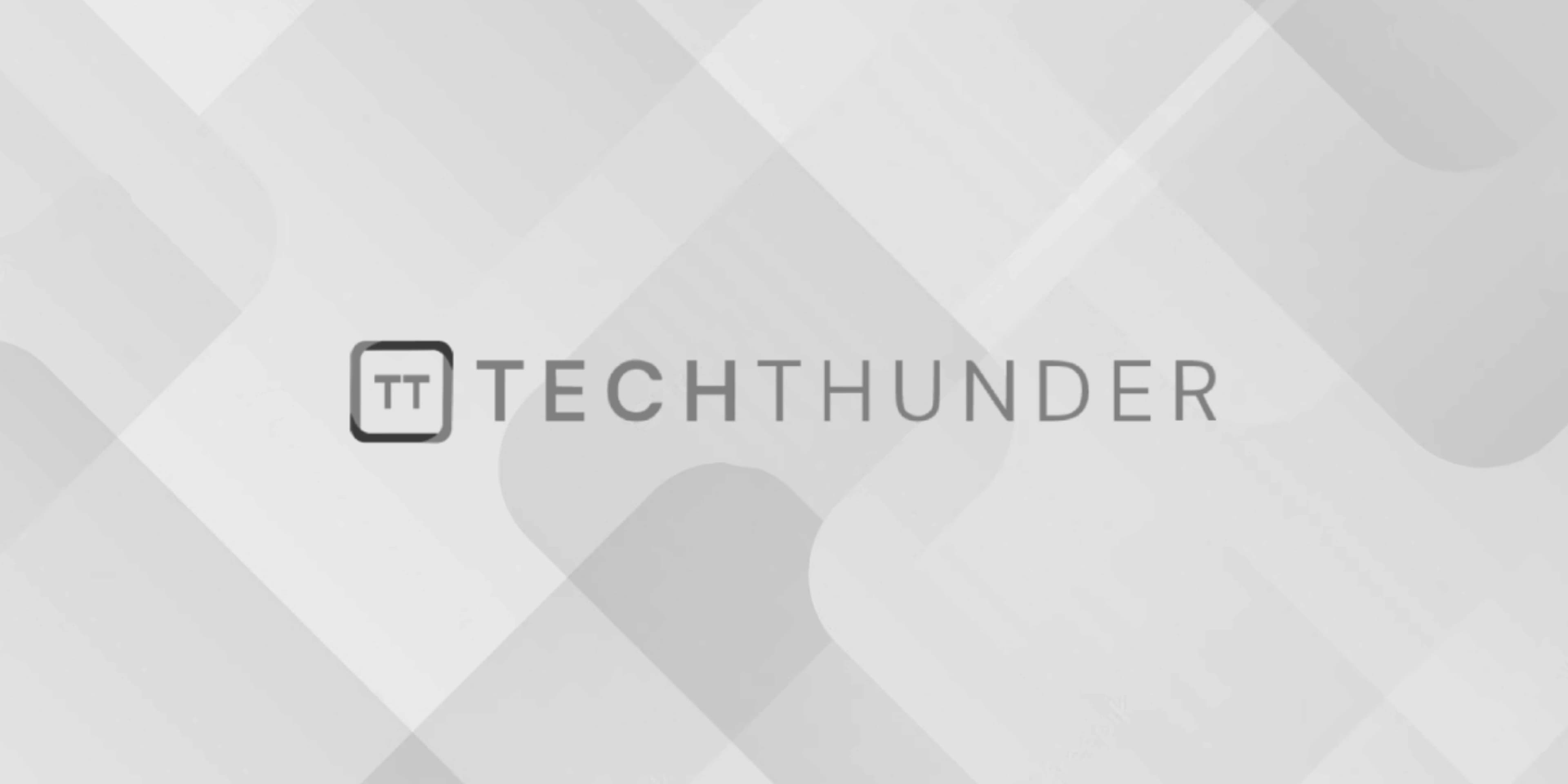
367 views
C++ Flow Control
Flow control in C++ refers to the mechanisms and statements that allow you to control the order in which instructions are executed in a program. It enables you to make decisions, loop through code, and control the flow of your program based on certain conditions. There are several key flow control structures in C++:
- Conditional Statements:
if
statement: Allows you to execute a block of code if a certain condition is true.else
statement: Used in conjunction withif
to specify an alternative block of code to execute if the condition is false.else if
statement: Used to check additional conditions after the initialif
condition.switch
statement: Used to select one of many code blocks to execute based on the value of an expression.
Example of an if
statement:
C++
int x = 10;
if (x > 5) {
// This code block will execute because x is greater than 5.
cout << "x is greater than 5." << endl;
} else {
// This block will execute if the condition is false.
cout << "x is not greater than 5." << endl;
}
- Looping Statements:
for
loop: Repeats a block of code a specified number of times.while
loop: Repeats a block of code as long as a specified condition is true.do-while
loop: Repeats a block of code at least once, then continues as long as a specified condition is true.
Example of a for
loop:
C++
for (int i = 0; i < 5; i++) {
// This code block will execute 5 times.
cout << "Iteration " << i << endl;
}
Example of a while
loop:
C++
int n = 0;
while (n < 5) {
// This code block will execute as long as n is less than 5.
cout << "n is " << n << endl;
n++;
}
- Control Statements:
break
statement: Used to exit from a loop or aswitch
statement prematurely.continue
statement: Skips the current iteration of a loop and proceeds to the next iteration.return
statement: Exits a function and returns a value.
Example of a break
statement:
C++
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exit the loop when i is 5.
}
cout << "i is " << i << endl;
}
These flow control structures allow you to create more dynamic and responsive C++ programs by controlling the flow of execution based on conditions, loops, and decision-making.