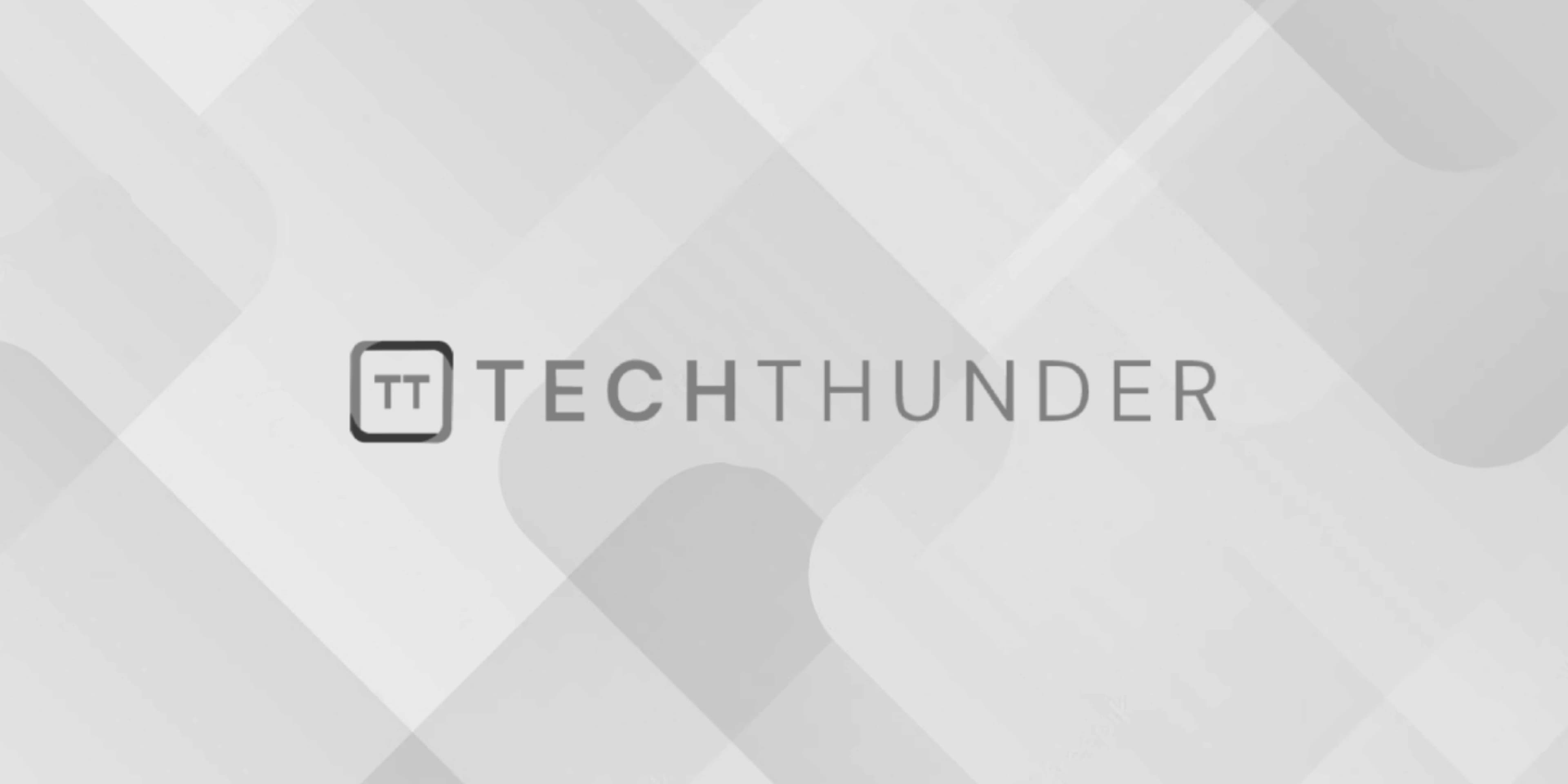
162 views
Dynamic Array in C++
The C++ dynamic array is typically implemented using the std::vector
container from the Standard Template Library (STL). A dynamic array, often referred to as a “vector,” allows you to create an array whose size can change dynamically during runtime. Here’s how you can use std::vector
in C++:
C++
#include <iostream>
#include <vector>
int main() {
// Declare an empty dynamic array (vector)
std::vector<int> dynamicArray;
// Add elements to the dynamic array
dynamicArray.push_back(1);
dynamicArray.push_back(2);
dynamicArray.push_back(3);
// Access elements in the dynamic array
std::cout << "Elements in the dynamic array:" << std::endl;
for (int i = 0; i < dynamicArray.size(); ++i) {
std::cout << dynamicArray[i] << " ";
}
std::cout << std::endl;
// Modify elements in the dynamic array
dynamicArray[1] = 4;
// Remove elements from the dynamic array
dynamicArray.pop_back();
// Size of the dynamic array
std::cout << "Size of the dynamic array: " << dynamicArray.size() << std::endl;
// Clear all elements in the dynamic array
dynamicArray.clear();
// Check if the dynamic array is empty
if (dynamicArray.empty()) {
std::cout << "The dynamic array is empty." << std::endl;
} else {
std::cout << "The dynamic array is not empty." << std::endl;
}
return 0;
}
In this example:
- We include the necessary headers (
<iostream>
and<vector>
) for usingstd::vector
. - We declare an empty dynamic array (vector) called
dynamicArray
. - We use the
push_back()
method to add elements to the end of the dynamic array. - We access elements using the index notation, just like a regular array.
- We modify elements using the same index notation.
- We use the
pop_back()
method to remove the last element from the dynamic array. - We use the
size()
method to determine the size of the dynamic array. - We use the
clear()
method to remove all elements from the dynamic array. - We use the
empty()
method to check if the dynamic array is empty.
std::vector
takes care of dynamically resizing the array as needed, so you don’t have to worry about memory management or manual resizing. It provides a convenient and efficient way to work with dynamic arrays in C++.