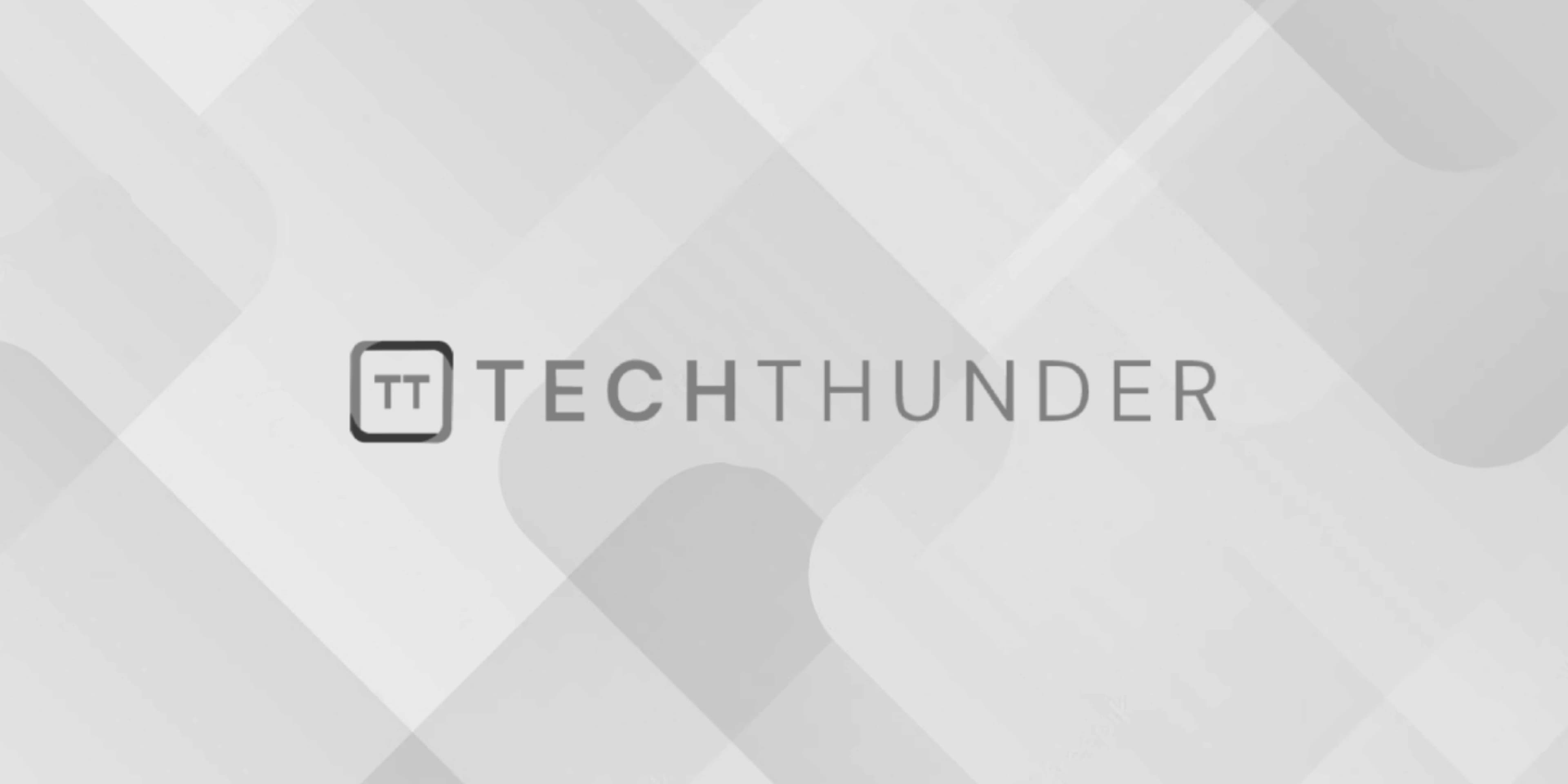
Virtual function vs Pure virtual function in C++
The virtual functions and pure virtual functions are key features of object-oriented programming that enable polymorphism and dynamic method dispatch. However, they serve different purposes and have distinct characteristics:
- Virtual Function:
- A virtual function is a member function declared in a base class with the
virtual
keyword. - Virtual functions can have a default implementation in the base class, and derived classes have the option to override them.
- When a virtual function is called through a pointer or reference to a base class, the actual function to be executed is determined at runtime based on the object’s dynamic type (the type of the object the pointer or reference points to).
- If a derived class does not provide an implementation for a virtual function declared in the base class, the base class’s implementation (if present) is used as the default. Example:
class Base {
public:
virtual void show() {
std::cout << "Base class\n";
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class\n";
}
};
In this example, show
is a virtual function, and Derived
provides its own implementation. When calling show
through a base class pointer pointing to a Derived
object, the Derived
class’s implementation is executed.
- Pure Virtual Function:
- A pure virtual function is a virtual function declared in a base class with the
virtual
keyword and the= 0
specifier. It has no implementation in the base class. - A class containing one or more pure virtual functions is called an abstract base class, and it cannot be instantiated directly.
- Derived classes of an abstract base class must provide implementations for all pure virtual functions declared in the base class. Otherwise, they remain abstract classes. Example:
class AbstractBase {
public:
virtual void pureVirtualFunction() = 0;
};
class Derived : public AbstractBase {
public:
void pureVirtualFunction() override {
std::cout << "Derived class implements the pure virtual function\n";
}
};
In this example, AbstractBase
is an abstract base class with a pure virtual function. Derived
provides an implementation for the pure virtual function, making it a concrete class.
In summary, the main difference between virtual functions and pure virtual functions is that virtual functions can have default implementations in the base class and can be optionally overridden in derived classes, while pure virtual functions have no implementation in the base class and must be implemented in derived classes to create concrete objects. Both types of functions enable polymorphism and dynamic dispatch in C++ by allowing the selection of the appropriate method to execute based on the object’s actual type at runtime.