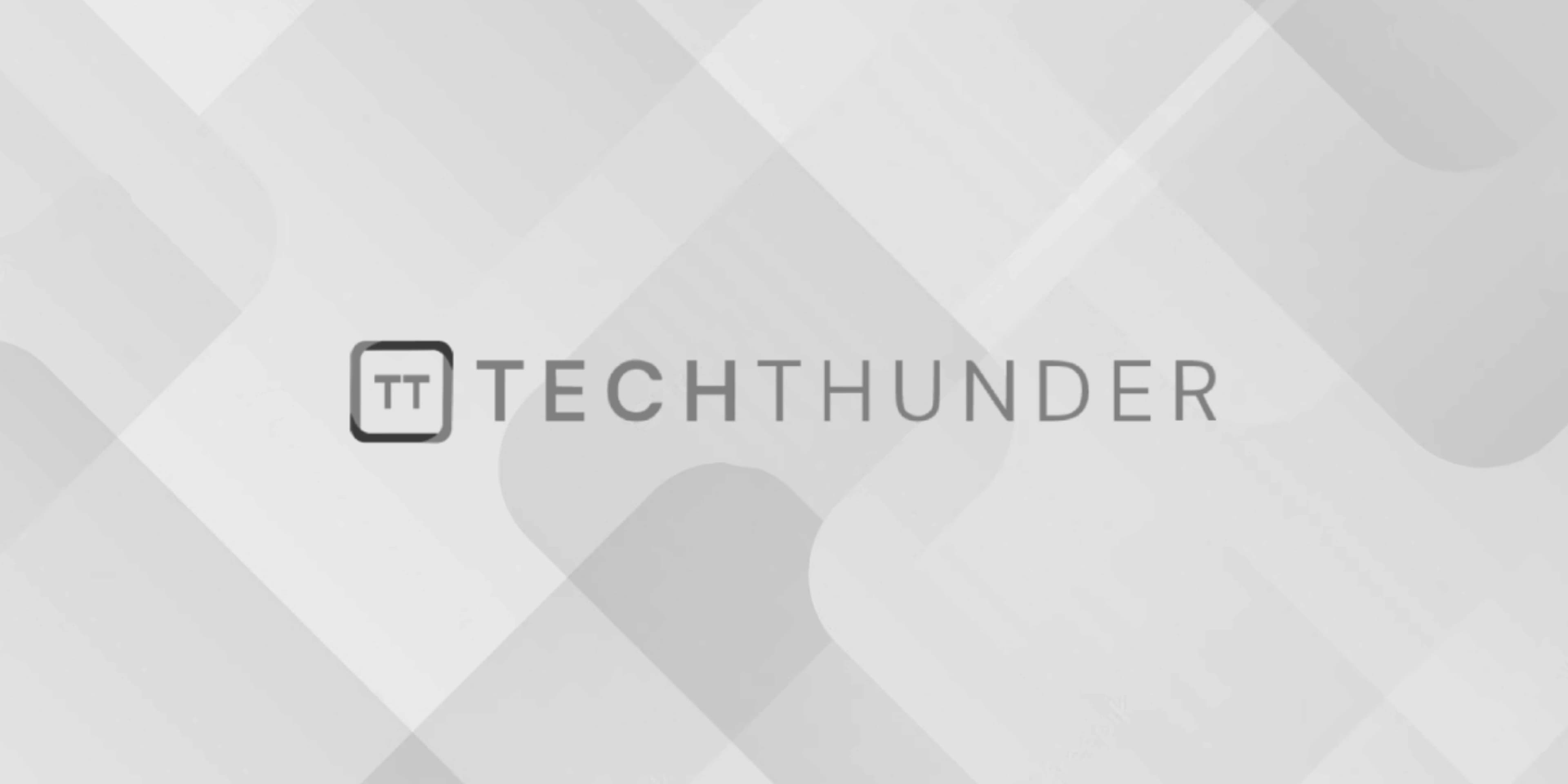
Generic Programming in C++
Generic programming in C++ is a programming paradigm that allows you to write code that can work with a variety of data types without sacrificing type safety. C++ achieves generic programming primarily through templates, which provide a mechanism for writing code that operates on types as parameters, rather than specific concrete types. This enables you to create highly reusable and efficient algorithms and data structures.
Here are some key components and concepts related to generic programming in C++:
- Templates:
- Templates are the foundation of generic programming in C++. They allow you to write code that works with types as parameters.
- There are two main types of templates: function templates and class templates.
// Function template
template <typename T>
T add(T a, T b) {
return a + b;
}
// Class template
template <typename T>
class MyContainer {
// ...
};
- Type Parameters:
- Templates use type parameters (often denoted as
typename
orclass
) to represent the types that the template will operate on. - These type parameters are specified when you use the template.
int result = add<int>(3, 5); // Explicitly specify the type parameter
- Function Templates:
- Function templates allow you to write generic functions that work with different data types.
int result1 = add(3, 5); // Type inference
double result2 = add(3.5, 2.7); // Type inference
- Class Templates:
- Class templates allow you to define generic classes that can work with different data types.
MyContainer<int> intContainer; // Using int as the type parameter
MyContainer<double> doubleContainer; // Using double as the type parameter
- Standard Template Library (STL):
- The C++ Standard Library provides a rich set of generic data structures and algorithms, including containers like vectors, maps, and algorithms like sorting and searching.
- These are implemented using class and function templates and can be customized for user-defined types.
std::vector<int> intVector;
std::map<std::string, double> priceMap;
std::sort(intVector.begin(), intVector.end());
- Concepts:
- C++20 introduced concepts, which allow you to specify constraints on template parameters. Concepts help improve template error messages and provide more control over type requirements.
template <typename T>
requires std::is_integral_v<T>
T add(T a, T b) {
return a + b;
}
Generic programming in C++ empowers you to write flexible and efficient code that can work with a wide range of data types. By using templates and the Standard Library, you can achieve a high level of code reusability and maintainability in your C++ projects.