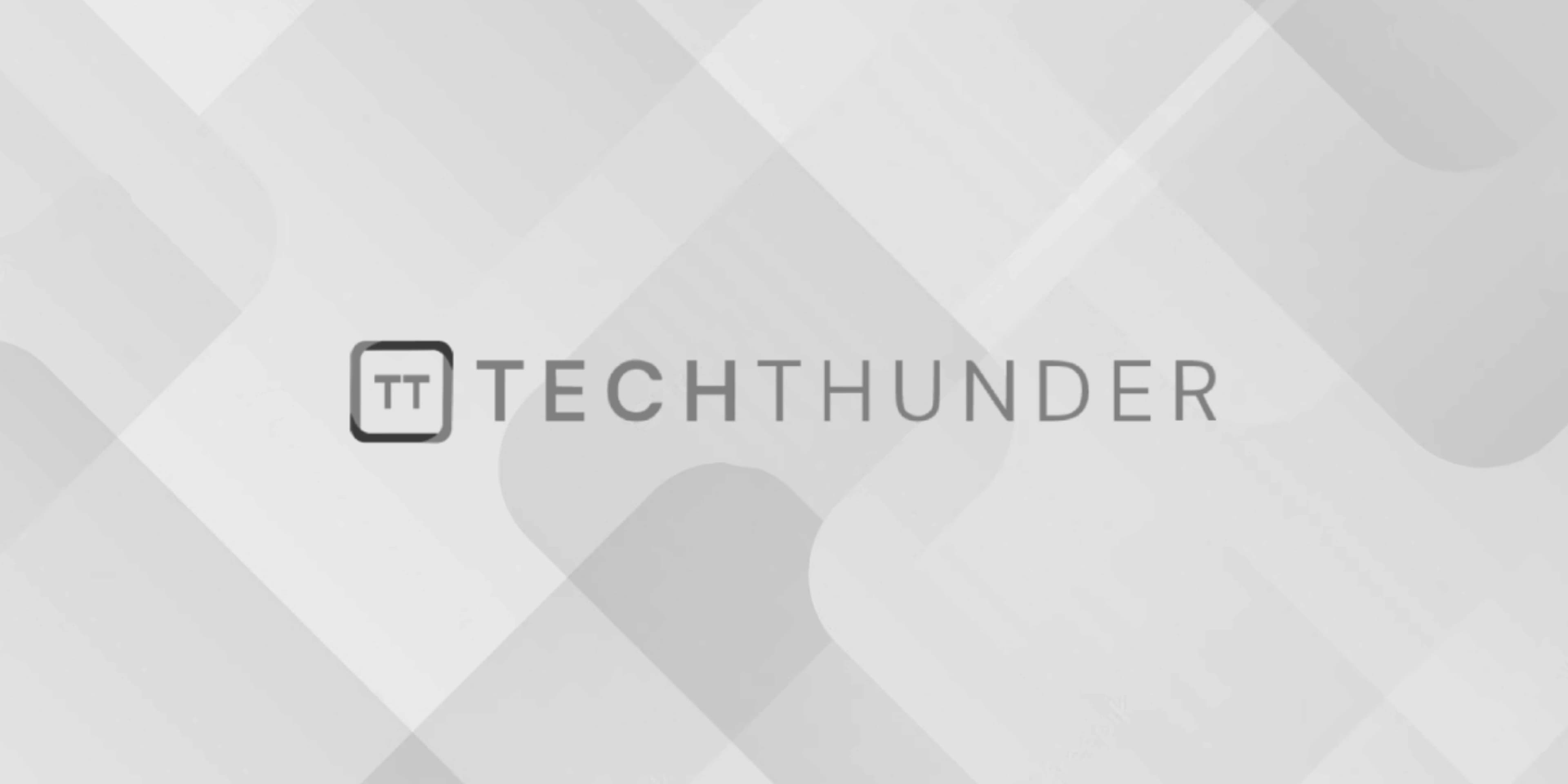
249 views
Operator Overloading in C++
Operator overloading in C++ allows you to define custom behaviors for operators when used with user-defined types (classes and structures). This feature enables you to make your user-defined types work with operators in a way that is meaningful for your application.
Here’s a brief overview of how operator overloading works in C++:
- Defining Operator Overloads: To overload an operator, you need to define a function for that operator with a special syntax. The function name is the operator itself, and it takes one or more arguments, just like any other function. For binary operators (e.g.,
+
,-
,*
), you typically define a binary operator member function that takes one argument of the same type as the class and returns a new instance of the class.
C++
class MyType {
public:
MyType operator+(const MyType& other) const {
// Define how the + operator works for your class
MyType result;
// Perform addition logic here
return result;
}
};
- Usage: After overloading an operator, you can use it just like any other built-in operator. The compiler will automatically call your overloaded function when the operator is used with instances of your class.
C++
MyType a, b, c;
c = a + b; // Calls your overloaded operator+
- Unary Operators: Unary operators (e.g.,
++
,--
,-
) can also be overloaded. These operators usually take no arguments for a member function.
C++
class MyType {
public:
MyType operator++() {
// Define how the ++ operator (pre-increment) works for your class
// Modify the current object
return *this;
}
};
- Global vs. Member Functions: You can overload operators as member functions of your class or as global functions. Global function overloads often make sense when you need to overload operators for cases where the left-hand operand is not your user-defined type.
- Rules and Restrictions: Operator overloads should follow some conventions. For example, they should return the result by value, by reference, or by pointer, depending on the desired behavior. There are also some operators that cannot be overloaded (
.
,::
,?:
,sizeof
,typeid
, etc.).
Here’s a more complete example of operator overloading for a custom complex number class:
C++
class Complex {
public:
double real;
double imag;
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
// Other operator overloads like ==, !=, etc.
};
With this Complex
class, you can perform addition, subtraction, and multiplication of complex numbers using the +
, -
, and *
operators.