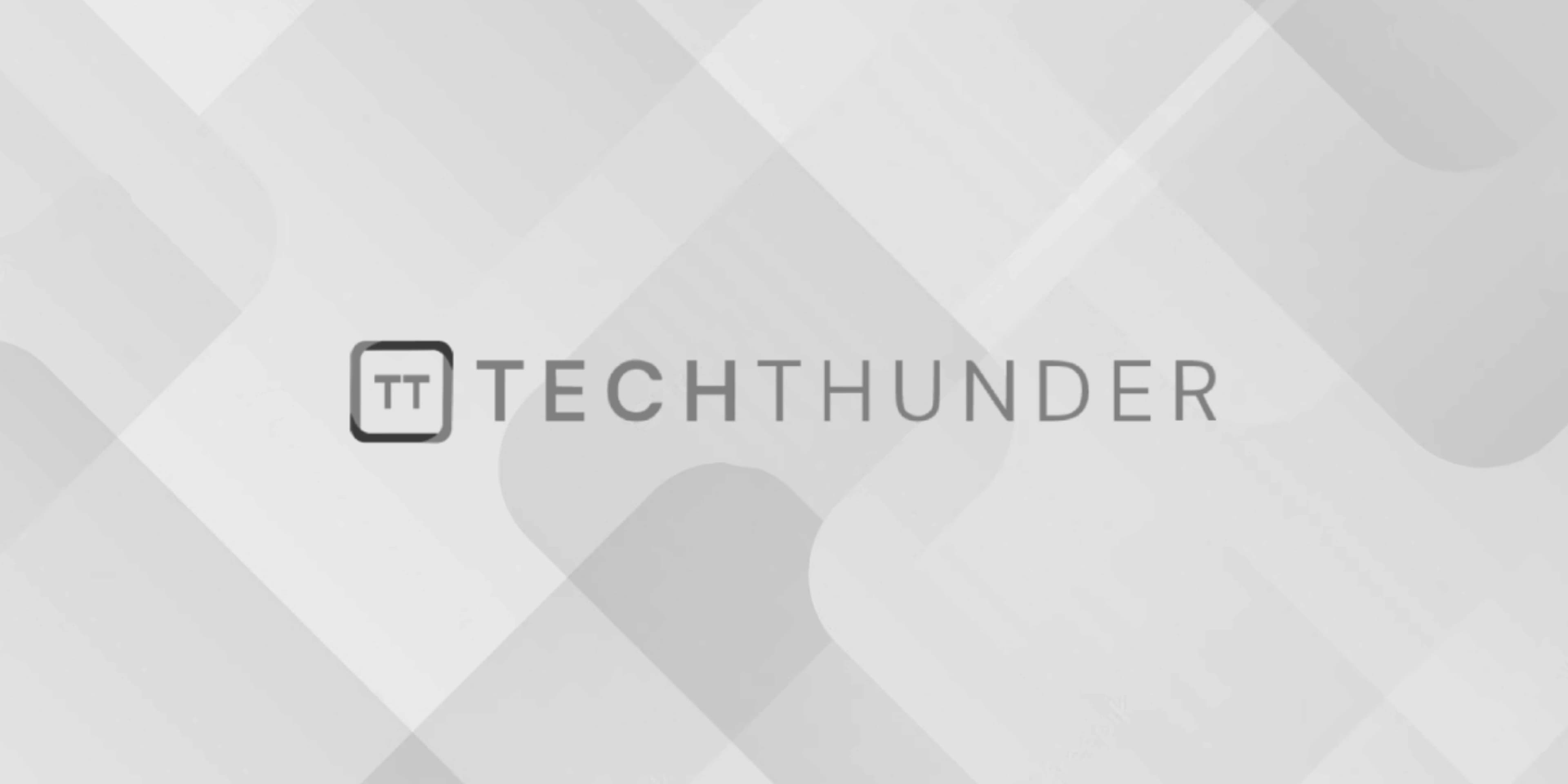
Default arguments in C++
Default arguments in C++ allow you to specify default values for function parameters. When a function is called, if the caller does not provide a value for a parameter with a default argument, the default value specified in the function declaration will be used. Default arguments provide flexibility by allowing you to call a function with fewer arguments than its definition.
Here’s how you can define and use default arguments in C++:
#include <iostream>
// Function with default arguments
void printMessage(const std::string& message = "Hello, World!") {
std::cout << message << std::endl;
}
int main() {
// Calling the function with and without providing an argument
printMessage(); // Uses the default argument
printMessage("Custom message"); // Uses the provided argument
return 0;
}
In the example above, the printMessage
function has a default argument of "Hello, World!"
for its message
parameter. When you call printMessage()
without providing an argument, the default value is used. When you call printMessage("Custom message")
, the provided argument is used instead.
Here are some key points to remember about default arguments:
- Default arguments are specified in the function declaration, not the function definition. They must be placed in the function prototype.
- Default arguments should be placed from right to left in the parameter list. You cannot have default arguments for parameters to the left of non-defaulted parameters. For example, this is not allowed:
// Invalid: Default argument before non-defaulted parameter
void invalidFunction(int a = 0, int b);
- Once you provide a default argument for a parameter, all the parameters to its right should also have default arguments. For example, this is not allowed:
// Invalid: Non-defaulted parameter after default argument
void invalidFunction(int a = 0, int b, int c = 2);
- You can override default arguments by providing explicit values when calling the function.
Default arguments are a helpful feature in C++ as they allow you to provide sensible defaults for function parameters while still allowing flexibility for callers who may not need to specify every parameter.