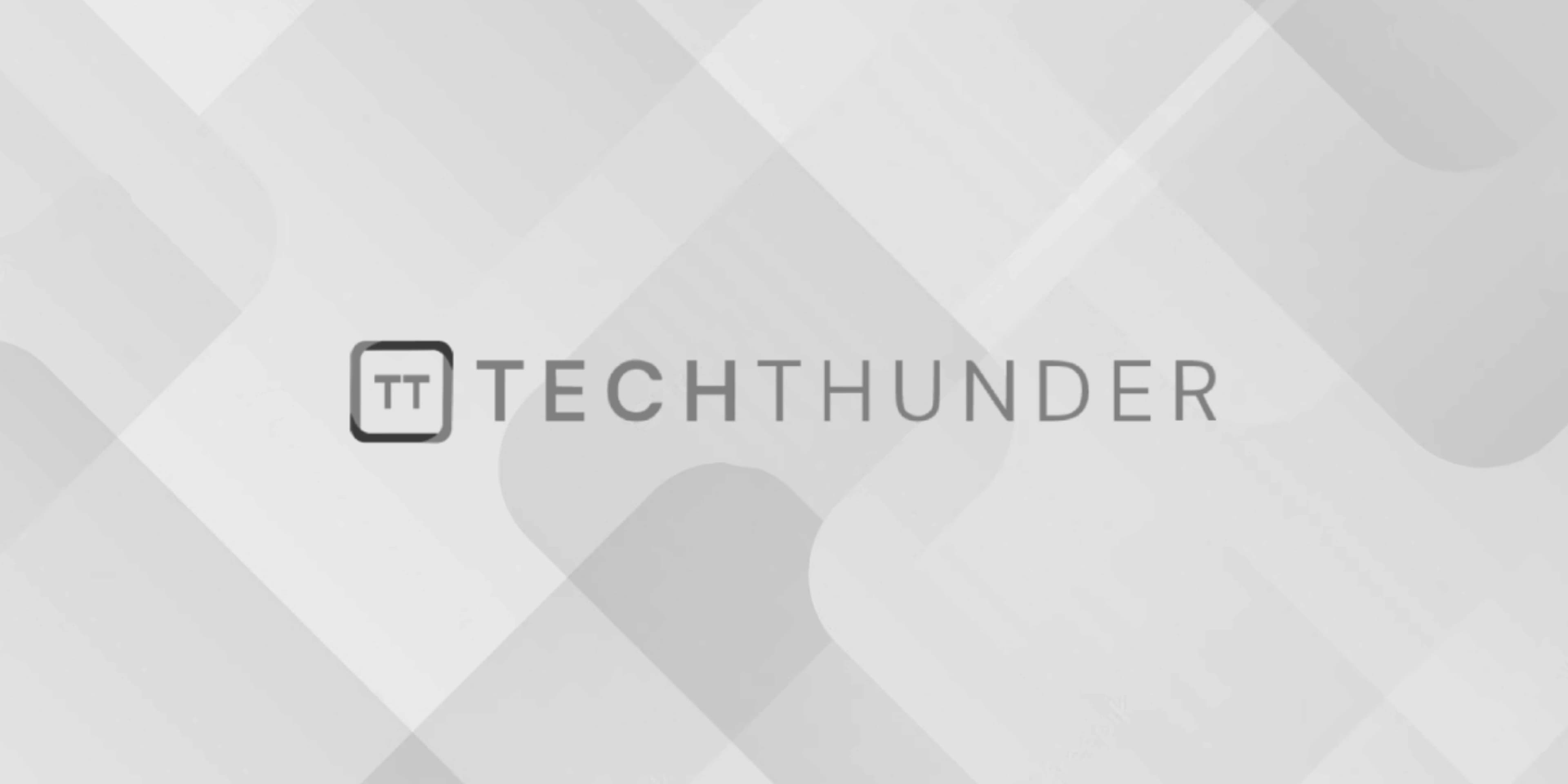
102 views
Declare a C++ Function Returning Pointer to Array of Integer Pointers
The C++ can declare a function that returns a pointer to an array of integer pointers like this:
C++
int** myFunction(int size);
In this declaration:
int**
is the return type, indicating that the function returns a pointer to an array of integer pointers.myFunction
is the name of the function.int size
is an optional parameter that specifies the size of the array or any other parameters your function may require.
Here’s a more detailed example of how you might implement such a function:
C++
#include <iostream>
int** createArrayOfPointers(int size) {
// Allocate memory for an array of integer pointers
int** arrayOfPointers = new int*[size];
// Allocate memory for each individual integer array
for (int i = 0; i < size; ++i) {
arrayOfPointers[i] = new int[5]; // Each pointer points to an array of 5 integers
}
return arrayOfPointers;
}
int main() {
int size = 3;
int** array = createArrayOfPointers(size);
// Access and modify elements in the array
for (int i = 0; i < size; ++i) {
for (int j = 0; j < 5; ++j) {
array[i][j] = i * 10 + j;
}
}
// Print the elements
for (int i = 0; i < size; ++i) {
for (int j = 0; j < 5; ++j) {
std::cout << array[i][j] << ' ';
}
std::cout << std::endl;
}
// Deallocate memory
for (int i = 0; i < size; ++i) {
delete[] array[i]; // Deallocate individual integer arrays
}
delete[] array; // Deallocate the array of pointers
return 0;
}
In this example:
createArrayOfPointers
is a function that allocates memory for an array of integer pointers and then allocates memory for each individual integer array that each pointer points to. It returns the pointer to the array of integer pointers.- In the
main
function, we callcreateArrayOfPointers
to create the array of integer pointers and then access and modify elements in the array as needed. - Finally, we deallocate the memory to avoid memory leaks.
Please note that proper memory management is crucial when working with dynamic arrays and pointers to arrays. In practice, you should consider using smart pointers or containers like std::vector
to simplify memory management and improve code safety.