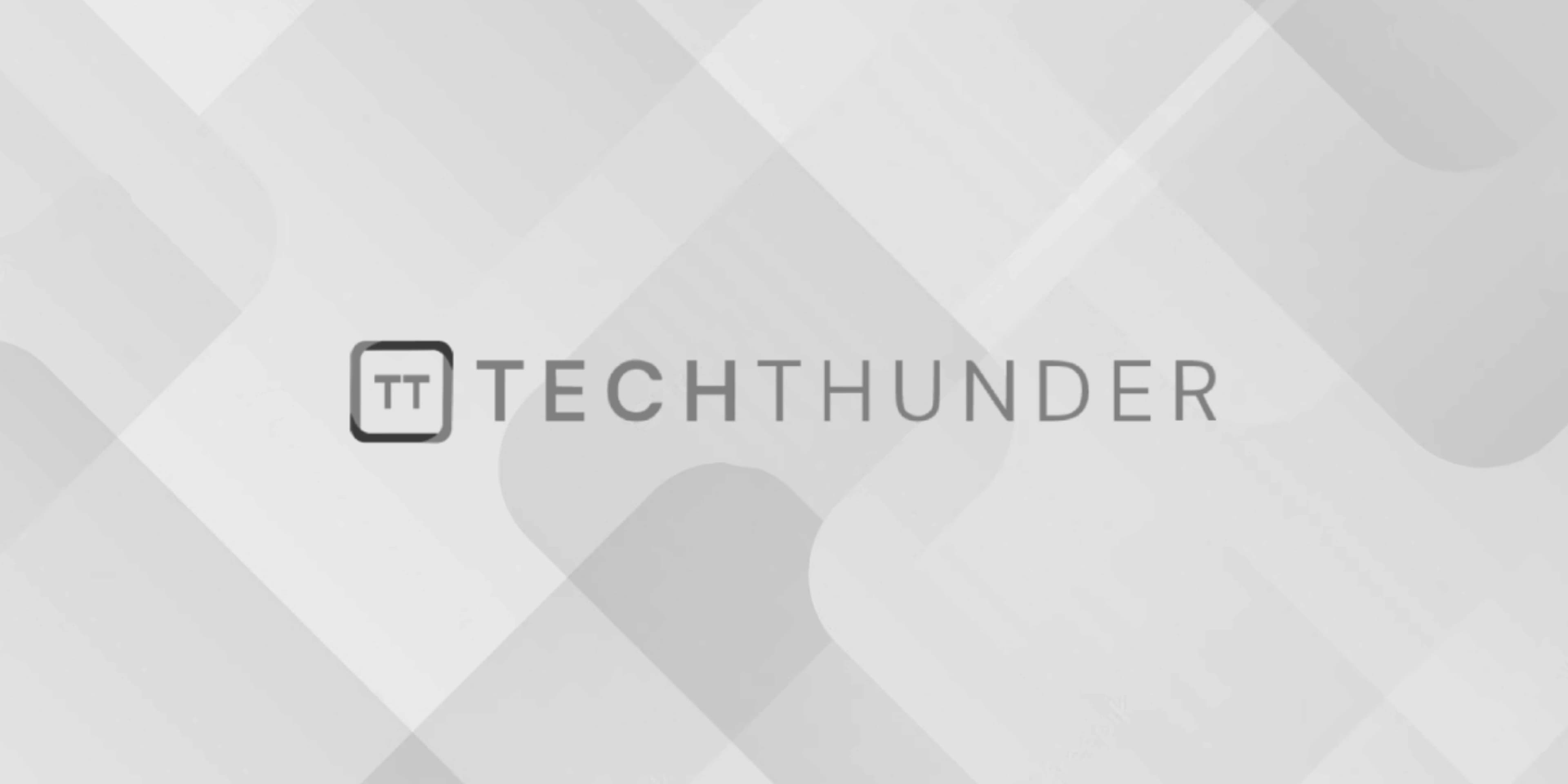
389 views
Accumulator in C++
The term “accumulator” generally refers to a variable used to accumulate or collect values over a series of iterations or operations. Accumulators are commonly used in loops, especially when you need to calculate the sum, product, or some other aggregate of multiple values.
Here’s a basic example of how you might use an accumulator to calculate the sum of a series of numbers using a for
loop:
#include <iostream>
int main() {
int total = 0; // Initialize the accumulator to zero
for (int i = 1; i <= 5; i++) {
total += i; // Accumulate the sum of numbers 1 to 5
}
std::cout << "The sum is: " << total << std::endl;
return 0;
}
In this example:
total
is the accumulator variable, initialized to zero.- Inside the
for
loop, the value ofi
(ranging from 1 to 5) is added to thetotal
at each iteration, effectively accumulating the sum. - After the loop, the value of
total
represents the accumulated sum, which is then printed to the console.
You can use accumulators in various scenarios, such as calculating averages, finding the maximum or minimum value in a list, or any other situation where you need to maintain and update a running total or aggregation. The specific use and type of accumulator variable depend on the problem you are trying to solve.