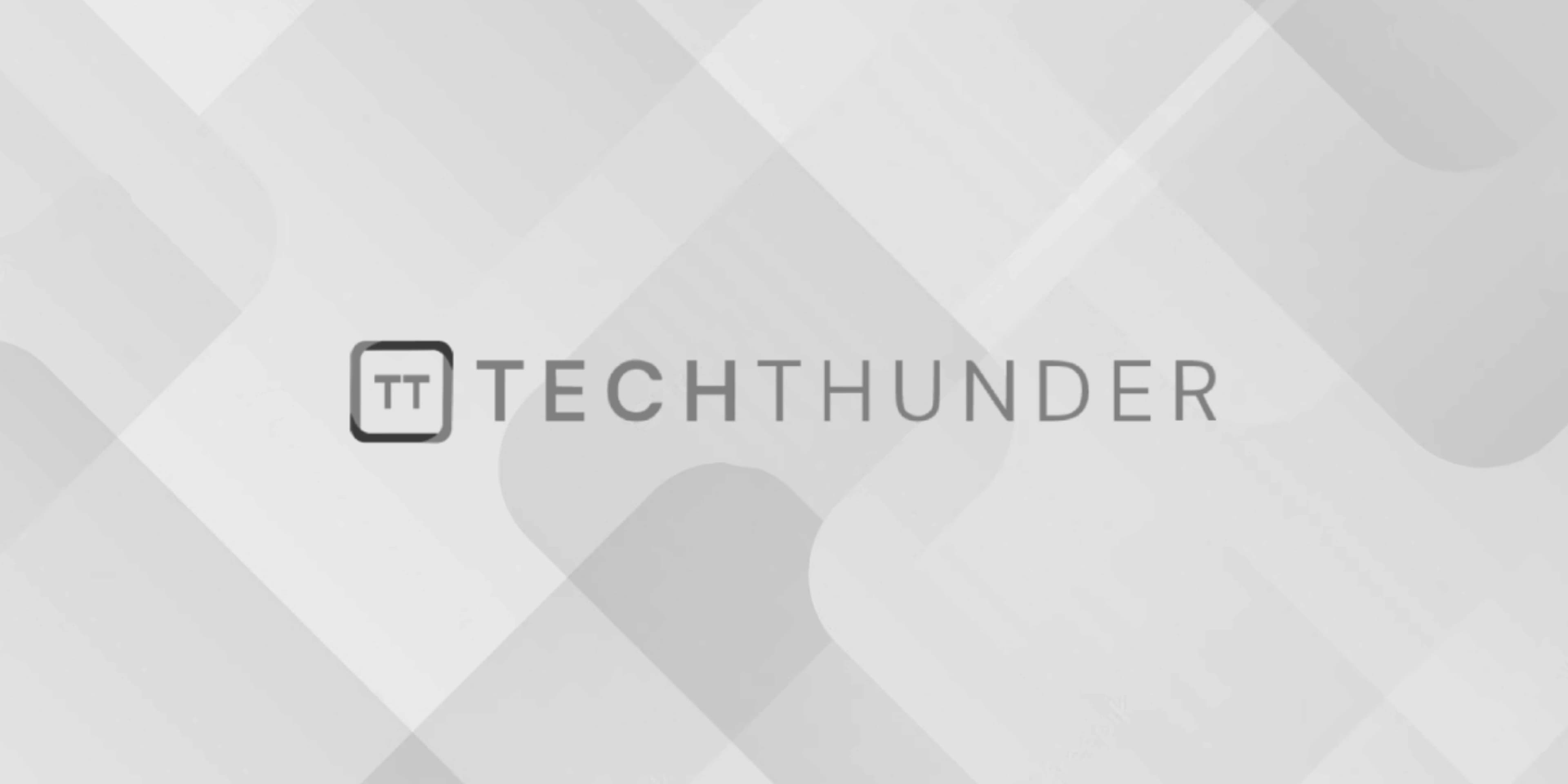
Hierarchical inheritance in C++
Hierarchical inheritance is one of the types of inheritance in C++ and is a fundamental concept in object-oriented programming (OOP). In hierarchical inheritance, one base class is inherited by multiple derived classes. Each of these derived classes can have its own additional members and methods in addition to inheriting the common members and methods from the base class.
Here’s how hierarchical inheritance works in C++:
- Base Class: Start by defining a base class that contains common attributes and behaviors that you want to share among multiple derived classes.
class Animal {
public:
void eat() {
std::cout << "Animal is eating." << std::endl;
}
};
- Derived Classes: Create one or more derived classes that inherit from the base class. Each derived class can have its own unique attributes and behaviors in addition to what it inherits from the base class.
class Dog : public Animal {
public:
void bark() {
std::cout << "Dog is barking." << std::endl;
}
};
class Cat : public Animal {
public:
void meow() {
std::cout << "Cat is meowing." << std::endl;
}
};
- Using the Classes: You can now create objects of the derived classes and access their inherited and unique members.
int main() {
Dog dog;
Cat cat;
dog.eat(); // Inherited from Animal
dog.bark(); // Unique to Dog
cat.eat(); // Inherited from Animal
cat.meow(); // Unique to Cat
return 0;
}
In this example, Animal
is the base class, and Dog
and Cat
are two different derived classes that inherit from Animal
. Each derived class has its own unique method (bark
for Dog
and meow
for Cat
) while also inheriting the eat
method from the base class.
Hierarchical inheritance allows you to create a class hierarchy with a single base class and multiple derived classes, each adding its own features. This promotes code reusability and organization by structuring classes in a logical and hierarchical manner. However, be mindful of potential code duplication and the potential for deep inheritance hierarchies, which can make the code harder to maintain.