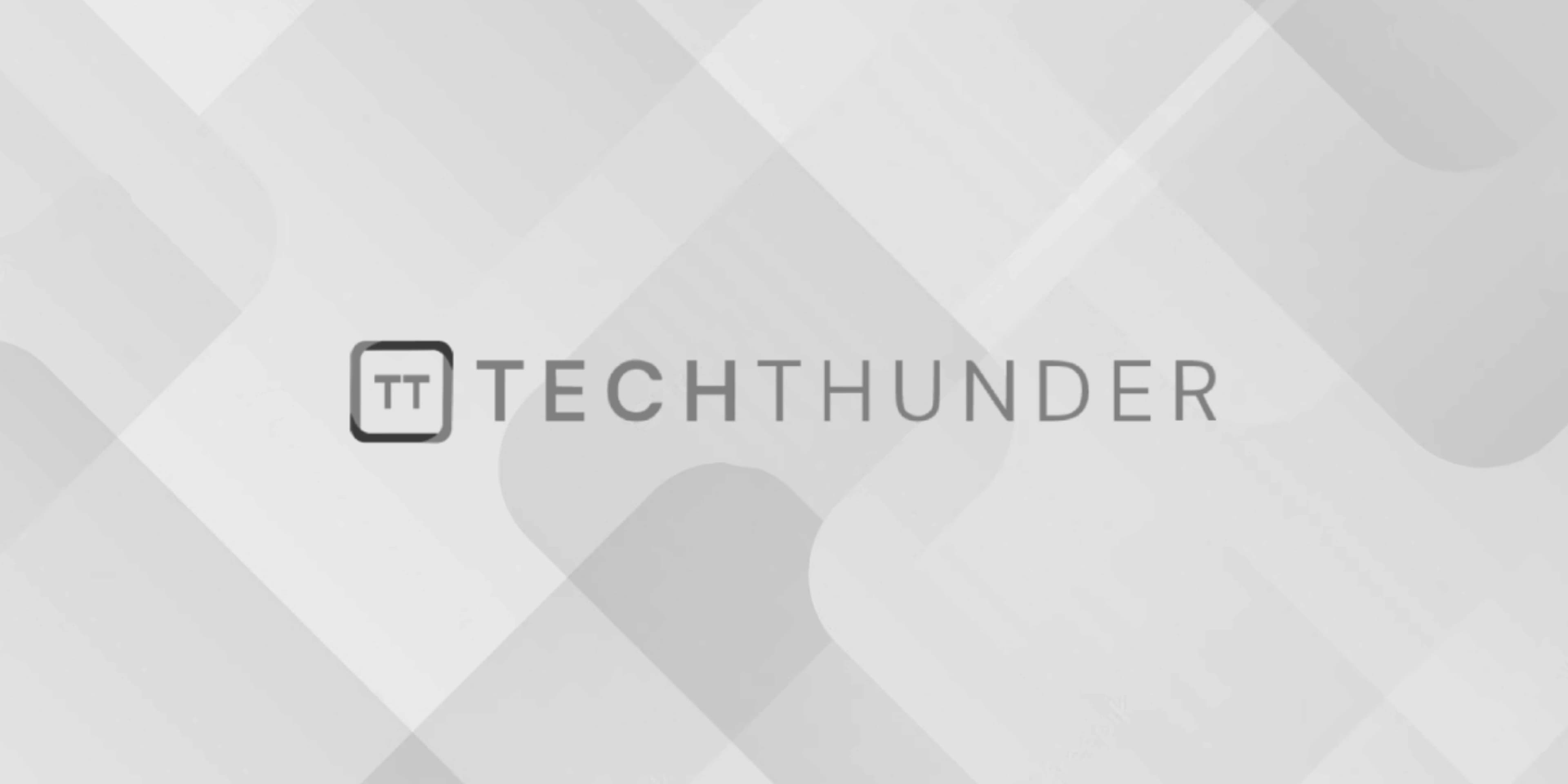
395 views
Adding Vectors in C++
The C++ add two vectors by simply concatenating them using the +
operator or by using the insert
method or push_back
method from the C++ Standard Library. Here are a few ways to add two vectors:
Method 1: Using the +
Operator
C++
#include <iostream>
#include <vector>
int main() {
std::vector<int> vector1 = {1, 2, 3};
std::vector<int> vector2 = {4, 5, 6};
// Concatenate vector2 to vector1 using the + operator
std::vector<int> result = vector1 + vector2;
// Print the result
for (int value : result) {
std::cout << value << " ";
}
std::cout << std::endl;
return 0;
}
Method 2: Using the insert
Method
C++
#include <iostream>
#include <vector>
int main() {
std::vector<int> vector1 = {1, 2, 3};
std::vector<int> vector2 = {4, 5, 6};
// Insert the elements of vector2 into vector1
vector1.insert(vector1.end(), vector2.begin(), vector2.end());
// Print the result
for (int value : vector1) {
std::cout << value << " ";
}
std::cout << std::endl;
return 0;
}
Method 3: Using the push_back
Method
C++
#include <iostream>
#include <vector>
int main() {
std::vector<int> vector1 = {1, 2, 3};
std::vector<int> vector2 = {4, 5, 6};
// Append the elements of vector2 to vector1 using push_back
for (int value : vector2) {
vector1.push_back(value);
}
// Print the result
for (int value : vector1) {
std::cout << value << " ";
}
std::cout << std::endl;
return 0;
}
All of these methods achieve the same result, which is adding the elements of vector2
to vector1
. You can choose the method that you find most convenient for your specific use case.