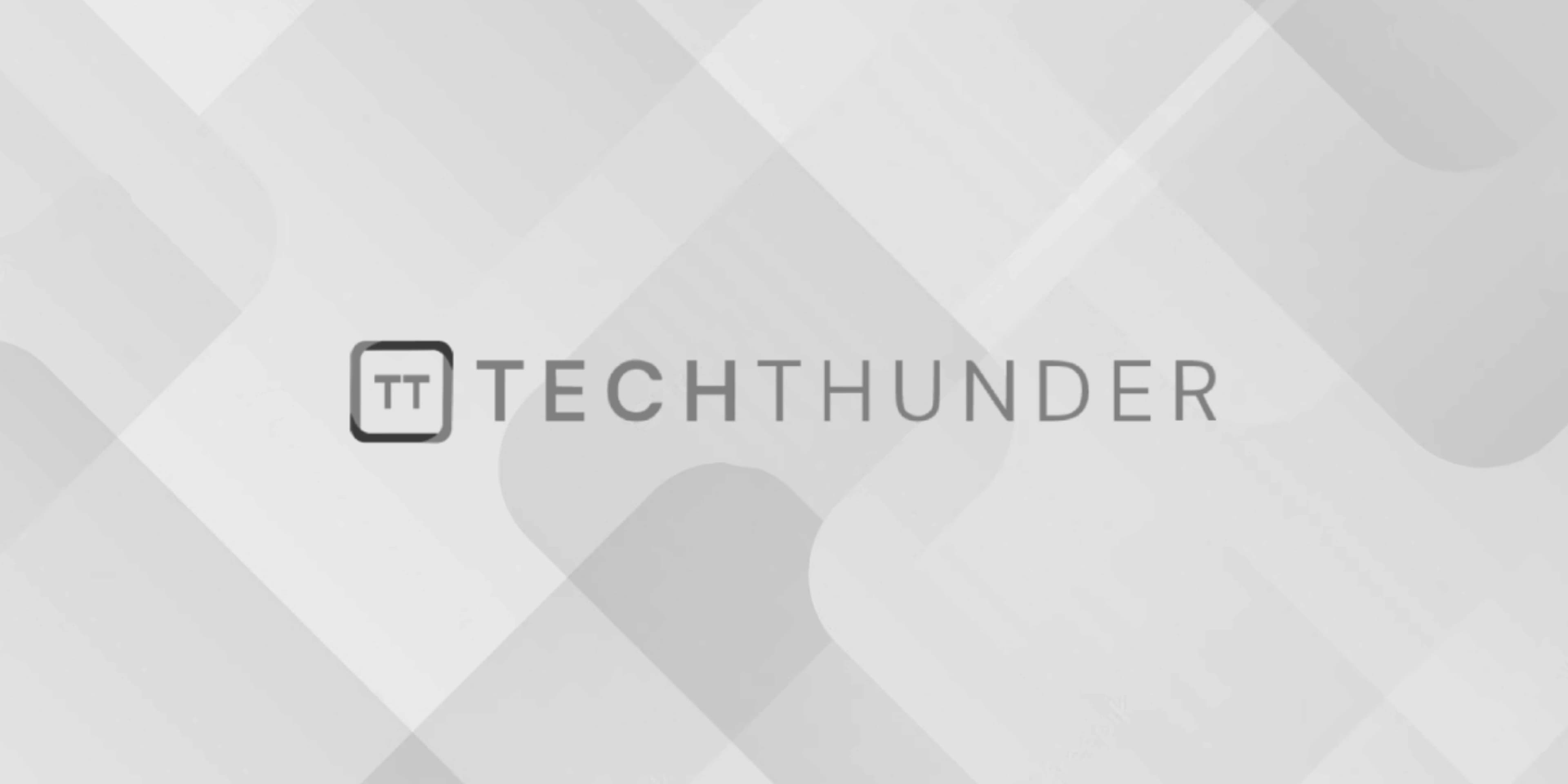
387 views
Reverse an Array in C++
To reverse an array in C++, you can use a simple loop to swap the elements from the beginning and end of the array, gradually moving towards the center. Here’s a C++ code example that demonstrates how to reverse an array:
C++
#include <iostream>
void reverseArray(int arr[], int size) {
int start = 0;
int end = size - 1;
while (start < end) {
// Swap the elements at the start and end positions
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
// Move the start and end indices towards the center
start++;
end--;
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
std::cout << "Original Array: ";
for (int i = 0; i < size; i++) {
std::cout << arr[i] << " ";
}
reverseArray(arr, size);
std::cout << "\nReversed Array: ";
for (int i = 0; i < size; i++) {
std::cout << arr[i] << " ";
}
return 0;
}
In this code, the reverseArray
function reverses the elements in the array by swapping the first element with the last, the second element with the second-to-last, and so on until it reaches the middle of the array.
When you run the code, it will first print the original array and then the reversed array.