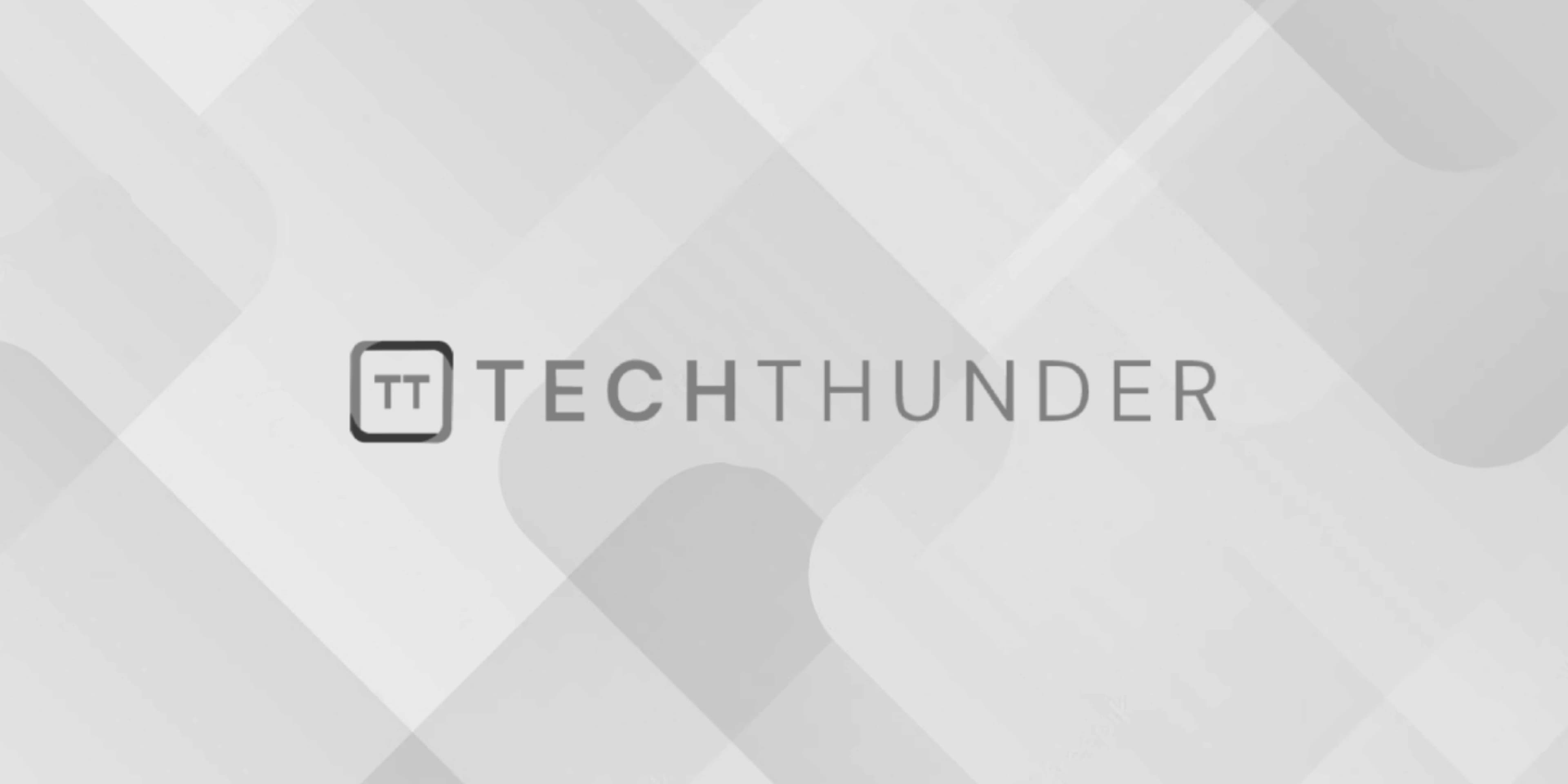
Function Prototype in C++
The C++ function prototype is a declaration of a function that specifies its name, return type, and parameter types, but not its actual implementation. Function prototypes are used to inform the compiler about the existence and interface of a function before it is called within the program. This is especially important when functions are defined in separate source files or when the order of function definitions matters.
A function prototype typically includes the following elements:
- Return Type: The data type that specifies what the function will return.
- Function Name: The unique name that identifies the function within the program.
- Parameter List: The list of input parameters along with their data types that the function expects.
Here’s the general syntax of a function prototype:
return_type function_name(parameter1_type parameter1_name, parameter2_type parameter2_name, ...);
For example, consider a simple function that calculates the sum of two integers:
// Function prototype
int add(int a, int b);
int main() {
int result = add(3, 5); // Function call
return 0;
}
// Function definition
int add(int a, int b) {
return a + b;
}
In this example:
- The function prototype
int add(int a, int b);
is declared before themain
function, which informs the compiler about theadd
function’s existence, return type (int
), and parameter types (int
andint
). - The actual implementation of the
add
function appears after themain
function.
Function prototypes are essential in C++ for several reasons:
- They allow functions to be defined in any order within a program.
- They enable the compiler to perform type checking and ensure that function calls are made with the correct number and types of arguments.
- They help catch errors and prevent unexpected behavior, improving code robustness.
- They serve as documentation, providing information about a function’s interface to other programmers.
In practice, it’s a good practice to include function prototypes for functions declared in header files to ensure consistent function signatures across multiple source files in a project. This practice is particularly important when working on larger projects with multiple source files.